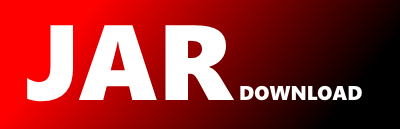
nl.tudelft.goal.SimpleIDE.actions.KillAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simpleidemodules Show documentation
Show all versions of simpleidemodules Show documentation
An IDE for GOAL based on JEdit.
The newest version!
/**
* GOAL interpreter that facilitates developing and executing GOAL multi-agent
* programs. Copyright (C) 2011 K.V. Hindriks, W. Pasman
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see .
*/
package nl.tudelft.goal.SimpleIDE.actions;
import java.awt.event.ActionEvent;
import java.util.List;
import javax.swing.SwingWorker;
import javax.swing.tree.TreeNode;
import goal.core.runtime.RuntimeManager;
import goal.core.runtime.service.environmentport.EnvironmentPort;
import goal.tools.IDEGOALInterpreter;
import goal.tools.debugger.IDEDebugger;
import goal.tools.debugger.SteppingDebugger.RunMode;
import goal.tools.errorhandling.Resources;
import goal.tools.errorhandling.Warning;
import goal.tools.errorhandling.WarningStrings;
import goal.tools.errorhandling.exceptions.GOALBug;
import goal.tools.errorhandling.exceptions.GOALException;
import languageTools.program.agent.AgentId;
import nl.tudelft.goal.SimpleIDE.EditManager;
import nl.tudelft.goal.SimpleIDE.IDEMainPanel;
import nl.tudelft.goal.SimpleIDE.IDENode;
import nl.tudelft.goal.SimpleIDE.IDEState;
import nl.tudelft.goal.SimpleIDE.IconFactory;
import nl.tudelft.goal.SimpleIDE.ProcessNode;
import nl.tudelft.goal.SimpleIDE.TextEditorInterface;
/**
* kill selected process node.
*
* @author W.Pasman
* @modified W.Pasman 20jun2011 into action
*/
public class KillAction extends GOALAction {
private static final long serialVersionUID = -8561815023910281587L;
public KillAction() {
setIcon(IconFactory.KILL_PROCESS.getIcon());
setShortcut('K');
}
@Override
public void eventOccured(IDEState currentState, Object evt) {
List extends IDENode> selection = currentState.getSelectedProcessNodes();
if (selection.isEmpty() || currentState.getViewMode() != IDEMainPanel.DEBUG_VIEW) {
setActionEnabled(false);
return;
}
IDENode node = selection.get(0);
switch (node.getType()) {
case MAS_PROCESS:
setDescription("Kill multi-agent system"); //$NON-NLS-1$
setActionEnabled(true);
break; // always, to always provide opportunity to
// terminate run environment
case AGENT_PROCESS:
setDescription("Kill agent"); //$NON-NLS-1$
setActionEnabled(((ProcessNode) node).getProcessRunMode() != RunMode.KILLED);
break;
case ENVIRONMENT_PROCESS:
setDescription("Kill environment"); //$NON-NLS-1$
setActionEnabled(((ProcessNode) node).getProcessRunMode() != RunMode.KILLED);
break;
default:
setActionEnabled(false);
}
}
@Override
protected void execute(TreeNode selectedNode, ActionEvent ae) throws GOALException {
killProcessNode((ProcessNode) selectedNode);
}
/**
* kill selected process node.
*
* @param node
* is node to be killed
* @throws GOALException
* @throws Exception
*/
private void killProcessNode(final ProcessNode node) throws GOALException {
if (node.getProcessRunMode() == RunMode.KILLED) {
return;
}
switch (node.getType()) {
case AGENT_PROCESS:
String agentName = node.toString();
// kill agent to avoid further call backs.
// introspectors will notice the kill and close panels if
// necessasry.
try {
RuntimeManager manager = getCurrentState().getRuntime();
manager.stopAgent(new AgentId(agentName));
} catch (Exception e) {
new Warning(String.format(Resources.get(WarningStrings.FAILED_AGENT_KILL), agentName), e).emit();
}
break;
case MAS_PROCESS:
// Close debug panel. Close all debug observers, including those
// without corresponding panel.
// We can not just keep closing all DebugObservers until no
// DebugObservers are left,
// because agents in PAUSE mode will not be removed as observer
// (that would put the agent into RUN mode! TRAC 702).
// HACK we thus make a list of all agents running in the MAS and try
// to close process inspectors with those names.
// This is a hack because we *might* (in future) have multiple MASs
// run at same time.
// Shut down runtime environment.
try {
getCurrentState().getRuntime().shutDown(true);
} catch (Exception e) {
new Warning(Resources.get(WarningStrings.FAILED_MAS_KILL), e).emit();
}
// close all panels: introspectors, sniffer; debug text panes are
// killed via events TODO: also close sniffer etc in similar manner
// List agentIds = ((RuntimeServiceManager) node
// .getUserObject()).getLocalAgentNames();
// for (AgentId id : agentIds) {
getIDE().getMainPanel().getDebugPanel().closeAll();
getIDE().getMainPanel().getProcessPanel().removeAll();
// .closeIntrospector(id.getName());
// }
// Switch view.
getIDE().getMainPanel().switchView();
for (TextEditorInterface editor : EditManager.getInstance().getEditors()) {
editor.setEditable(true);
}
EditManager.getInstance().setEditable(true);
break;
case ENVIRONMENT_PROCESS:
try {
new SwingWorker() {
@Override
protected Void doInBackground() throws Exception {
((EnvironmentPort) node.getUserObject()).shutDown();
return null;
}
}.execute();
} catch (Exception e) {
new Warning(Resources.get(WarningStrings.FAILED_KILL_ENV), e).emit();
}
break;
default: // FIXME redundant check, if we make NodeType more specific
throw new GOALBug(this + "should only be enabled while selection is a PROCESS node, but found" //$NON-NLS-1$
+ node);
}
// update view and switch to edit view if needed
if (getCurrentState().getViewMode() == IDEMainPanel.DEBUG_VIEW && getCurrentState().getRuntime() == null) {
getIDE().getMainPanel().switchView();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy