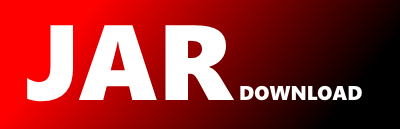
org.jenetics.stat.MinMax Maven / Gradle / Ivy
Show all versions of org.jenetics Show documentation
/*
* Java Genetic Algorithm Library (jenetics-3.2.0).
* Copyright (c) 2007-2015 Franz Wilhelmstötter
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Author:
* Franz Wilhelmstötter ([email protected])
*/
package org.jenetics.stat;
import static java.util.Objects.requireNonNull;
import java.util.Comparator;
import java.util.function.Consumer;
import java.util.stream.Collector;
/**
* This consumer class is used for calculating the min and max value
* according to the given {@code Comparator}.
*
* This class is designed to work with (though does not require) streams. For
* example, you can compute minimum and maximum values with:
*
{@code
* final Stream stream = ...
* final MinMax minMax = stream.collect(
* MinMax::of,
* MinMax::accept,
* MinMax::combine
* );
* }
*
*
* Implementation note:
* This implementation is not thread safe. However, it is safe to use on a
* parallel stream, because the parallel implementation of
* {@link java.util.stream.Stream#collect Stream.collect()}provides the
* necessary partitioning, isolation, and merging of results for safe and
* efficient parallel execution.
*
* @author Franz Wilhelmstötter
* @since 3.0
* @version 3.0
*/
public final class MinMax implements Consumer {
private final Comparator super C> _comparator;
private C _min;
private C _max;
private long _count = 0L;
private MinMax(final Comparator super C> comparator) {
_comparator = requireNonNull(comparator);
}
/**
* Accept the element for min-max calculation.
*
* @param object the element to use for min-max calculation
*/
@Override
public void accept(final C object) {
_min = min(_comparator, _min, object);
_max = max(_comparator, _max, object);
++_count;
}
/**
* Combine two {@code MinMax} objects.
*
* @param other the other {@code MinMax} object to combine
* @return {@code this}
* @throws java.lang.NullPointerException if the {@code other} object is
* {@code null}.
*/
public MinMax combine(final MinMax other) {
_min = min(_comparator, _min, other._min);
_max = max(_comparator, _max, other._max);
_count += other._count;
return this;
}
/**
* Return the current minimal object or {@code null} if no element has been
* accepted yet.
*
* @return the current minimal object
*/
public C getMin() {
return _min;
}
/**
* Return the current maximal object or {@code null} if no element has been
* accepted yet.
*
* @return the current maximal object
*/
public C getMax() {
return _max;
}
/**
* Returns the count of values recorded.
*
* @return the count of recorded values
*/
public long getCount() {
return _count;
}
/* *************************************************************************
* Some static helper methods.
* ************************************************************************/
/**
* Return the minimum of two values, according the given comparator.
* {@code null} values are allowed.
*
* @param comp the comparator used for determining the min value
* @param a the first value to compare
* @param b the second value to compare
* @param the type of the compared objects
* @return the minimum value, or {@code null} if both values are {@code null}.
* If only one value is {@code null}, the non {@code null} values is
* returned.
*/
public static T
min(final Comparator super T> comp, final T a, final T b) {
return a != null ? b != null ? comp.compare(a, b) <= 0 ? a : b : a : b;
}
/**
* Return the maximum of two values, according the given comparator.
* {@code null} values are allowed.
*
* @param comp the comparator used for determining the max value
* @param a the first value to compare
* @param b the second value to compare
* @param the type of the compared objects
* @return the maximum value, or {@code null} if both values are {@code null}.
* If only one value is {@code null}, the non {@code null} values is
* returned.
*/
public static T
max(final Comparator super T> comp, final T a, final T b) {
return a != null ? b != null ? comp.compare(a, b) >= 0 ? a : b : a : b;
}
/* *************************************************************************
* Some static factory methods.
* ************************************************************************/
/**
* Return a {@code Collector} which calculates the minimum and maximum value.
* The given {@code comparator} is used for comparing two objects.
*
* {@code
* final Comparator comparator = ...
* final Stream stream = ...
* final MinMax moments = stream
* .collect(doubleMoments.toMinMax(comparator));
* }
*
* @param comparator the {@code Comparator} to use
* @param the type of the input elements
* @return a {@code Collector} implementing the min-max reduction
* @throws java.lang.NullPointerException if the given {@code mapper} is
* {@code null}
*/
public static
Collector> toMinMax(final Comparator super T> comparator) {
return Collector.of(
() -> MinMax.of(comparator),
MinMax::accept,
MinMax::combine
);
}
/**
* Return a {@code Collector} which calculates the minimum and maximum value.
* The reducing objects must be comparable.
*
* {@code
* final Stream stream = ...
* final MinMax moments = stream
* .collect(doubleMoments.toMinMax(comparator));
* }
*
* @param the type of the input elements
* @return a {@code Collector} implementing the min-max reduction
* @throws java.lang.NullPointerException if the given {@code mapper} is
* {@code null}
*/
public static >
Collector> toMinMax() {
return toMinMax((a, b) -> a.compareTo(b));
}
/**
* Create a new {@code MinMax} consumer with the given
* {@link java.util.Comparator}.
*
* @param comparator the comparator used for comparing two elements
* @param the element type
* @return a new {@code MinMax} consumer
* @throws java.lang.NullPointerException if the {@code comparator} is
* {@code null}.
*/
public static MinMax of(final Comparator super T> comparator) {
return new MinMax<>(comparator);
}
/**
* Create a new {@code MinMax} consumer.
*
* @param the element type
* @return a new {@code MinMax} consumer
*/
public static > MinMax of() {
return of((a, b) -> a.compareTo(b));
}
}