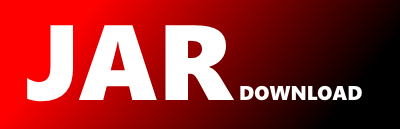
org.bitbucket.bradleysmithllc.etlunit.feature.file.ContextFile Maven / Gradle / Ivy
The newest version!
package org.bitbucket.bradleysmithllc.etlunit.feature.file;
/*
* #%L
* etlunit-file
* %%
* Copyright (C) 2010 - 2023 bradleysmithllc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.fasterxml.jackson.databind.JsonNode;
import com.github.fge.jackson.JsonLoader;
import org.bitbucket.bradleysmithllc.etlunit.util.JSonBuilderProxy;
import java.io.File;
import java.io.IOException;
public class ContextFile
{
private final File file;
private final String fileName;
private final String destinationName;
private final String variableName;
private final String contextName;
private final String classifierName;
public ContextFile(File path, String fileName, String destinationName, String variableName, String classifier, String contextName)
{
this.file = path;
this.fileName = fileName;
this.destinationName = destinationName;
this.variableName = variableName;
this.contextName = contextName;
this.classifierName = classifier;
}
public File getFile()
{
return file;
}
public String getFileName()
{
return fileName;
}
public String getDestinationName()
{
return destinationName;
}
public String getVariableName()
{
return variableName;
}
public String getContextName()
{
return contextName;
}
public String getClassifierName()
{
return classifierName;
}
public JsonNode toJsonNode()
{
JSonBuilderProxy proxy = new JSonBuilderProxy().object().key("file").value(file.getAbsolutePath());
proxy = proxy.key("file-name").value(fileName);
if (classifierName != null)
{
proxy = proxy.key("classifier-name").value(classifierName);
}
if (destinationName != null)
{
proxy = proxy.key("destination-name").value(destinationName);
}
if (variableName != null)
{
proxy = proxy.key("variable-name").value(variableName);
}
if (contextName != null)
{
proxy = proxy.key("context-name").value(contextName);
}
proxy.endObject();
try
{
return JsonLoader.fromString(proxy.toString());
}
catch (IOException e)
{
throw new RuntimeException("Bad Builder!", e);
}
}
public static ContextFile parse(JsonNode jsonNode)
{
ContextFileBuilder contextFileBuilder = new ContextFileBuilder(new File(jsonNode.get("file").asText()), jsonNode.get("file-name").asText());
JsonNode q = jsonNode.get("destination-name");
if (q != null)
{
contextFileBuilder.withDestinationName(q.asText());
}
q = jsonNode.get("classifier-name");
if (q != null)
{
contextFileBuilder.withClassifier(q.asText());
}
q = jsonNode.get("variable-name");
if (q != null)
{
contextFileBuilder.withVariableName(q.asText());
}
q = jsonNode.get("context-name");
if (q != null)
{
contextFileBuilder.withContextName(q.asText());
}
return contextFileBuilder.create();
}
@Override
public boolean equals(Object o)
{
if (this == o)
{
return true;
}
if (o == null || getClass() != o.getClass())
{
return false;
}
ContextFile that = (ContextFile) o;
if (classifierName != null ? !classifierName.equals(that.classifierName) : that.classifierName != null)
{
return false;
}
if (contextName != null ? !contextName.equals(that.contextName) : that.contextName != null)
{
return false;
}
if (!destinationName.equals(that.destinationName))
{
return false;
}
if (!file.equals(that.file))
{
return false;
}
if (!fileName.equals(that.fileName))
{
return false;
}
if (variableName != null ? !variableName.equals(that.variableName) : that.variableName != null)
{
return false;
}
return true;
}
@Override
public int hashCode()
{
int result = file.hashCode();
result = 31 * result + fileName.hashCode();
result = 31 * result + destinationName.hashCode();
result = 31 * result + (variableName != null ? variableName.hashCode() : 0);
result = 31 * result + (contextName != null ? contextName.hashCode() : 0);
result = 31 * result + (classifierName != null ? classifierName.hashCode() : 0);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy