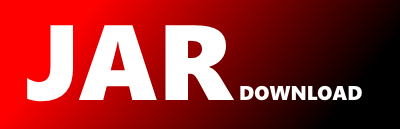
org.bitbucket.bradleysmithllc.etlunit.feature.database.DatabaseConfiguration Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.feature.database;
/*
* #%L
* etlunit-database
* %%
* Copyright (C) 2010 - 2014 bradleysmithllc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.fasterxml.jackson.databind.ObjectMapper;
import org.bitbucket.bradleysmithllc.etlunit.feature.database.json.*;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestValueObject;
import java.io.IOException;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class DatabaseConfiguration {
private final DatabaseConnection defaultConnection;
private final String defaultImplementationId;
private final ImplementationDefaults implementationDefaults;
private final String dataFileColumnDelimiter;
private final String dataFileRowDelimiter;
private final String dataFileNullToken;
private final Map connectionMap = new HashMap();
public DatabaseConfiguration(ETLTestValueObject featureConfiguration, String projectName, String projectVersion, String userName, String projectUid) {
ObjectMapper objectMapper = new ObjectMapper();
DatabaseFeatureModuleConfiguration dfmc;
try {
dfmc = objectMapper.readValue(featureConfiguration.getJsonNode().toString(), DatabaseFeatureModuleConfiguration.class);
} catch (IOException e) {
throw new RuntimeException("Error reading configuration into Json class", e);
}
defaultImplementationId = dfmc.getDefaultImplementationId();
String dfcd = dfmc.getDataFileColumnDelimiter();
if (dfcd != null) {
dataFileColumnDelimiter = dfcd;
} else {
dataFileColumnDelimiter = "\t";
}
String dfrd = dfmc.getDataFileRowDelimiter();
if (dfrd != null) {
dataFileRowDelimiter = dfrd;
} else {
dataFileRowDelimiter = "\n";
}
String dfnd = dfmc.getDataFileNullDelimiter();
if (dfnd != null) {
dataFileNullToken = dfnd;
} else {
dataFileNullToken = "null";
}
// load the implementation defaults before the database definitions so that they may be referred back to
implementationDefaults = dfmc.getImplementationDefaults();
DatabaseDefinitions dbdefs = dfmc.getDatabaseDefinitions();
if (dbdefs == null) {
throw new IllegalArgumentException("Database configuration missing database definitions");
}
Map vmap = dbdefs.getAdditionalProperties();
Set> eset = vmap.entrySet();
for (Map.Entry entry : eset) {
String id = entry.getKey();
DatabaseDefinitionsProperty value = entry.getValue();
DatabaseConnection dc = new DatabaseConnectionImpl(id, value, this, projectName, projectVersion, userName, projectUid);
connectionMap.put(id, dc);
}
String defConn = dfmc.getDefaultConnectionId();
if (defConn != null) {
defaultConnection = connectionMap.get(defConn);
if (defaultConnection == null)
{
throw new IllegalArgumentException("Default connection id " + defConn + " not found");
}
} else {
defaultConnection = null;
}
}
public boolean hasDefaultConnection()
{
return getDefaultConnection() != null;
}
public DatabaseConnection getDefaultConnection() {
return defaultConnection;
}
public String getDefaultImplementationId() {
return defaultImplementationId;
}
public DatabaseConnection getDatabaseConnection(String id)
{
return connectionMap.get(id);
}
public String getDataFileColumnDelimiter()
{
return dataFileColumnDelimiter;
}
public String getDataFileRowDelimiter()
{
return dataFileRowDelimiter;
}
public String getDataFileNullToken()
{
return dataFileNullToken;
}
public String getEffectiveServerName(DatabaseDefinitionsProperty conn) {
String implId = getImplementationId(conn);
String serverName = conn.getServerName();
if (serverName == null)
{
// check for an implementation default
ImplementationDefaultsProperty implementationDefaultsProperty = getImplDefaults(implId);
if (implementationDefaultsProperty != null)
{
serverName = implementationDefaultsProperty.getServerName();
}
}
// special host name which means 'A default is set for this implementation id and I don't want to specify a name', use the local host
if (serverName != null && serverName.equals("[local]"))
{
serverName = null;
}
// host name is still null - default to the local host
if (serverName == null)
{
try {
serverName = InetAddress.getLocalHost().getHostName();
} catch (UnknownHostException e) {
throw new IllegalArgumentException(e);
}
}
return serverName;
}
private ImplementationDefaultsProperty getImplDefaults(String implId) {
if (implementationDefaults != null)
{
Map implDe = implementationDefaults.getAdditionalProperties();
if (implDe != null)
{
return implDe.get(implId);
}
}
return null;
}
public int getEffectiveServerPort(DatabaseDefinitionsProperty conn) {
String implId = getImplementationId(conn);
Long serverPort = conn.getServerPort();
if (serverPort == null)
{
// check for an implementation default
ImplementationDefaultsProperty implementationDefaultsProperty = getImplDefaults(implId);
if (implementationDefaultsProperty != null)
{
serverPort = implementationDefaultsProperty.getServerPort();
}
}
return serverPort != null ? serverPort.intValue() : -1;
}
public String getEffectiveUserName(DatabaseDefinitionsProperty conn) {
String implId = getImplementationId(conn);
String userName = conn.getUserName();
if (userName == null)
{
// check for an implementation default
ImplementationDefaultsProperty implementationDefaultsProperty = getImplDefaults(implId);
if (implementationDefaultsProperty != null)
{
userName = implementationDefaultsProperty.getUserName();
}
}
return userName;
}
public String getEffectivePassword(DatabaseDefinitionsProperty conn) {
String implId = getImplementationId(conn);
String password = conn.getPassword();
if (password == null)
{
// check for an implementation default
ImplementationDefaultsProperty implementationDefaultsProperty = getImplDefaults(implId);
if (implementationDefaultsProperty != null)
{
password = implementationDefaultsProperty.getPassword();
}
}
return password;
}
public Map getEffectiveImplementationProperties(DatabaseDefinitionsProperty conn) {
String implId = getImplementationId(conn);
Map properties = null;
ImplementationProperties__1 implementationProperties = conn.getImplementationProperties();
if (implementationProperties != null)
{
properties = implementationProperties.getAdditionalProperties();
}
if (properties == null && implementationDefaults != null)
{
// check for an implementation default
ImplementationDefaultsProperty implementationDefaultsProperty = getImplDefaults(implId);
if (implementationDefaultsProperty != null && implementationDefaultsProperty.getImplementationProperties() != null)
{
properties = implementationDefaultsProperty.getImplementationProperties().getAdditionalProperties();
}
}
return properties;
}
private String getImplementationId(DatabaseDefinitionsProperty conn) {
return conn.getImplementationId() == null ? getDefaultImplementationId() : conn.getImplementationId();
}
public Map getConnectionMap()
{
return connectionMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy