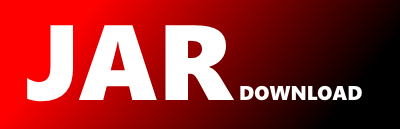
org.bitbucket.bradleysmithllc.etlunit.cli.DescribeCmd Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.cli;
import org.clamshellcli.api.Command;
import org.clamshellcli.api.Configurator;
import org.clamshellcli.api.Context;
import org.clamshellcli.api.IOConsole;
import org.bitbucket.bradleysmithllc.etlunit.feature.*;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class DescribeCmd implements Command {
private static final String NAMESPACE = "syscmd";
public static final String ACTION_NAME = "describe";
public Descriptor getDescriptor() {
return new Descriptor() {
public String getNamespace() {
return NAMESPACE;
}
public String getName() {
return ACTION_NAME;
}
public String getDescription() {
return "Displays meta information about a feature and / or operations";
}
public String getUsage() {
return "describe (operation)?";
}
public Map getArguments() {
return Collections.emptyMap();
}
};
}
public Object execute(Context context) {
String [] inputLine = (String []) context.getValue(Context.KEY_COMMAND_LINE_ARGS);
IOConsole console = context.getIoConsole();
// the first argument is the feature name
if (inputLine == null || inputLine.length < 1)
{
console.writeOutput("Missing feature name" + Configurator.VALUE_LINE_SEP);
return null;
}
ServiceLocatorFeatureLocator locator = new ServiceLocatorFeatureLocator();
List list = locator.getFeatures(FeatureLocator.feature_type.external);
if (list.size() != 0) {
String featureName = inputLine[0];
for (Feature feature : list) {
if (feature.getFeatureName().equals(featureName))
{
FeatureMetaInfo metaInfo = feature.getMetaInfo();
if (inputLine.length == 1)
{
String featureUsage = metaInfo.getFeatureUsage();
if (featureUsage != null)
{
console.writeOutput(featureUsage + Configurator.VALUE_LINE_SEP);
String config = metaInfo.getFeatureConfiguration();
if (config != null)
{
console.writeOutput(config + Configurator.VALUE_LINE_SEP);
}
else
{
console.writeOutput("Feature does not provide configuration information" + Configurator.VALUE_LINE_SEP);
}
Map ops = metaInfo.getExportedOperations();
if (ops != null)
{
console.writeOutput("exported operations:" + Configurator.VALUE_LINE_SEP);
for (Map.Entry operationEntry : ops.entrySet())
{
String operation = operationEntry.getKey();
String desc = operationEntry.getValue().getDescription();
if (desc == null)
{
desc = "NA";
}
console.writeOutput("\t" + operation + "\t\t" + desc + Configurator.VALUE_LINE_SEP);
}
}
else
{
console.writeOutput("Feature does not provide operation information" + Configurator.VALUE_LINE_SEP);
}
}
else
{
console.writeOutput("Feature " + featureName + " does not provide any description" + Configurator.VALUE_LINE_SEP);
}
}
else
{
// grab an operation
String operation = inputLine[1];
Map ops = metaInfo.getExportedOperations();
FeatureOperation featureOperation = ops.get(operation);
if (ops == null || featureOperation == null)
{
console.writeOutput("Feature " + featureName + " does not export operation " + operation + Configurator.VALUE_LINE_SEP);
return null;
}
String proto = featureOperation.getPrototype();
String usage = featureOperation.getUsage();
String desc = featureOperation.getDescription();
if (proto == null && usage == null && desc == null)
{
console.writeOutput("Operation " + operation + " does not provide any description" + Configurator.VALUE_LINE_SEP);
}
else
{
if (proto == null)
{
proto = "NA";
}
if (usage == null)
{
usage = "NA";
}
if (desc == null)
{
desc = "NA";
}
console.writeOutput("Description:" + Configurator.VALUE_LINE_SEP);
console.writeOutput("\t" + desc + Configurator.VALUE_LINE_SEP);
console.writeOutput("Prototype:" + Configurator.VALUE_LINE_SEP);
console.writeOutput("\t" + proto + Configurator.VALUE_LINE_SEP);
console.writeOutput("Usage:" + Configurator.VALUE_LINE_SEP);
console.writeOutput("\t" + usage + Configurator.VALUE_LINE_SEP);
}
}
return null;
}
}
console.writeOutput("Feature " + featureName + " could not be located" + Configurator.VALUE_LINE_SEP);
} else {
console.writeOutput("No features available" + Configurator.VALUE_LINE_SEP);
}
return null;
}
public void plug(Context context) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy