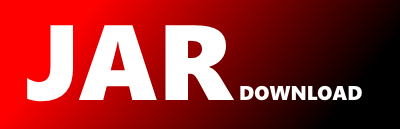
org.bitbucket.bradleysmithllc.etlunit.maven.SurefireStatusReporter Maven / Gradle / Ivy
package org.bitbucket.bradleysmithllc.etlunit.maven;
/*
* #%L
* etlunit-maven
* %%
* Copyright (C) 2010 - 2014 bradleysmithllc
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import org.bitbucket.bradleysmithllc.etlunit.NullStatusReporterImpl;
import org.bitbucket.bradleysmithllc.etlunit.StatusReporter;
import org.bitbucket.bradleysmithllc.etlunit.TestExecutionError;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestClass;
import org.bitbucket.bradleysmithllc.etlunit.parser.ETLTestMethod;
import org.bitbucket.bradleysmithllc.etlunit.util.HashMapArrayList;
import org.bitbucket.bradleysmithllc.etlunit.util.IOUtils;
import org.bitbucket.bradleysmithllc.etlunit.util.MapList;
import org.bitbucket.bradleysmithllc.etlunit.util.StringUtils;
import java.io.File;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.List;
import java.util.Map;
public class SurefireStatusReporter extends NullStatusReporterImpl {
private MapList currentTests = new HashMapArrayList();
private int numFailures;
private int numErrors;
private final File targetDirectory;
SurefireStatusReporter(File target)
{
targetDirectory = target;
}
private void publishReport() {
for (Map.Entry> classEntry : currentTests.entrySet())
{
String qname = classEntry.getKey().getQualifiedName();
StringBuffer buffer = new StringBuffer("\n");
buffer.append("\n");
buffer.append(" \n");
for (TestResult result : classEntry.getValue())
{
buffer.append("\n");
if (result.testResult == TestResult.result.error)
{
buffer.append("\t \n");
}
else if (result.testResult == TestResult.result.fail)
{
buffer.append("\t \n");
}
buffer.append(" \n");
}
buffer.append(" ");
try {
IOUtils.writeBufferToFile(new File(targetDirectory, "TEST-" + qname + ".xml"), buffer);
} catch (IOException e) {
throw new RuntimeException("");
}
}
}
@Override
public synchronized void testCompleted(ETLTestMethod method, StatusReporter.CompletionStatus status) {
TestResult result = new TestResult();
result.testName = method.getName();
result.testResult = TestResult.result.pass;
switch(status.getTestResult())
{
case success:
break;
case error:
result.testResult = TestResult.result.error;
StringWriter stringWriter = new StringWriter();
PrintWriter writer = new PrintWriter(stringWriter);
for (TestExecutionError err : status.getErrors())
{
err.printStackTrace(writer);
}
result.failureMessage = status.getErrors().toString() + "\n" + stringWriter.toString();
numErrors++;
break;
case failure:
result.testResult = TestResult.result.fail;
result.failureMessage = status.getAssertionFailures().toString();
numFailures++;
break;
}
currentTests.getOrCreate(method.getTestClass()).add(result);
}
@Override
public void testsCompleted() {
publishReport();
}
}
final class TestResult {
enum result {
error,
fail,
pass
}
result testResult;
String testName;
long elapsedTime;
String failureMessage;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy