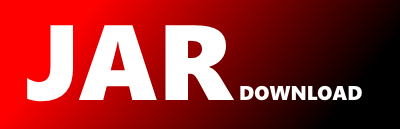
org.bitbucket.cowwoc.requirements.java.CollectionVerifier Maven / Gradle / Ivy
/*
* Copyright (c) 2015 Gili Tzabari
* Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package org.bitbucket.cowwoc.requirements.java;
import org.bitbucket.cowwoc.requirements.java.capabilities.ObjectCapabilities;
import java.util.Collection;
import java.util.function.Consumer;
/**
* Verifies the requirements of a {@link Collection}.
*
* @param the type of the collection
* @param the type of elements in the collection
*/
public interface CollectionVerifier, E>
extends ObjectCapabilities, C>
{
/**
* Ensures that the actual value is empty.
*
* @return this
* @throws IllegalArgumentException if the actual value is not empty
*/
CollectionVerifier isEmpty();
/**
* Ensures that the actual value is not empty.
*
* @return this
* @throws IllegalArgumentException if the actual value is empty
*/
CollectionVerifier isNotEmpty();
/**
* Ensures that the actual value contains an element.
*
* @param element the element that must exist
* @return this
* @throws IllegalArgumentException if the collection does not contain {@code element}
*/
CollectionVerifier contains(E element);
/**
* Ensures that the actual value contains an element.
*
* @param expected the element that must exist
* @param name the name of the expected element
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if the collection does not contain {@code expected}. If {@code name}
* is empty.
*/
CollectionVerifier contains(E expected, String name);
/**
* Ensures that the actual value contains exactly the specified elements; nothing less, nothing more.
* This method is equivalent to an {@link #isEqualTo(Object) equality comparison} that ignores element
* ordering.
*
* @param expected the elements that must exist
* @return this
* @throws NullPointerException if {@code expected} is null
* @throws IllegalArgumentException if the collection is missing any elements in {@code expected};
* if the collection contains elements not found in
* {@code expected}
*/
CollectionVerifier containsExactly(Collection expected);
/**
* Ensures that the actual value contains exactly the specified elements; nothing less, nothing more.
* This method is equivalent to an {@link #isEqualTo(Object) equality comparison} that ignores element
* ordering.
*
* @param expected the elements that must exist
* @param name the name of the expected elements
* @return this
* @throws NullPointerException if {@code expected} or {@code name} are null
* @throws IllegalArgumentException if the collection is missing any elements in {@code expected}. If
* the collection contains elements not found in {@code expected}. If
* {@code name} is empty.
*/
CollectionVerifier containsExactly(Collection expected, String name);
/**
* Ensures that the actual value contains any of specified elements.
*
* @param expected the elements that must exist
* @return this
* @throws NullPointerException if {@code expected} is null
* @throws IllegalArgumentException if the collection does not contain any of {@code expected}
*/
CollectionVerifier containsAny(Collection expected);
/**
* Ensures that the actual value contains any of the specified elements.
*
* @param expected the elements that must exist
* @param name the name of the expected elements
* @return this
* @throws NullPointerException if {@code expected} or {@code name} are null
* @throws IllegalArgumentException if the collection does not contain any of {@code expected}. If
* {@code name} is empty.
*/
CollectionVerifier containsAny(Collection expected, String name);
/**
* Ensures that the actual value contains all of the specified elements.
*
* @param expected the elements that must exist
* @return this
* @throws NullPointerException if {@code expected} is null
* @throws IllegalArgumentException if the collection does not contain all of {@code expected}
*/
CollectionVerifier containsAll(Collection expected);
/**
* Ensures that the actual value contains all of the specified elements.
*
* @param expected the elements that must exist
* @param name the name of the expected elements
* @return this
* @throws NullPointerException if {@code expected} or {@code name} are null
* @throws IllegalArgumentException if the collection does not contain all of {@code expected}. If
* {@code name} is empty.
*/
CollectionVerifier containsAll(Collection expected, String name);
/**
* Ensures that the actual value does not contain an element.
*
* @param element the element that must not exist
* @return this
* @throws IllegalArgumentException if the collection contains {@code element}
*/
CollectionVerifier doesNotContain(E element);
/**
* Ensures that the actual value does not contain an element.
*
* @param element the element that must not exist
* @param name the name of the element
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if the collection contains {@code element}. If {@code name} is empty.
*/
CollectionVerifier doesNotContain(E element, String name);
/**
* Ensures that the actual value does not contain exactly the specified elements; nothing less, nothing more.
*
* @param other the elements that must not exist
* @return this
* @throws NullPointerException if {@code other} is null
* @throws IllegalArgumentException if the collection contains all of the elements in {@code other};
* nothing less, nothing more).
*/
CollectionVerifier doesNotContainExactly(Collection other);
/**
* Ensures that the actual value does not contain exactly the specified elements; nothing less, nothing more.
*
* @param other the elements that must not exist
* @param name the name of the collection
* @return this
* @throws NullPointerException if {@code other} or {@code name} are null
* @throws IllegalArgumentException if the collection contains all of the elements in {@code other};
* nothing less, nothing more. If {@code name} is empty.
*/
CollectionVerifier doesNotContainExactly(Collection other, String name);
/**
* Ensures that the actual value does not contain any of the specified elements.
*
* @param expected the elements that must not exist
* @return this
* @throws NullPointerException if {@code expected} is null
* @throws IllegalArgumentException if the collection contains any of {@code expected}
*/
CollectionVerifier doesNotContainAny(Collection expected);
/**
* Ensures that the actual value does not contain any of the specified elements.
*
* @param elements the elements that must not exist
* @param name the name of the elements
* @return this
* @throws NullPointerException if {@code elements} or {@code name} are null
* @throws IllegalArgumentException if the collection contains any of {@code elements}. If {@code name}
* is empty.
*/
CollectionVerifier doesNotContainAny(Collection elements, String name);
/**
* Ensures that the actual value does not contain all of the specified elements.
*
* @param expected the elements that must not exist
* @return this
* @throws NullPointerException if {@code expected} is null
* @throws IllegalArgumentException if the collection contains all of {@code expected}
*/
CollectionVerifier doesNotContainAll(Collection expected);
/**
* Ensures that the actual value does not contain all of the specified elements.
*
* @param elements the elements that must not exist
* @param name the name of the elements
* @return this
* @throws NullPointerException if {@code elements} or {@code name} are null
* @throws IllegalArgumentException if the collection contains all of {@code elements}. If {@code name}
* is empty.
*/
CollectionVerifier doesNotContainAll(Collection elements, String name);
/**
* Ensures that the actual value does not contain any duplicate elements.
*
* @return this
* @throws IllegalArgumentException if the collection contains any duplicate elements
*/
CollectionVerifier doesNotContainDuplicates();
/**
* Returns a verifier for the collection's size.
*
* @return a verifier for the collection's size
*/
PrimitiveNumberVerifier size();
/**
* Verifies nested requirements. This mechanism can be used to
* group related requirements.
*
* @param consumer verifies the collection's size
* @return this
*/
CollectionVerifier size(Consumer> consumer);
/**
* Returns a verifier for the actual value as an array.
*
* @param type the array type
* @return a verifier for the actual value as an array
*/
ArrayVerifier asArray(Class type);
/**
* Verifies nested requirements. This mechanism can be used to
* group related requirements.
*
* @param type the array type
* @param consumer verifies the actual value as an array
* @return this
*/
CollectionVerifier asArray(Class type, Consumer> consumer);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy