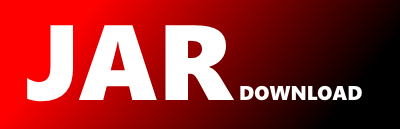
loggersoft.kotlin.utils.graph.Analyst.kt Maven / Gradle / Ivy
/*
* Copyright (C) 2018 Alexander Kornilov ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package loggersoft.kotlin.utils.graph
/**
* Represents an interface of analytic operations with a graph.
*
* @author Alexander Kornilov ([email protected]).
*/
interface Analyst {
/**
* A source graph.
*/
val graph: Graph
/**
* Returns vertexes list of the [graph] in topological order if possible; empty list otherwise.
* @note the [graph] should be oriented.
*/
fun sortTopological(): List>
/**
* Returns shortest edge between [startFrom] and [endWith] vertexes if present; `null` otherwise.
* If [onlyWeighted] is `false` for edges without weight the [defaultWeight] will be used.
* If [onlyWeighted] is `true` the edges without weight will not be taken into account.
* @throws NoSuchElementException
*/
fun getShortestEdge(startFrom: V, endWith: V, onlyWeighted: Boolean = true, defaultWeight: Double = Edge.DefaultWeight): Edge?
= if (onlyWeighted) graph.getEdges(startFrom, endWith).filter { it.hasWeight }.minBy { it.weight }
else graph.getEdges(startFrom, endWith).minBy { it.weightOrDefault(defaultWeight) }
/**
* Returns `true` when path from [startFrom] to [endsWith] can be found; `false` otherwise.
* @throws NoSuchElementException
*/
fun hasPath(startFrom: V, endWith: V): Boolean
/**
* Returns optimal path between [startFrom] and [endWith] vertexes if it exists; `null` otherwise.
* To determinate the optimal path, the weight of edges is taken into account.
* For edges without weight [Edge.DefaultWeight] is used.
* @throws NoSuchElementException
*/
fun getPath(startFrom: V, endWith: V): Path?
/**
* Returns list of all possible paths between [startFrom] and [endWith] vertexes.
* The result list will be sorted by total path weight from minimal to maximum.
* @throws NoSuchElementException
*/
fun getPaths(startFrom: V, endWith: V, limit: Int = -1): List>
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy