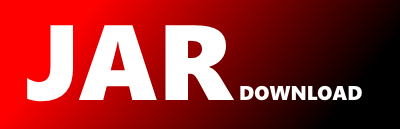
loggersoft.kotlin.utils.graph.Graph.kt Maven / Gradle / Ivy
/*
* Copyright (C) 2018 Alexander Kornilov ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package loggersoft.kotlin.utils.graph
/**
* Represents a graph.
*
* @author Alexander Kornilov ([email protected]).
*/
interface Graph : Cloneable {
/**
* A list of vertexes values.
*/
val values: Collection
/**
* A list of vertexes.
*/
val vertexes: Collection>
/**
* Indicates that the graph is empty.
*/
val isEmpty: Boolean
get() = values.isEmpty()
/**
* Indicates that the graph has only oriented edges.
*/
val isOriented: Boolean
get() = orientedEdgesCount == edgesCount
/**
* A total number of edges in the graph.
*/
val edgesCount: Int
/**
* A number of oriented edges.
*/
val orientedEdgesCount: Int
/**
* A number of edges with mutable weight.
*/
val dynamicEdgesCount: Int
/**
* Clones the graph.
*/
public override fun clone(): Graph
/**
* Clones the graph as [MutableGraph].
*/
fun cloneAsMutable(): MutableGraph
/**
* Returns `true` if graph contains [value]; `false` otherwise.
*/
operator fun contains(value: V): Boolean = value in values
/**
* Returns `true` if graph contains [edge]; `false` otherwise.
*/
operator fun contains(edge: Edge): Boolean
= try { edge in get(edge.startFrom).startsIn && edge in get(edge.endWith).endsIn } catch (_: NoSuchElementException) { false }
/**
* Returns vertex by [value].
* @throws NoSuchElementException
*/
operator fun get(value: V): Vertex
/**
* Returns list of the edges which starts from [startFrom] and ends with [endWith].
* The result list can be limited by [limit].
* @throws NoSuchElementException
*/
fun getEdges(startFrom: V, endWith: V, limit: Int = -1): Collection>
/**
* Returns `true` if [startFrom] and [endWith] vertexes are connected; `false` otherwise.
* @throws NoSuchElementException
*/
fun isConnected(startFrom: V, endWith: V): Boolean = getEdges(startFrom, endWith, 1).isNotEmpty()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy