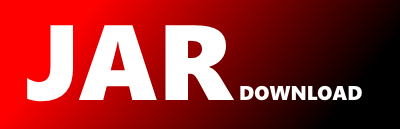
org.bitbucket.cowwoc.guava.stream.MultimapCollectorBuilder Maven / Gradle / Ivy
Show all versions of guava-jdk8 Show documentation
package org.bitbucket.cowwoc.guava.stream;
import com.google.common.collect.Multimap;
import java.util.function.BiConsumer;
import java.util.function.BinaryOperator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
import java.util.stream.Collector.Characteristics;
import org.bitbucket.cowwoc.preconditions.Preconditions;
/**
* Builds a stream {@link Collector} that returns a {@link Multimap}.
*
* @author Gili Tzabari
* @param the type of the input elements
* @param the type of keys stored in the map
* @param the type of values stored in the map
* @param the output type of the collector
*/
public class MultimapCollectorBuilder>
{
private final Function super T, ? extends K> keyMapper;
private final Function super T, ? extends V> valueMapper;
private Supplier> supplier;
private Function, R> finisher;
private Characteristics[] characteristics;
/**
* Creates a new builder.
*
* @param keyMapper a function that transforms the input into map keys
* @param valueMapper a function that transforms the input into map values
* @param supplier a function which returns a new, empty {@code Multimap} into which
* intermediate results will be added
* @param finisher a function that transforms the intermediate {@code Multimap} into the
* final result
* @param characteristics the collector characteristics
* @throws NullPointerException if any of the arguments are null
*/
public MultimapCollectorBuilder(Function super T, ? extends K> keyMapper,
Function super T, ? extends V> valueMapper, Supplier> supplier,
Function, R> finisher, Characteristics... characteristics)
{
Preconditions.requireThat(keyMapper, "keyMapper").isNotNull();
Preconditions.requireThat(valueMapper, "valueMapper").isNotNull();
Preconditions.requireThat(supplier, "supplier").isNotNull();
Preconditions.requireThat(finisher, "finisher").isNotNull();
Preconditions.requireThat(characteristics, "characteristics").isNotNull();
this.keyMapper = keyMapper;
this.valueMapper = valueMapper;
this.supplier = supplier;
this.finisher = finisher;
this.characteristics = characteristics;
}
/**
* @return a function that transforms the input into map keys
*/
public Function super T, ? extends K> keyMapper()
{
return keyMapper;
}
/**
* @return a function that transforms the input into map values
*/
public Function super T, ? extends V> valueMapper()
{
return valueMapper;
}
/**
* @return a function which returns a new, empty {@code Multimap} into which intermediate results
* will be added
*/
public Supplier> supplier()
{
return supplier;
}
/**
* @param supplier a function which returns a new, empty {@code Multimap} into which intermediate
* results will be added
* @return this
* @throws NullPointerException if supplier is null
*/
public MultimapCollectorBuilder supplier(Supplier> supplier)
throws NullPointerException
{
Preconditions.requireThat(supplier, "supplier").isNotNull();
this.supplier = supplier;
return this;
}
/**
* @return a function that transforms the intermediate {@code Multimap} into the final result
*/
public Function, R> finisher()
{
return finisher;
}
/**
* Sets a function that transforms the intermediate {@code Multimap} into the final result.
*
* Make sure to update the collector
* {@link #characteristics(java.util.stream.Collector.Characteristics[]) characteristics} if
* necessary.
*
* @param finisher a function that transforms the intermediate {@code Multimap} into the final
* result
* @return this
* @throws NullPointerException if finisher is null
*/
public MultimapCollectorBuilder finisher(Function, R> finisher)
throws NullPointerException
{
Preconditions.requireThat(finisher, "finisher").isNotNull();
this.finisher = finisher;
return this;
}
/**
* @return the collector characteristics
*/
@SuppressWarnings("ReturnOfCollectionOrArrayField")
public Characteristics[] characteristics()
{
return characteristics;
}
/**
* @param characteristics the collector characteristics
* @return this
* @throws NullPointerException if characteristics is null
*/
@SuppressWarnings("AssignmentToCollectionOrArrayFieldFromParameter")
public MultimapCollectorBuilder characteristics(
Characteristics... characteristics) throws NullPointerException
{
Preconditions.requireThat(characteristics, "characteristics").isNotNull();
this.characteristics = characteristics;
return this;
}
/**
* @return a new collector
*/
public Collector build()
{
BiConsumer, T> accumulator = (map, entry) ->
{
K key = keyMapper.apply(entry);
if (key == null)
throw new IllegalArgumentException("keyMapper(" + entry + ") returned null");
V value = valueMapper.apply(entry);
if (value == null)
throw new IllegalArgumentException("valueMapper(" + entry + ") returned null");
map.put(key, value);
};
BinaryOperator> combiner = (left, right) ->
{
left.putAll(right);
return left;
};
return Collector.of(supplier, accumulator, combiner, finisher, characteristics);
}
}