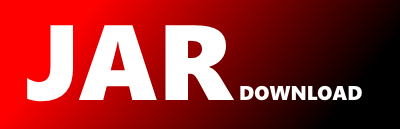
loggersoft.kotlin.utils.event.Signal.kt Maven / Gradle / Ivy
/*
* Copyright (C) 2018 Alexander Kornilov ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package loggersoft.kotlin.utils.event
/**
* A receiver of the event.
*/
typealias Receiver = (sender: S, event: E) -> Unit
/**
* Represents interface for subscription on an event.
*
* @author Alexander Kornilov ([email protected]).
*/
interface Subscription {
/**
* Subscribes [receiver] on signal.
* Optional parameter [subscriber] is used to [unsubscribe].
* @return [subscriber]
*/
fun subscribe(subscriber: Any, receiver: Receiver): Any
/**
* Subscribes [receiver] on signal without possibility to [unsubscribe].
*/
fun subscribe(receiver: Receiver) {
subscribe(receiver, receiver)
}
/**
* Unsubscribe [subscriber] from event.
*/
fun unsubscribe(subscriber: Any): Boolean
/**
* Operator equivalent of [subscribe] without possibility to [unsubscribe].
*/
operator fun plusAssign(receiver: Receiver)
= subscribe(receiver)
/**
* Operator equivalent of [unsubscribe]
*/
operator fun minusAssign(subscriber: Any) {
unsubscribe(subscriber)
}
/**
* Operator equivalent of [subscribe] with possibility to [unsubscribe].
*/
operator fun set(index: Any, receiver: Receiver) {
subscribe(index, receiver)
}
}
/**
* Represents interface of a signal for using inside sender object.
*
* @author Alexander Kornilov ([email protected]).
*/
interface Signal {
/**
* Sender object of signal.
*/
val sender: S
/**
* Reference to subscription object: the public part of [Signal] interface.
*/
val subscription: Subscription
/**
* Unmodified list of receivers.
*/
val receivers: Collection>
/**
* Checks [subscriber] for presence in subscribers list.
*/
fun hasSubscriber(subscriber: Any): Boolean
/**
* Unsubscribe all subscribers.
*/
fun unsubscribeAll()
/**
* Emits signal for all receivers with specified [event].
*/
fun emit(event: E)
/**
* Operator equivalent of [hasSubscriber].
*/
operator fun contains(subscriber: Any): Boolean
= hasSubscriber(subscriber)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy