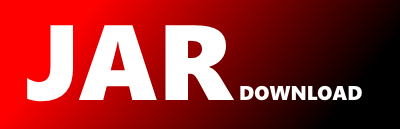
org.bitbucket.bradleysmithllc.etlunit.cli.TestCmd Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.cli;
import org.apache.maven.model.Model;
import org.apache.maven.model.io.DefaultModelReader;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import org.bitbucket.bradleysmithllc.etlunit.*;
import org.bitbucket.bradleysmithllc.etlunit.regexp.TestExpression;
import org.clamshellcli.api.Command;
import org.clamshellcli.api.Configurator;
import org.clamshellcli.api.Context;
import org.clamshellcli.api.IOConsole;
import org.bitbucket.bradleysmithllc.etlunit.feature.*;
import org.bitbucket.bradleysmithllc.etlunit.maven.ETLUnitMojo;
import org.bitbucket.bradleysmithllc.etlunit.feature.debug.ConsoleFeatureModule;
import org.bitbucket.bradleysmithllc.etlunit.feature.debug.RunAllFeatureModule;
import org.bitbucket.bradleysmithllc.etlunit.util.MapList;
import javax.inject.Inject;
import java.io.File;
import java.io.IOException;
import java.util.*;
public class TestCmd implements Command {
private static final String NAMESPACE = "syscmd";
public static final String ACTION_NAME = "test";
private MapList mapList;
public Object execute(Context ctx) {
IOConsole console = ctx.getIoConsole();
String line = String.valueOf(ctx.getValue(Context.KEY_COMMAND_LINE_INPUT));
line = line.substring(ACTION_NAME.length()).trim();
try {
ETLTestVM vm = getEtlTestVM();
SetCmd.prepareVm(vm);
// add a feature to select only the tests we were directed to
if (!line.equals(""))
{
vm.addFeature(new UserDirectedClassDirectorFeature("cli-test-selector", line));
}
vm.addFeature(new AbstractFeature()
{
@Inject
public void receiveRuntimeSupport(MapList ml)
{
mapList = ml;
}
@Override
public String getFeatureName()
{
return "mapListGrabber.0910281028100821309230";
}
});
// 'activate' the console output
ConsoleFeatureModule console1 = new ConsoleFeatureModule();
vm.addFeature(console1);
// install a dummy feature so a director can be installed which will accept all tests
vm.addFeature(new RunAllFeatureModule());
vm.installFeatures();
console.writeOutput("Running with options:" + Configurator.VALUE_LINE_SEP);
// alphabetize the list of features and options so the output is deterministic
List featureList = new ArrayList(mapList.keySet());
Collections.sort(featureList, new Comparator() {
@Override
public int compare(Feature o1, Feature o2)
{
return o1.getFeatureName().compareTo(o2.getFeatureName());
}
});
for (Feature feature : featureList)
{
console.writeOutput(feature.getFeatureName() + Configurator.VALUE_LINE_SEP);
List runtimeOptions = new ArrayList(mapList.get(feature));
Collections.sort(runtimeOptions, new Comparator() {
@Override
public int compare(RuntimeOption o1, RuntimeOption o2)
{
return o1.getName().compareTo(o2.getName());
}
});
for (RuntimeOption option : runtimeOptions)
{
console.writeOutput("\t" + option.getDescriptor().getName() + ": " + option.isEnabled() + Configurator.VALUE_LINE_SEP);
}
}
console.getOutputStream().flush();
//TEST!
TestResults results = vm.runTests();
TestResultMetrics metrics = results.getMetrics();
List resultsByClass = results.getResultsByClass();
for (TestClassResult testResult : resultsByClass)
{
for (TestMethodResult methodResult : testResult.getMethodResults())
{
if (
methodResult.getMetrics().getNumberOfAssertionFailures() != 0
||
methodResult.getMetrics().getNumberOfErrors() != 0
)
{
}
}
}
if (metrics.getNumberOfErrors() > 0 || metrics.getNumberOfAssertionFailures() > 0) {
throw new MojoExecutionException("Build failed with test errors");
}
} catch (MojoExecutionException exc) {
// ignore this one - it's just the mojo letting us know there were
// failures
} catch (Exception exc) {
exc.printStackTrace();
console.writeOutput(exc.toString() + Configurator.VALUE_LINE_SEP);
}
return null;
}
public static ETLTestVM getEtlTestVM() throws IOException
{
File basedir = new File(".");
Model model = new DefaultModelReader().read(new File(basedir, "pom.xml"), null);
MavenProject mavenProject = new MavenProject(model);
final Configuration con = ETLUnitMojo.loadConfiguration(basedir, mavenProject.getName(), mavenProject.getVersion(), CliMain.class.getClassLoader());
ServiceLocatorFeatureLocator loc = new ServiceLocatorFeatureLocator();
ETLUnitMojo.getTempDirectoryRoot(basedir);
return new ETLTestVM(loc, con);
}
public void plug(Context plug) {
// no load-time setup needed
}
public Descriptor getDescriptor() {
return new Descriptor() {
public String getNamespace() {
return NAMESPACE;
}
public String getName() {
return ACTION_NAME;
}
public String getDescription() {
return "Runs tests";
}
public String getUsage() {
return "Type 'testNames'";
}
public Map getArguments() {
Map map = new HashMap();
map.put("className", "Pattern of the test class to run");
map.put("testName", "Pattern of the test methods to run");
return map;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy