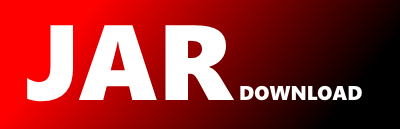
org.bitbucket.cowwoc.guava.stream.ImmutableSetMultimapCollectorBuilder Maven / Gradle / Ivy
Show all versions of guava-jdk8 Show documentation
package org.bitbucket.cowwoc.guava.stream;
import com.google.common.collect.ImmutableSetMultimap;
import com.google.common.collect.Multimap;
import java.util.Comparator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Collector.Characteristics;
import org.bitbucket.cowwoc.preconditions.Preconditions;
/**
* Builds a stream {@link Collector} that returns an {@link ImmutableSetMultimap}.
*
* @author Gili Tzabari
* @param the type of the input elements
* @param the type of keys stored in the map
* @param the type of values stored in the map
*/
public final class ImmutableSetMultimapCollectorBuilder
extends MultimapCollectorBuilder>
{
private Comparator super K> keysComparator;
private Comparator super V> valuesComparator;
/**
* Creates a new builder.
*
* @param keyMapper a function that transforms the input into map keys
* @param valueMapper a function that transforms the input into map values
* @param supplier a function which returns a new, empty {@code Multimap} into which
* intermediate results will be added
* @param characteristics the collector characteristics
* @throws NullPointerException if any of the arguments are null
*/
public ImmutableSetMultimapCollectorBuilder(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper, Supplier> supplier,
Characteristics... characteristics)
{
super(keyMapper, valueMapper, supplier, ImmutableSetMultimap::copyOf, characteristics);
}
/**
* Sorts the map keys.
*
* WARNING: Invoking this method overrides the
* {@link #finisher(java.util.function.Function) finisher}.
*
* @param comparator specifies the key sorting order
* @return this
* @throws NullPointerException if comparator is null
*/
public ImmutableSetMultimapCollectorBuilder orderKeysBy(
Comparator super K> comparator) throws NullPointerException
{
Preconditions.requireThat(comparator, "comparator").isNotNull();
this.keysComparator = comparator;
return this;
}
/**
* @return the key sorting order
*/
public Comparator super K> orderKeysBy()
{
return keysComparator;
}
/**
* Sorts the map values.
*
* WARNING: Invoking this method overrides the
* {@link #finisher(java.util.function.Function) finisher}.
*
* @param comparator specifies the value sorting order
* @return this
* @throws NullPointerException if comparator is null
*/
public ImmutableSetMultimapCollectorBuilder orderValuesBy(
Comparator super V> comparator) throws NullPointerException
{
Preconditions.requireThat(comparator, "comparator").isNotNull();
this.valuesComparator = comparator;
return this;
}
/**
* @return the value sorting order
*/
public Comparator super V> orderValuesBy()
{
return valuesComparator;
}
/**
* {@inheritDoc} WARNING: Invoking this method overrides the
* {@link #orderKeysBy(java.util.Comparator) key} and
* {@link #orderValuesBy(java.util.Comparator) value} sort order.
*/
@Override
public ImmutableSetMultimapCollectorBuilder finisher(
Function, ImmutableSetMultimap> finisher)
throws NullPointerException
{
this.keysComparator = null;
this.valuesComparator = null;
super.finisher(finisher);
return this;
}
/**
* @return a new collector
*/
public Collector> build()
{
if (keysComparator != null || valuesComparator != null)
{
super.finisher(value ->
{
ImmutableSetMultimap.Builder builder = ImmutableSetMultimap.builder().
putAll(value);
if (keysComparator != null)
builder.orderKeysBy(keysComparator);
if (valuesComparator != null)
builder.orderValuesBy(valuesComparator);
return builder.build();
});
}
return super.build();
}
}