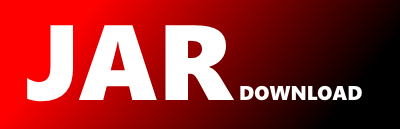
org.bitbucket.cowwoc.guava.stream.GuavaCollectorBuilder Maven / Gradle / Ivy
Show all versions of guava-jdk8 Show documentation
package org.bitbucket.cowwoc.guava.stream;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.HashBiMap;
import com.google.common.collect.HashMultimap;
import com.google.common.collect.HashMultiset;
import com.google.common.collect.ImmutableBiMap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.ImmutableMultiset;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSortedMap;
import com.google.common.collect.ImmutableSortedMultiset;
import com.google.common.collect.ImmutableSortedSet;
import com.google.common.collect.Multimap;
import com.google.common.collect.TreeMultiset;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector.Characteristics;
import static java.util.stream.Collector.Characteristics.UNORDERED;
/**
* Captures the type of the input elements.
*
* @param the type of the input elements
* @author Gili Tzabari
*/
public final class GuavaCollectorBuilder
{
/**
* Meant to be constructed by GuavaCollector.
*/
GuavaCollectorBuilder()
{
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into a {@code Multimap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultimapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return a builder for a {@code Multimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public MultimapCollectorBuilder> toMultimap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new MultimapCollectorBuilder<>(keyMapper, valueMapper, ArrayListMultimap::create,
input -> input, Characteristics.UNORDERED, Characteristics.IDENTITY_FINISH);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableMultimap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultimapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return a builder for an {@code ImmutableMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public
MultimapCollectorBuilder> toImmutableMultimap(
Function super T, ? extends K> keyMapper, Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new MultimapCollectorBuilder<>(keyMapper, valueMapper, ArrayListMultimap::create,
ImmutableMultimap::copyOf, Characteristics.UNORDERED);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableMultiset}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultisetCollectorBuilder#supplier(Supplier)} to replace it.
*
* @return a builder for an {@code ImmutableMultiset} collector
*/
public MultisetCollectorBuilder> toImmutableMultiset()
{
return new MultisetCollectorBuilder<>(HashMultiset::create, ImmutableMultiset::copyOf,
Characteristics.UNORDERED);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableMap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @return a builder for a {@code ImmutableMap} collector
* @throws NullPointerException if any of the arguments are null
*/
public MapCollectorBuilder> toImmutableMap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new MapCollectorBuilder<>(keyMapper, valueMapper, HashMap::new, ImmutableMap::copyOf,
Characteristics.UNORDERED);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableSortedMap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @param comparator a comparator that defines the sort order of the keys
* @return a builder for an {@code ImmutableSortedMap} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public MapCollectorBuilder>
toImmutableSortedMap(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper, Comparator comparator)
throws NullPointerException
{
return new MapCollectorBuilder<>(keyMapper, valueMapper, HashMap::new,
input -> ImmutableSortedMap.copyOf(input, comparator));
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableBiMap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultimapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @return a builder for an {@code ImmutableBiMap} collector
* @throws NullPointerException if any of the arguments are null
*/
public MapCollectorBuilder>
toImmutableBiMap(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new MapCollectorBuilder<>(keyMapper, valueMapper, HashBiMap::create,
ImmutableBiMap::copyOf, UNORDERED);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableList}.
*
* The default intermediate container is not thread-safe. Use
* {@link ListCollectorBuilder#supplier(Supplier)} to replace it.
*
* @return a builder for an {@code ImmutableList} collector
*/
public ListCollectorBuilder> toImmutableList()
{
return new ListCollectorBuilder<>(ArrayList::new, ImmutableList::copyOf);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableSet}.
*
* The default intermediate container is not thread-safe. Use
* {@link SetCollectorBuilder#supplier(Supplier)} to replace it.
*
* @return a builder for an {@code ImmutableSet} collector
*/
public SetCollectorBuilder> toImmutableSet()
{
return new SetCollectorBuilder<>(HashSet::new, ImmutableSet::copyOf);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableSortedSet}.
*
* The default intermediate container is not thread-safe. Use
* {@link SetCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param comparator a comparator that defines the sort order of the keys
* @return a builder for an {@code ImmutableSortedSet} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public SetCollectorBuilder> toImmutableSortedSet(
Comparator comparator)
throws NullPointerException
{
return new SetCollectorBuilder<>(HashSet::new, ImmutableSortedSet::copyOf);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableSortedSet}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultisetCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param comparator a comparator that defines the sort order of the elements
* @return a builder for an {@code ImmutableSortedSet} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public MultisetCollectorBuilder>
toImmutableSortedMultiset(Comparator comparator)
throws NullPointerException
{
return new MultisetCollectorBuilder<>(() -> TreeMultiset.create(comparator),
input -> ImmutableSortedMultiset.copyOf(comparator, input));
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableListMultimap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultimapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return a builder for an {@code ImmutableListMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public MultimapCollectorBuilder>
toImmutableListMultimap(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new MultimapCollectorBuilder<>(keyMapper, valueMapper, ArrayListMultimap::create,
ImmutableListMultimap::copyOf);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableSetMultimap}.
*
* The default intermediate container is not thread-safe. Use
* {@link MultimapCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return a builder for an {@code ImmutableListMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public ImmutableSetMultimapCollectorBuilder
toImmutableSetMultimap(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new ImmutableSetMultimapCollectorBuilder<>(keyMapper, valueMapper, HashMultimap::create,
UNORDERED);
}
/**
* Returns a builder for a {@code Collector} that accumulates elements into an
* {@code ImmutableTable}.
*
* The default intermediate container is not thread-safe. Use
* {@link TableCollectorBuilder#supplier(Supplier)} to replace it.
*
* @param the type of the table rows
* @param the type of the table columns
* @param the type of the table values
* @param rowMapper a function that transforms the input to table rows
* @param columnMapper a function that transforms the input to table columns
* @param valueMapper a function that transforms the input to table values
* @return a builder for an {@code ImmutableListMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public ImmutableTableCollectorBuilder
toImmutableTable(Function super T, ? extends R> rowMapper,
Function super T, ? extends C> columnMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return new ImmutableTableCollectorBuilder<>(rowMapper, columnMapper, valueMapper,
HashBasedTable::create, UNORDERED);
}
}