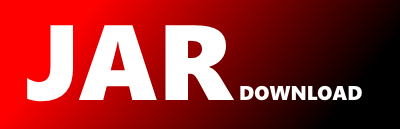
org.bitbucket.bradleysmithllc.etlunit.feature.file.FileFeatureModule Maven / Gradle / Ivy
The newest version!
package org.bitbucket.bradleysmithllc.etlunit.feature.file;
/*
* #%L
* etlunit-file
* %%
* Copyright (C) 2010 - 2023 bradleysmithllc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.google.inject.Binder;
import com.google.inject.Injector;
import com.google.inject.Module;
import org.bitbucket.bradleysmithllc.etlunit.context.VariableContext;
import org.bitbucket.bradleysmithllc.etlunit.feature.AbstractFeature;
import org.bitbucket.bradleysmithllc.etlunit.feature.FeatureModule;
import org.bitbucket.bradleysmithllc.etlunit.listener.ClassListener;
import org.bitbucket.bradleysmithllc.etlunit.metadata.*;
import org.bitbucket.bradleysmithllc.etlunit.parser.*;
import javax.inject.Inject;
import java.util.Arrays;
import java.util.List;
/**
* Staged file objects look like this:
* fileContext_[contextName] [
* {
* file: '/path/to/file'
* (, classifier: 'variableName')?
* (, destination-name: 'destinationName')?
* }(,{ . . . })*
* ]
*
* If this is the default context, then the variable name will be fileContext_DEFAULT.
* Where file is the path to the file to be utilized, and classifier is a meaning that is specific to the file consumer.
*/
@FeatureModule
public class FileFeatureModule extends AbstractFeature
{
private final FileAssertHandler fileAssertHandler = new FileAssertHandler(this);
private final FileStageHandler stageHandler = new FileStageHandler();
private final FileRegisterHandler registerHandler = new FileRegisterHandler();
private MetaDataManager metaDataManager;
private MetaDataContext fileMetaContext;
private static final List prerequisites = Arrays.asList("logging", "assert", "stage");
private static final String CONTEXT_PREFIX = "fileContext[";
private static final String CONTEXT_POSTFIX = "]";
private static final String DEFAULT_CONTEXT_NAME = FileContext.DEFAULT_CONTEXT_NAME;
private final FileRuntimeSupportImpl fileRuntimeSupport = new FileRuntimeSupportImpl();
private MetaDataContext dataMetaContext;
@Inject
public void receiveMetaDataManager(MetaDataManager manager)
{
metaDataManager = manager;
fileMetaContext = metaDataManager.getMetaData().getOrCreateContext("file");
dataMetaContext = metaDataManager.getMetaData().getOrCreateContext("data");
}
@Override
public void initialize(Injector inj)
{
postCreate(fileAssertHandler);
postCreate(stageHandler);
postCreate(registerHandler);
}
@Override
protected Injector preCreateSub(Injector inj)
{
Injector inj2 = inj.createChildInjector(new Module()
{
public void configure(Binder binder)
{
binder.bind(FileRuntimeSupport.class).toInstance(fileRuntimeSupport);
}
});
fileRuntimeSupport.finishCreate();
return inj2;
}
@Override
public List getPrerequisites()
{
return prerequisites;
}
@Override
protected List getSupportedFolderNamesSub()
{
return Arrays.asList("files");
}
public String getFeatureName()
{
return "file";
}
@Override
public List getListenerList()
{
return Arrays.asList(stageHandler, (ClassListener) fileAssertHandler, registerHandler);
}
static String getFileContextName(ETLTestValueObject param)
{
ETLTestValueObject contextNameObj = param.query("context-name");
String contextName = null;
if (contextNameObj != null)
{
contextName = contextNameObj.getValueAsString();
}
return contextName;
}
static String getFileContextId(ETLTestValueObject param)
{
return getContextKey(getFileContextName(param));
}
static void setFileContext(ETLTestValueObject param, VariableContext vcontext, String json)
{
String fileContextId = getFileContextId(param);
if (!vcontext.hasVariableBeenDeclared(fileContextId))
{
try
{
vcontext.declareAndSetValue(fileContextId, ETLTestParser.loadObject("{}"));
}
catch (ParseException e)
{
throw new RuntimeException(e);
}
}
ETLTestValueObject fcontext = getFileContextContainer(param, vcontext);
new ETLTestValueObjectBuilder(fcontext).key("file-list").jsonValue(json);
}
static ETLTestValueObject getFileContext(ETLTestValueObject param, VariableContext vcontext)
{
ETLTestValueObject context = getFileContextContainer(param, vcontext);
if (context != null)
{
return context.query("file-list");
}
return null;
}
static ETLTestValueObject getFileContextContainer(ETLTestValueObject param, VariableContext vcontext)
{
String contextKey = getFileContextId(param);
if (vcontext.hasVariableBeenDeclared(contextKey))
{
return vcontext.getValue(contextKey);
}
return null;
}
public MetaDataContext getFileMetaContext()
{
return fileMetaContext;
}
public MetaDataContext getDataMetaContext()
{
return dataMetaContext;
}
/**
* @param id - null for default context
* @return
*/
private static String getContextKey(String id)
{
return CONTEXT_PREFIX + (id == null ? DEFAULT_CONTEXT_NAME : id) + CONTEXT_POSTFIX;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy