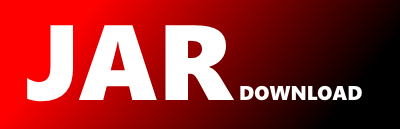
org.bitbucket.bradleysmithllc.etlunit.feature.file.FileAssertExtender Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.feature.file;
import com.google.inject.name.Named;
import org.bitbucket.bradleysmithllc.etlunit.*;
import org.bitbucket.bradleysmithllc.etlunit.context.VariableContext;
import org.bitbucket.bradleysmithllc.etlunit.feature.Feature;
import org.bitbucket.bradleysmithllc.etlunit.feature.RuntimeOption;
import org.bitbucket.bradleysmithllc.etlunit.feature.extend.Extender;
import org.bitbucket.bradleysmithllc.etlunit.feature.file.json.file._assert.AssertHandler;
import org.bitbucket.bradleysmithllc.etlunit.feature.file.json.file._assert.AssertRequest;
import org.bitbucket.bradleysmithllc.etlunit.io.file.*;
import org.bitbucket.bradleysmithllc.etlunit.metadata.MetaDataArtifact;
import org.bitbucket.bradleysmithllc.etlunit.metadata.MetaDataArtifactContent;
import org.bitbucket.bradleysmithllc.etlunit.metadata.MetaDataContext;
import org.bitbucket.bradleysmithllc.etlunit.metadata.MetaDataPackageContext;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestMethod;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestOperation;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestValueObject;
import javax.inject.Inject;
import java.io.File;
import java.util.*;
public class FileAssertExtender implements Extender, AssertHandler
{
private final FileFeatureModule fileFeatureModule;
private FileRuntimeSupport fileRuntimeSupport;
private RuntimeSupport runtimeSupport;
private DiffManager diffManager;
private Log applicationLog;
private DataFileManager dataFileManager;
@Inject
@Named("file.refreshAssertionData")
private RuntimeOption refreshAssertionData = null;
public FileAssertExtender(FileFeatureModule fileFeatureModule)
{
this.fileFeatureModule = fileFeatureModule;
}
@Inject
public void receiveDataFileManager(DataFileManager dfm)
{
dataFileManager = dfm;
}
@Inject
public void receiveRuntimeSupport(RuntimeSupport rs)
{
runtimeSupport = rs;
}
@Inject
public void receiveApplicationLog(@Named("applicationLog") Log log)
{
this.applicationLog = log;
}
@Inject
public void receiveFileRuntimeSupport(FileRuntimeSupport support)
{
fileRuntimeSupport = support;
}
@Inject
public void receiveDiffManager(DiffManager reporter)
{
diffManager = reporter;
}
public Feature getFeature()
{
return fileFeatureModule;
}
public action_code _assert(final AssertRequest operation, final ETLTestMethod mt, final ETLTestOperation op, final ETLTestValueObject obj, final VariableContext context, ExecutionContext econtext) throws TestAssertionFailure, TestExecutionError, TestWarning
{
MetaDataContext fcontext = fileFeatureModule.getDataMetaContext();
final MetaDataPackageContext pcontext = fcontext.createPackageContextForCurrentTest(MetaDataPackageContext.path_type.test_source);
String fileName = operation.getFile();
final File assertionSource = fileRuntimeSupport.getAssertionFileForCurrentTest(fileName);
MetaDataArtifact pcontextArtifact;
MetaDataArtifactContent artContent;
String targetFileName = operation.getTargetFileName();
if (targetFileName == null)
{
targetFileName = fileName;
}
FileProducer fp = fileRuntimeSupport.getRegisteredProducer(operation.getProducer());
final File target = fp.locateFileWithClassifierAndContext(targetFileName, operation.getClassifier(), operation.getContext());
if (fileRuntimeSupport.processDataSetAssertion(new FileRuntimeSupport.DataSetAssertionRequest() {
@Override
public FileRuntimeSupportImpl.DataSetAssertionRequest.assertMode getAssertMode() {
AssertRequest.AssertionMode assertionMode = operation.getAssertionMode();
return assertionMode != null ? FileRuntimeSupport.DataSetAssertionRequest.assertMode.valueOf(assertionMode.name().toLowerCase()) : assertMode.equals;
}
@Override
public String getActualSchemaName() {
String tfn = operation.getTargetFileName();
return tfn != null ? tfn : getExpectedSchemaName();
}
@Override
public String getActualBackupSchemaName() {
return getActualSchemaName();
}
@Override
public String getExpectedSchemaName() {
return operation.getFile();
}
@Override
public String getExpectedBackupSchemaName() {
return getExpectedSchemaName();
}
@Override
public boolean hasColumnList() {
return operation.getColumnListMode() != null;
}
@Override
public FileRuntimeSupportImpl.DataSetAssertionRequest.columnListMode getColumnListMode() {
AssertRequest.ColumnListMode columnListMode2 = operation.getColumnListMode();
return columnListMode2 != null ? FileRuntimeSupport.DataSetAssertionRequest.columnListMode.valueOf(columnListMode2.name().toLowerCase()) : columnListMode.exclude;
}
@Override
public Set getColumnList() {
return operation.getColumnList();
}
@Override
public String getFailureId() {
return operation.getFailureId();
}
@Override
public File getExpectedDataset() {
return assertionSource;
}
@Override
public File getActualDataset() {
return target;
}
@Override
public ETLTestMethod getTestMethod() {
return mt;
}
@Override
public ETLTestOperation getTestOperation() {
return op;
}
@Override
public ETLTestValueObject getOperationParameters() {
return obj;
}
@Override
public VariableContext getVariableContext() {
return context;
}
@Override
public MetaDataPackageContext getMetaDataPackageContext() {
return pcontext;
}
@Override
public boolean refreshAssertionData() {
return refreshAssertionData.isEnabled();
}
@Override
public DataFileSchema getSourceSchema() {
return null;
}
}))
return action_code.handled;
return ClassResponder.action_code.handled;
}
public action_code process(ETLTestMethod mt, ETLTestOperation op, ETLTestValueObject parameters, VariableContext vcontext, ExecutionContext econtext) throws TestAssertionFailure, TestExecutionError, TestWarning
{
return action_code.defer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy