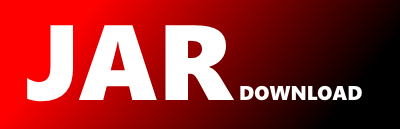
org.bitbucket.bradleysmithllc.etlunit.feature.database.DatabaseImplementation Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.feature.database;
import org.bitbucket.bradleysmithllc.etlunit.TestExecutionError;
import org.bitbucket.bradleysmithllc.etlunit.feature.database.db.Table;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestMethod;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestOperation;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestValueObject;
import java.io.File;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public interface DatabaseImplementation {
/** Connect to the database as sysadmin - the user name and password supplied in the database
* configuration.
*/
int SYSADMIN = -1;
/** Connect to the database as a test-provided user.
*/
int DATABASE = 0;
Table.type translateTableType(String JDBCMetaTableTypeName);
String getPassword(DatabaseConnection dc, String mode, int id);
String getLoginName(DatabaseConnection dc, String mode, int id);
String getPassword(DatabaseConnection dc, String mode);
String getLoginName(DatabaseConnection dc, String mode);
String getDatabaseName(DatabaseConnection dc, String mode);
void setJdbcClient(JDBCClient client);
enum operation {
/**
* (static 1) Create database logins and containers.
*/
createDatabase,
/**
* (static 2) Create database tables, etc.
*/
preCreateSchema,
/**
* (static 3) Create database tables, etc.
*/
postCreateSchema,
/**
* (static 4) Complete any tasks required for the database
*/
completeDatabaseInitialization,
/**
* (static 5) Request type to materialize views
*/
materializeViews,
/**
* (static 6) Request type to drop constraints after creating the database. At this point the database meta data will be
* available for implementations to use.
*/
dropConstraints,
/**
* (test 1) Request to clean all database tables prior to running tests.
*/
preCleanTables,
/**
* (test 2) Request to clean all database tables prior to running tests.
*/
postCleanTables
}
interface BaseRequest
{
String getMode();
DatabaseConnection getConnection();
ETLTestMethod getTestMethod();
}
interface InitializeRequest extends BaseRequest
{
File resolveSchemaReference(String ddlRef);
}
interface DatabaseRequest extends BaseRequest
{
ETLTestMethod getTestMethod();
}
interface OperationRequest
{
InitializeRequest getInitializeRequest() throws UnsupportedOperationException;
DatabaseRequest getDatabaseRequest() throws UnsupportedOperationException;
}
enum database_state
{
pass,
fail
}
database_state getDatabaseState(DatabaseConnection dc, String mode);
String getImplementationId();
Object processOperation(operation op, OperationRequest request) throws UnsupportedOperationException;
Connection getConnection(DatabaseConnection dc, String mode) throws TestExecutionError;
Connection getConnection(DatabaseConnection dc, String mode, int identifier) throws TestExecutionError;
void prepareConnectionForInsert(Connection connection, Table target, DatabaseConnection dc, String mode) throws Exception;
void returnConnection(Connection conn, DatabaseConnection dc, String mode, int id, boolean normalState) throws TestExecutionError;
String getDefaultSchema(DatabaseConnection dc, String mode);
void dispose();
boolean isTableTestVisible(DatabaseConnection dc, String mode, Table table);
void purgeTableForTest(DatabaseConnection dc, String mode, Table table) throws Exception;
/**
* Use this database's conventions to escape the identifier. E.G., in SQL Server
* this might return [name], and in another database it might return "name".
*
* @param table
* @return
* @throws Exception
*/
String escapeQualifiedIdentifier(Table table);
/**
* Use this database's conventions to escape the identifier. E.G., in SQL Server
* this might return [name], and in another database it might return "name".
*
* @param name
* @return
* @throws Exception
*/
String escapeIdentifier(String name);
/**
* Gets a jdbdc url for connecting to the specified database connection and mode.
* @param dc - connection
* @param mode - mode name or null for the default
* @param id - an implementation-specific id. The only system-defined value is 0 which means a normal connection.
* @return
*/
String getJdbcUrl(DatabaseConnection dc, String mode, int id);
/**
* Gets a jdbdc url for connecting to the specified database connection and mode. Uses implementation id 0 which
* is defined as the default.
* @param dc - connection
* @param mode - mode name or null for the default
* @return
*/
String getJdbcUrl(DatabaseConnection dc, String mode);
Class getJdbcDriverClass();
/**
* Restrict queryies pulling meta-data from the database to the user's schema only. If false, all tables
* visible in the database which are returned from the meta data query will be sent to the isTableTestVisible
* method.
* @param dc
* @return
*/
boolean restrictToOwnedSchema(DatabaseConnection dc);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy