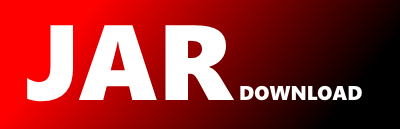
org.bitbucket.bradleysmithllc.etlunit.cli.AddAvailableFeatureCmd Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.cli;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ArrayNode;
import org.clamshellcli.api.Command;
import org.clamshellcli.api.Configurator;
import org.clamshellcli.api.Context;
import org.clamshellcli.api.IOConsole;
import com.fasterxml.jackson.databind.JsonNode;
import org.bitbucket.bradleysmithllc.etlunit.feature.Feature;
import org.bitbucket.bradleysmithllc.etlunit.maven.ETLUnitMojo;
import org.bitbucket.bradleysmithllc.etlunit.util.IOUtils;
import java.io.File;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AddAvailableFeatureCmd implements Command {
private static final String NAMESPACE = "syscmd";
public static final String ACTION_NAME = "add-feature";
public Object execute(Context ctx) {
IOConsole console = ctx.getIoConsole();
String [] inputLine = (String []) ctx.getValue(Context.KEY_COMMAND_LINE_ARGS);
File cfg = new File(".");
if (inputLine == null || inputLine.length != 1)
{
console.writeOutput("Please specify exactly one feature to add" + Configurator.VALUE_LINE_SEP);
return null;
}
try {
List installedFeatures = ListInstalledFeaturesCmd.getInstalledFeatures(cfg);
String featureName = inputLine[0];
if (installedFeatures != null && installedFeatures.contains(featureName))
{
console.writeOutput("Feature " + featureName + " already added" + Configurator.VALUE_LINE_SEP);
}
else
{
List availableFeatures = ListAvailableFeaturesCmd.getAvailableFeatures();
Feature toInstall = null;
for (Feature feature : availableFeatures)
{
if (feature.getFeatureName().equals(featureName))
{
toInstall = feature;
}
}
if (toInstall != null)
{
// load the config file and pretty print it
ObjectMapper mapper = new ObjectMapper();
File etlunitConfiguration = ETLUnitMojo.getEtlunitConfiguration(cfg);
JsonNode node = mapper.readTree("{" + IOUtils.readFileToString(etlunitConfiguration) + "}");
( (ArrayNode) node.get("install-features")).add(featureName);
String config = mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(node);
int index = config.indexOf("{");
config = config.substring(index + 1);
index = config.lastIndexOf("}");
config = config.substring(0, index - 1).trim();
IOUtils.writeBufferToFile(etlunitConfiguration, new StringBuffer(config));
console.writeOutput("Feature " + featureName + " added" + Configurator.VALUE_LINE_SEP);
}
else
{
console.writeOutput("Feature " + featureName + " not available. Run list-available-features for valid features to install" + Configurator.VALUE_LINE_SEP);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public void plug(Context plug) {
// no load-time setup needed
}
public Descriptor getDescriptor() {
return new Descriptor() {
public String getNamespace() {
return NAMESPACE;
}
public String getName() {
return ACTION_NAME;
}
public String getDescription() {
return "Adds a known feature to the project (listed in " + ListAvailableFeaturesCmd.ACTION_NAME + ")";
}
public String getUsage() {
return "";
}
public Map getArguments() {
Map map = new HashMap();
return map;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy