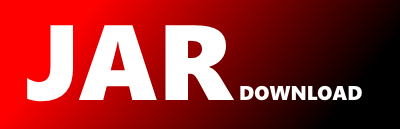
org.birchframework.bridge.jmstemplate.JMSTemplateConfigurer Maven / Gradle / Ivy
/*===============================================================
= Copyright (c) 2021 Birch Framework
= This program is free software: you can redistribute it and/or modify
= it under the terms of the GNU General Public License as published by
= the Free Software Foundation, either version 3 of the License, or
= any later version.
= This program is distributed in the hope that it will be useful,
= but WITHOUT ANY WARRANTY; without even the implied warranty of
= MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
= GNU General Public License for more details.
= You should have received a copy of the GNU General Public License
= along with this program. If not, see .
==============================================================*/
package org.birchframework.bridge.jmstemplate;
import java.time.Duration;
import javax.jms.ConnectionFactory;
import javax.jms.TopicConnectionFactory;
import org.springframework.beans.factory.ObjectProvider;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.boot.autoconfigure.jms.JmsProperties;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.boot.context.properties.PropertyMapper;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.core.JmsTemplate;
import org.springframework.jms.support.converter.MessageConverter;
import org.springframework.jms.support.destination.DestinationResolver;
/**
* Configures JMS templates given different {@link ConnectionFactory} instances. Mainly used to configure instances of {@link JmsTemplate}s
* for queues vs topics, based on their respective connection factories.
* @author Keivan Khalichi
*/
@Configuration
@ConditionalOnClass(JmsTemplate.class)
@ConditionalOnProperty(prefix = "spring.jms", name = "template")
@EnableConfigurationProperties(JmsProperties.class)
@RefreshScope
@SuppressWarnings("SpringFacetCodeInspection")
public class JMSTemplateConfigurer {
private final JmsProperties jmsProperties;
private final ObjectProvider messageConverter;
private final ObjectProvider destinationResolver;
public JMSTemplateConfigurer(final JmsProperties theJmsProperties,
final ObjectProvider theMessageConverter,
final ObjectProvider theDestinationResolver) {
this.jmsProperties = theJmsProperties;
this.messageConverter = theMessageConverter;
this.destinationResolver = theDestinationResolver;
}
@SuppressWarnings("AutoBoxing")
public void configureJMSTemplate(final JmsTemplate theJMSTemplate) {
final var aMapper = PropertyMapper.get();
final var aTemplateProperties = this.jmsProperties.getTemplate();
theJMSTemplate.setPubSubDomain(this.jmsProperties.isPubSubDomain());
aMapper.from(this.destinationResolver::getIfUnique).whenNonNull().to(theJMSTemplate::setDestinationResolver);
aMapper.from(this.messageConverter::getIfUnique).whenNonNull().to(theJMSTemplate::setMessageConverter);
aMapper.from(aTemplateProperties::getDefaultDestination).whenNonNull().to(theJMSTemplate::setDefaultDestinationName);
aMapper.from(aTemplateProperties::getDeliveryDelay).whenNonNull().as(Duration::toMillis).to(theJMSTemplate::setDeliveryDelay);
aMapper.from(aTemplateProperties::determineQosEnabled).to(theJMSTemplate::setExplicitQosEnabled);
aMapper.from(aTemplateProperties::getDeliveryMode).whenNonNull().as(JmsProperties.DeliveryMode::getValue).to(theJMSTemplate::setDeliveryMode);
aMapper.from(aTemplateProperties::getPriority).whenNonNull().to(theJMSTemplate::setPriority);
aMapper.from(aTemplateProperties::getTimeToLive).whenNonNull().as(Duration::toMillis).to(theJMSTemplate::setTimeToLive);
aMapper.from(aTemplateProperties::getReceiveTimeout).whenNonNull().as(Duration::toMillis).to(theJMSTemplate::setReceiveTimeout);
aMapper.from(() -> theJMSTemplate.getConnectionFactory() instanceof TopicConnectionFactory).to(theJMSTemplate::setPubSubDomain);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy