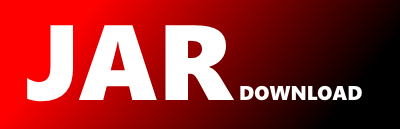
loggersoft.kotlin.utils.graph.MutableGraph.kt Maven / Gradle / Ivy
/*
* Copyright (C) 2018 Alexander Kornilov ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package loggersoft.kotlin.utils.graph
/**
* Represents a mutable graph.
*
* @author Alexander Kornilov ([email protected]).
*/
interface MutableGraph : Graph {
/**
* Adds new vertex to graph by [value].
* @returns a new vertex.
*/
fun addVertex(value: V): Vertex
/**
* Removes the vertex by [value].
*/
fun removeVertex(value: V)
/**
* Adds new vertex for [value] as operator "+=".
*/
operator fun plusAssign(value: V) { addVertex(value) }
/**
* Removes the vertex by [value] as operator "-=".
*/
operator fun minusAssign(value: V) = removeVertex(value)
/**
* Creates and add a new edge to the graph by several parameters.
* If [startFrom] or [endWith] values don't present in graph them will be added as new vertexes.
* @return the created edge.
*/
@Suppress("UNCHECKED_CAST")
fun addEdge(startFrom: V,
endWith: V,
value: E,
isOriented: Boolean = true,
isDynamicWeight: Boolean = false,
hasWeight: Boolean = true,
weight: Double = Edge.DefaultWeight): Edge
= addEdge(when {
value is AbstractEdge -> ExternalEdge(startFrom, endWith, value) as Edge
isDynamicWeight -> DynamicEdge(startFrom, endWith, value, isOriented).apply {
this.hasWeight = hasWeight
this.weight = weight
}
else -> StaticEdge(startFrom, endWith, value, isOriented, hasWeight, weight)
})
/**
* Adds a new [edge].
* @return itself.
*/
fun addEdge(edge: Edge): Edge
/**
* Removes [edge] from the graph.
* @throws NoSuchElementException
*/
fun removeEdge(edge: Edge)
/**
* Removes all edges between [startFrom] and [endWith].
* @return total number of removed edges.
* @throws NoSuchElementException
*/
fun removeEdges(startFrom: V, endWith: V): Int
= getEdges(startFrom, endWith).apply { forEach(::removeEdge) }.size
/**
* Adds new [edge] as operator "+=".
*/
operator fun plusAssign(edge: Edge) { addEdge(edge) }
/**
* Removes the [edge] as operator "-=".
*/
operator fun minusAssign(edge: Edge) = removeEdge(edge)
/**
* Clears the graph.
*/
fun clear()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy