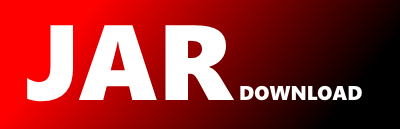
org.bitbucket.cowwoc.guava.stream.GuavaCollectors Maven / Gradle / Ivy
Show all versions of guava-jdk8 Show documentation
package org.bitbucket.cowwoc.guava.stream;
import com.google.common.collect.ImmutableBiMap;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.ImmutableMultiset;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSetMultimap;
import com.google.common.collect.ImmutableSortedMap;
import com.google.common.collect.ImmutableSortedMultiset;
import com.google.common.collect.ImmutableSortedSet;
import com.google.common.collect.ImmutableTable;
import com.google.common.collect.Multimap;
import java.util.Comparator;
import java.util.function.Function;
import java.util.stream.Collector;
/**
* Stream collectors for Guava collections.
*
* Builders offer more flexibility but require explicit type parameters. See
* http://stackoverflow.com/a/31383947/14731 for more information.
*
* @author Gili Tzabari
*/
public final class GuavaCollectors
{
/**
* Returns a for a {@code Collector} that accumulates elements into a {@code Multimap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return a {@code Multimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toMultimap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toMultimap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableMultimap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return an {@code ImmutableMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableMultimap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableMultimap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableMultiset}.
*
* @param the type of the input elements
* @return an {@code ImmutableMultiset} collector
*/
public static Collector> toImmutableMultiset()
{
return GuavaCollectors.builder().toImmutableMultiset().build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableMap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @return a {@code ImmutableMap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableMap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableMap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableSortedMap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @param comparator a comparator that defines the sort order of the keys
* @return an {@code ImmutableSortedMap} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableSortedMap(
Function super T, ? extends K> keyMapper, Function super T, ? extends V> valueMapper,
Comparator comparator)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableSortedMap(keyMapper, valueMapper, comparator).
build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableBiMap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the map keys
* @param valueMapper a function that transforms the map values
* @return an {@code ImmutableBiMap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableBiMap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableBiMap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableList}.
*
*
* @param the type of the input elements
* @return an {@code ImmutableList} collector
*/
public static Collector> toImmutableList()
{
return GuavaCollectors.builder().toImmutableList().build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableSet}.
*
* @param the type of the input elements
* @return an {@code ImmutableList} collector
*/
public static Collector> toImmutableSet()
{
return GuavaCollectors.builder().toImmutableSet().build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableSortedSet}.
*
* @param the type of the input elements
* @param comparator a comparator that defines the sort order of the keys
* @return a {@code ImmutableSortedSet} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableSortedSet(
Comparator comparator)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableSortedSet(comparator).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableSortedSet}.
*
* @param the type of the input elements
* @param comparator a comparator that defines the sort order of the elements
* @return a {@code ImmutableSortedSet} collector
* @see Comparator#naturalOrder()
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableSortedMultiset(
Comparator comparator)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableSortedMultiset(comparator).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableListMultimap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return an {@code ImmutableListMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableListMultimap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableListMultimap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableSetMultimap}.
*
* @param the type of the input elements
* @param the type of the map keys
* @param the type of the map values
* @param keyMapper a function that transforms the input to map keys
* @param valueMapper a function that transforms the input to map values
* @return an {@code ImmutableSetMultimap} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableSetMultimap(
Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableSetMultimap(keyMapper, valueMapper).build();
}
/**
* Returns a {@code Collector} that accumulates elements into an {@code ImmutableTable}.
*
* @param the type of the input elements
* @param the type of the table rows
* @param the type of the table columns
* @param the type of the table values
* @param rowMapper a function that transforms the input to table rows
* @param columnMapper a function that transforms the input to table columns
* @param valueMapper a function that transforms the input to table values
* @return an {@code ImmutableTable} collector
* @throws NullPointerException if any of the arguments are null
*/
public static Collector> toImmutableTable(
Function super T, ? extends R> rowMapper,
Function super T, ? extends C> columnMapper,
Function super T, ? extends V> valueMapper)
throws NullPointerException
{
return GuavaCollectors.builder().toImmutableTable(rowMapper, columnMapper, valueMapper).
build();
}
/**
* @param the type of input elements
* @return a new collector builder
*/
public static GuavaCollectorBuilder builder()
{
return new GuavaCollectorBuilder<>();
}
/**
* Prevent construction.
*/
private GuavaCollectors()
{
}
}