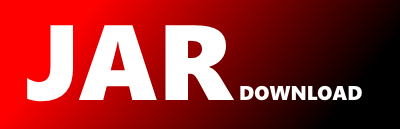
org.bitbucket.cowwoc.guava.stream.ImmutableTableCollectorBuilder Maven / Gradle / Ivy
Show all versions of guava-jdk8 Show documentation
package org.bitbucket.cowwoc.guava.stream;
import com.google.common.collect.ImmutableTable;
import com.google.common.collect.Table;
import java.util.Comparator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Collector.Characteristics;
import org.bitbucket.cowwoc.preconditions.Preconditions;
/**
* Builds a stream {@link Collector} that returns an {@link ImmutableTable}.
*
* @author Gili Tzabari
* @param the type of the input elements
* @param the type of rows stored in the table
* @param the type of columns stored in the table
* @param the type of values stored in the table
*/
public final class ImmutableTableCollectorBuilder
extends TableCollectorBuilder>
{
private Comparator super R> rowSortingOrder;
private Comparator super C> columnSortingOrder;
/**
* Creates a new builder.
*
* @param rowMapper a function that transforms the input into table rows
* @param columnMapper a function that transforms the input into table columns
* @param valueMapper a function that transforms the input into table values
* @param supplier a function which returns a new, empty {@code Table} into which
* intermediate results will be added
* @param characteristics the collector characteristics
* @throws NullPointerException if any of the arguments are null
*/
public ImmutableTableCollectorBuilder(Function super T, ? extends R> rowMapper,
Function super T, ? extends C> columnMapper, Function super T, ? extends V> valueMapper,
Supplier
> supplier, Characteristics... characteristics)
{
super(rowMapper, columnMapper, valueMapper, supplier, ImmutableTable::copyOf, characteristics);
}
/**
* Sorts the table rows.
*
* WARNING: Invoking this method overrides the
* {@link #finisher(java.util.function.Function) finisher}.
*
* @param comparator specifies the row sorting order
* @return this
* @throws NullPointerException if comparator is null
*/
public ImmutableTableCollectorBuilder orderRowsBy(
Comparator super R> comparator) throws NullPointerException
{
Preconditions.requireThat(comparator, "comparator").isNotNull();
this.rowSortingOrder = comparator;
return this;
}
/**
* @return the row sorting order
*/
public Comparator super R> orderRowsBy()
{
return rowSortingOrder;
}
/**
* Sorts the table columns.
*
* WARNING: Invoking this method overrides the
* {@link #finisher(java.util.function.Function) finisher}.
*
* @param comparator specifies the column sorting order
* @return this
* @throws NullPointerException if comparator is null
*/
public ImmutableTableCollectorBuilder orderColumnsBy(
Comparator super C> comparator) throws NullPointerException
{
Preconditions.requireThat(comparator, "comparator").isNotNull();
this.columnSortingOrder = comparator;
return this;
}
/**
* @return the column sorting order
*/
public Comparator super C> orderColumnsBy()
{
return columnSortingOrder;
}
/**
* {@inheritDoc} WARNING: Invoking this method overrides the
* {@link #orderRowsBy(java.util.Comparator) row} and
* {@link #orderColumnsBy(java.util.Comparator) column} sort order.
*/
@Override
public ImmutableTableCollectorBuilder finisher(
Function, ImmutableTable> finisher)
throws NullPointerException
{
this.rowSortingOrder = null;
this.columnSortingOrder = null;
super.finisher(finisher);
return this;
}
@Override
public Collector> build()
{
if (rowSortingOrder != null || columnSortingOrder != null)
{
super.finisher(value ->
{
ImmutableTable.Builder builder = ImmutableTable.builder().
putAll(value);
if (rowSortingOrder != null)
builder.orderRowsBy(rowSortingOrder);
if (columnSortingOrder != null)
builder.orderColumnsBy(columnSortingOrder);
return builder.build();
});
}
return super.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy