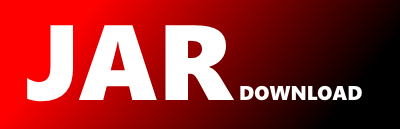
org.jenetics.util.ISeq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.jenetics Show documentation
Show all versions of org.jenetics Show documentation
Jenetics - Java Genetic Algorithm Library
/*
* Java Genetic Algorithm Library (jenetics-3.1.0).
* Copyright (c) 2007-2015 Franz Wilhelmstötter
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Author:
* Franz Wilhelmstötter ([email protected])
*/
package org.jenetics.util;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collector;
/**
* Immutable, ordered, fixed sized sequence.
*
* @see MSeq
*
* @author Franz Wilhelmstötter
* @since 1.0
* @version 3.0
*/
public interface ISeq
extends
Seq,
Copyable>
{
@Override
public ISeq subSeq(final int start, final int end);
@Override
public ISeq subSeq(final int start);
@Override
public ISeq map(final Function super T, ? extends B> mapper);
/**
* Return a shallow copy of this sequence. The sequence elements are not
* cloned.
*
* @return a shallow copy of this sequence.
*/
@Override
public MSeq copy();
/* *************************************************************************
* Some static factory methods.
* ************************************************************************/
/**
* Returns a {@code Collector} that accumulates the input elements into a
* new {@code ISeq}.
*
* @param the type of the input elements
* @return a {@code Collector} which collects all the input elements into an
* {@code ISeq}, in encounter order
*/
public static Collector> toISeq() {
return Collector.of(
(Supplier>)ArrayList::new,
List::add,
(left, right) -> { left.addAll(right); return left; },
ISeq::of
);
}
/**
* Create a new {@code ISeq} from the given values.
*
* @param the element type
* @param values the array values.
* @return a new {@code ISeq} with the given values.
* @throws NullPointerException if the {@code values} array is {@code null}.
*/
@SafeVarargs
public static ISeq of(final T... values) {
return MSeq.of(values).toISeq();
}
/**
* Create a new {@code ISeq} from the given values.
*
* @param the element type
* @param values the array values.
* @return a new {@code ISeq} with the given values.
* @throws NullPointerException if the {@code values} array is {@code null}.
*/
@SuppressWarnings("unchecked")
public static ISeq of(final Iterable extends T> values) {
return values instanceof ISeq>
? (ISeq)values
: values instanceof MSeq>
? ((MSeq)values).toISeq()
: MSeq.of(values).toISeq();
}
public static ISeq of(Supplier extends T> supplier, final int length) {
return MSeq.ofLength(length).fill(supplier).toISeq();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy