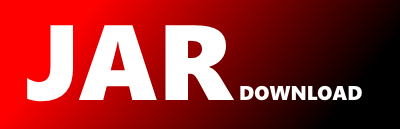
mentalState.converter.GOALMentalStateConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of msInterface Show documentation
Show all versions of msInterface Show documentation
Defines an interface and generic functionality of a mental state, independent from a specific KR language that is used to represent the content of a mental state.
/**
* The GOAL Mental State. Copyright (C) 2014 Koen Hindriks.
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see .
*/
package mentalState.converter;
import java.io.Serializable;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Deque;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import krTools.language.DatabaseFormula;
import krTools.language.Update;
import languageTools.program.agent.Module;
import mentalState.GoalBase;
import mentalState.MentalStateWithEvents;
import mentalState.error.MSTDatabaseException;
import mentalState.error.MSTQueryException;
/**
* Represents a mental state converter. The task of instances of this class is
* to translate a {@link MentalStateInterface} object to the binary
* representation, and back. Each instance has a single
* {@link MentalStateInterface} object associated with it, whose contents often
* changes.
* Serialization
*
* The serialization is currently made to support loading a previously learned
* behaviour (see {@link FileLearner}) for execution of a GOAL program. A
* de-serialized {@link GOALMentalStateConverter} is partial: {@link Module} and
* {@link GoalBase} are not restored fully. Also, we don't save all info stored
* here that is relevant for learning. Therefore, a de-serialized
* {@link GOALMentalStateConverter} can not be used to resume model checking or
* resume learning.
*
*/
public class GOALMentalStateConverter implements Serializable {
/** Auto-generated serial version UID */
private static final long serialVersionUID = 6591769350769988803L;
/**
* The conversion universe that this converter has access to, which contains all
* beliefs and goals that have occurred in the mental state in the past.
*/
private GOALConversionUniverse universe;
/************************** Public methods ********************************/
/**
* Creates a converter for the specified mental state.
*/
public GOALMentalStateConverter() {
this.universe = new GOALConversionUniverse();
}
/**
* Returns the universe associated with this converter.
*
* @return The universe.
*/
public GOALConversionUniverse getUniverse() {
return this.universe;
}
/**
* @param filter set of predicate signatures. Used to select the relevant
* beliefs from the beliefbase.
* @return {@link GOALState} created from given mentalState beliefs and goals.
* @throws MSTDatabaseException
* @throws MSTQueryException
*/
public GOALState translate(MentalStateWithEvents mentalState, Set belieffilter, Set goalfilter)
throws MSTDatabaseException, MSTQueryException {
Set beliefs = filteredBeliefs(mentalState, belieffilter);
Deque goalBaseStack = filteredGoals(mentalState, goalfilter);
return translate(beliefs, goalBaseStack);
}
/**
*
* @param mentalState the {@link MentalStateWithEvents}
* @param stateidMap a map of pairs, where state is a
* {@link GOALState} turned into string, and nr is a unique
* Integer ID for this state. This map can be modified
* @param statestrMap a map of where state description
* is a textual description of the mentalstate, and nr is a
* unique Integer ID for this state. This map can be
* modified
* @param belieffilter set of requested belief predicate signatures. Used to
* select the relevant beliefs from the beliefbase.
* @param goalfilter set of requested goal predicate signatures. Used to
* select the relevant beliefs from the goalbase.
* @return the MentalState translated to a state vector string. The returned
* state is also added to the list of known states with a unique ID, if
* it is not already there.
* @throws MSTDatabaseException
* @throws MSTQueryException
*/
public String getStateString(MentalStateWithEvents mentalState, Map stateidMap,
Map statestrMap, Set belieffilter, Set goalfilter)
throws MSTDatabaseException, MSTQueryException {
String state = translate(mentalState, belieffilter, goalfilter).toString();
if (!stateidMap.containsKey(state)) {
stateidMap.put(state, new Integer(stateidMap.size() + 1));
}
if (!statestrMap.containsKey(state)) {
String s = "";
Set beliefs = filteredBeliefs(mentalState, belieffilter);
List strset = new ArrayList<>(beliefs.size());
for (DatabaseFormula dbf : beliefs) {
strset.add(dbf.toString());
}
Collections.sort(strset);
s += strset.toString() + " ";
for (GoalBase base : filteredGoals(mentalState, goalfilter)) {
s += base.getName() + ":\n";
s += base.showContents() + "\n";
}
statestrMap.put(state, s);
}
return state;
}
/**
* Translates the current contents of {@link #mentalState} to a string
* representation.
*
* @param indent The margin (i.e. number of white spaces) that the string to be
* constructed should adhere to.
* @return The string representation of {@link #mentalState}.
* @throws MSTDatabaseException
* @throws MSTQueryException
*/
public String toString(MentalStateWithEvents mentalState, int indent)
throws MSTDatabaseException, MSTQueryException {
/* Process belief base */
Set formulas = new LinkedHashSet<>(mentalState.getBeliefs().size());
for (DatabaseFormula formula : mentalState.getBeliefs()) {
formulas.add(formula);
}
/* Process specified indentation to whitespace */
String tab = "";
for (int i = 0; i < indent; i++) {
tab += " ";
}
/* Build binary representation and belief base */
String string = tab + "Bit Repres.: " + translate(mentalState).toString() + "\n" + tab + "Belief base: "
+ formulas;
/* Build stack of goal bases */
int depth = 0;
for (GoalBase goalBase : mentalState.getAttentionStack()) {
String goals = "";
for (Update goal : goalBase.getUpdates()) {
goals += "[" + goal.toString() + "] ";
}
string += "\n" + tab + "Goal base " + depth + ": \"" + goalBase.getName() + "\" " + goals + "";
depth++;
}
/* Return */
return string;
}
/********************* support functions **********************/
/**
* Translates the contents of {@link #mentalState} to a binary representation.
*
* This method is used only by model checker which also uses this class to store
* mental state and focus stack...
*
* @return
* @throws MSTQueryException
* @throws MSTDatabaseException
*/
private GOALState translate(MentalStateWithEvents mentalState) throws MSTDatabaseException, MSTQueryException {
Set beliefs = mentalState.getBeliefs();
return translate(beliefs, mentalState.getAttentionStack());
}
/**
* Returns a filtered set of beliefs whose signature matches one of those in
* filter.
*
* @param mentalState
* @param filter A list of predicate signatures.
* @return all belief predicates from mentalState that are in the filter list.
* @throws MSTQueryException
* @throws MSTDatabaseException
*/
private static Set filteredBeliefs(MentalStateWithEvents mentalState, Set filter)
throws MSTDatabaseException, MSTQueryException {
Set beliefs = new HashSet<>(mentalState.getBeliefs());
Iterator iterator = beliefs.iterator();
while (iterator.hasNext()) {
if (!filter.contains(iterator.next().getSignature())) {
iterator.remove();
}
}
return beliefs;
}
private static Deque filteredGoals(MentalStateWithEvents mentalState, Set filter)
throws MSTDatabaseException, MSTQueryException {
Deque original = mentalState.getAttentionStack();
Deque returned = new ArrayDeque<>(original.size());
for (GoalBase base : original) {
Set updates = base.getUpdates(); // this is a fresh set
Iterator iterator = updates.iterator();
while (iterator.hasNext()) {
if (!filter.contains(iterator.next().getSignature())) {
iterator.remove();
}
}
GoalBase clone = mentalState.getOwnModel().createGoalBase(base.getName());
clone.setGoals(updates);
returned.add(clone);
}
return returned;
}
/**
* Translates the contents of the mental state to a binary representation.
*
* @return The binary representation.
*/
private GOALState translate(Set beliefs, Deque goalBaseStack) {
GOALState q = new GOALState(this);
// Convert beliefs.
for (DatabaseFormula formula : beliefs) {
GOALCE_Belief belief = new GOALCE_Belief(formula);
int index = this.universe.addIfNotContains(belief);
q.set(index);
}
// Convert goals.
int depth = 0;
for (GoalBase goalBase : goalBaseStack) {
// Add goals per goal base on the attention stack.
for (Update update : goalBase.getUpdates()) {
GOALCE_GoalAtDepth goal = new GOALCE_GoalAtDepth(update, depth);
int index = this.universe.addIfNotContains(goal);
q.set(index);
}
// Increment depth.
depth++;
}
// Add foci. TODO Check.
depth = 0;
for (GoalBase module : goalBaseStack) {
GOALCE_FocusAtDepth focus = new GOALCE_FocusAtDepth(module, depth);
int index = this.universe.addIfNotContains(focus);
q.set(index);
// Increment depth.
depth++;
}
// Return GOALState.
return q;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy