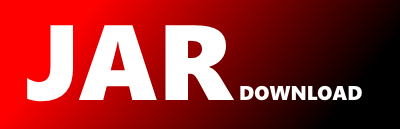
org.bitbucket.cowwoc.requirements.guava.Requirements Maven / Gradle / Ivy
/*
* Copyright 2014 Gili Tzabari.
* Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package org.bitbucket.cowwoc.requirements.guava;
import com.google.common.collect.Multimap;
import org.bitbucket.cowwoc.requirements.core.Configuration;
import org.bitbucket.cowwoc.requirements.internal.guava.impl.GuavaVerifiersImpl;
/**
* An entry point for verifying API requirements.
*
* The assertion status of the {@link Configuration} class determines whether {@code assertThat()}
* carries out a verification or does nothing.
*
* This class is immutable.
*
* @since 3.0.0
* @author Gili Tzabari
* @see GuavaVerifiers
*/
@SuppressWarnings(
{
"AssertWithSideEffects", "NestedAssignment"
})
public final class Requirements
{
private static final GuavaVerifiers DELEGATE = new GuavaVerifiersImpl();
/**
* @return true if assertions are enabled for this class
*/
public static boolean assertionsAreEnabled()
{
return DELEGATE.assertionsAreEnabled();
}
/**
* Verifies a {@code Multimap}.
*
* @param the type of key in the multimap
* @param the type of value in the multimap
* @param actual the actual value of the parameter
* @param name the name of the parameter
* @return a verifier for the parameter
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} is empty
*/
public static MultimapVerifier requireThat(Multimap actual, String name)
{
return DELEGATE.requireThat(actual, name);
}
/**
* Same as {@link #requireThat(Multimap, String)} but does nothing if assertions are disabled for
* this class.
*
* @param the type of key in the multimap
* @param the type of value in the multimap
* @param actual the actual value of the parameter
* @param name the name of the parameter
* @return a verifier for the parameter
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} is empty
*/
public static MultimapVerifier assertThat(Multimap actual, String name)
{
return DELEGATE.assertThat(actual, name);
}
/**
* Prevent construction.
*/
private Requirements()
{
}
}