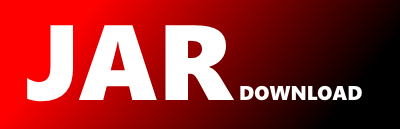
nl.tudelft.goal.SimpleIDE.actions.PauseAction Maven / Gradle / Ivy
/**
* GOAL interpreter that facilitates developing and executing GOAL multi-agent
* programs. Copyright (C) 2011 K.V. Hindriks, W. Pasman
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see .
*/
package nl.tudelft.goal.SimpleIDE.actions;
import java.awt.event.ActionEvent;
import java.util.List;
import javax.swing.tree.TreeNode;
import eis.iilang.EnvironmentState;
import goal.core.agent.Agent;
import goal.core.runtime.RuntimeManager;
import goal.core.runtime.service.environmentport.EnvironmentPort;
import goal.tools.IDEGOALInterpreter;
import goal.tools.debugger.SteppingDebugger.RunMode;
import goal.tools.errorhandling.Resources;
import goal.tools.errorhandling.Warning;
import goal.tools.errorhandling.WarningStrings;
import goal.tools.errorhandling.exceptions.GOALBug;
import goal.tools.errorhandling.exceptions.GOALException;
import nl.tudelft.goal.SimpleIDE.IDEMainPanel;
import nl.tudelft.goal.SimpleIDE.IDENode;
import nl.tudelft.goal.SimpleIDE.IDEState;
import nl.tudelft.goal.SimpleIDE.IconFactory;
import nl.tudelft.goal.SimpleIDE.NodeType;
import nl.tudelft.goal.SimpleIDE.ProcessNode;
/**
* Puts the selected MAS in pause mode.
*
* More specifically, based on the selection the following happens:
*
* - mas has been selected (or no process has been selected):
* ALL agents are put into pause mode.
* - agent process has been selected:
* the agent is put into pause mode (NOTE!!! this really is "STEPPING" mode, we
* are kind of abusing the debugger mode here and consequences are not
* immediately clear )
* - environment has been selected:
* pauses environment.
*
*
* @param node
* process node to be put into PAUSE mode.
* @author W.Pasman
* @modified W.Pasman 20jun2011 into action
*/
public class PauseAction extends GOALAction {
private static final long serialVersionUID = 1530252601201278907L;
public PauseAction() {
setIcon(IconFactory.PAUSE.getIcon());
}
@Override
public void eventOccured(IDEState currentState, Object evt) {
List extends IDENode> selection = currentState.getSelectedProcessNodes();
if (selection.isEmpty() || currentState.getViewMode() != IDEMainPanel.DEBUG_VIEW) {
setActionEnabled(false);
return;
}
IDENode node = selection.get(0);
NodeType nodeType = node.getType();
RunMode mode;
switch (nodeType) {
case MAS_PROCESS:
setDescription("Pause all agents"); //$NON-NLS-1$
mode = ((ProcessNode) node).getProcessRunMode();
setActionEnabled(mode == RunMode.RUNNING || mode == RunMode.STEPPING);
break;
case AGENT_PROCESS:
setDescription("Pause agent"); //$NON-NLS-1$
mode = ((ProcessNode) node).getProcessRunMode();
setActionEnabled(mode == RunMode.RUNNING || mode == RunMode.STEPPING);
break;
case ENVIRONMENT_PROCESS:
setDescription("Pause environment"); //$NON-NLS-1$
mode = ((ProcessNode) node).getProcessRunMode();
RuntimeManager, ?> runManager = currentState.getRuntime();
boolean connected = runManager != null && runManager.getEnvironmentPort() != null;
setActionEnabled(connected && mode != RunMode.KILLED);
break;
default:
setActionEnabled(false);
}
}
@Override
protected void execute(TreeNode selectedNode, ActionEvent ae) throws GOALException {
pause((ProcessNode) selectedNode);
}
@SuppressWarnings("unchecked")
private void pause(ProcessNode node) {
switch (node.getType()) {
case MAS_PROCESS:
// Pause all agents and environment that are part of the MAS.
for (int i = 0; i < node.getChildCount(); i++) {
ProcessNode childNode = (ProcessNode) node.getChildAt(i);
pause(childNode);
}
break;
case AGENT_PROCESS:
switch (node.getProcessRunMode()) {
case KILLED:
// ignore stepping of killed agents.
case STEPPING:
// ignore agents still busy with stepping.
case FINESTEPPING:
case QUERYING:
break;
case PAUSED:
case RUNNING:
Agent agent = (Agent) node.getUserObject();
agent.getController().getDebugger().finestep();
break;
case REMOTEPROCESS:
new Warning(Resources.get(WarningStrings.FAILED_PAUSE_REMOTEAGT)).emit();
break;
case UNKNOWN:
agent = (Agent) node.getUserObject();
if (agent.getController().getDebugger().getRunMode() != RunMode.KILLED) {
// Let's be brave and try to pause agent.
// We'll see what happens.
agent.getController().getDebugger().finestep();
}
break;
}
break;
case ENVIRONMENT_PROCESS:
if (((EnvironmentPort) node.getUserObject()).getEnvironmentState() == EnvironmentState.RUNNING) {
try {
((EnvironmentPort) node.getUserObject()).pause();
} catch (Exception e) {
new Warning(Resources.get(WarningStrings.FAILED_PAUSE), e).emit();
}
}
break;
default: // FIXME redundant check, if we make node types more accurate.
throw new GOALBug(this + " should only be enabled while selection is a PROCESS node, but found node of " //$NON-NLS-1$
+ node.getType() + " named " + node.getNodeName()); //$NON-NLS-1$
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy