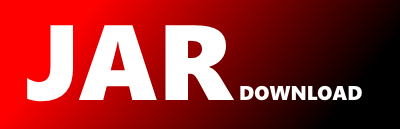
nl.tudelft.goal.SimpleIDE.prefgui.RuntimePrefPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simpleidemodules Show documentation
Show all versions of simpleidemodules Show documentation
An IDE for GOAL based on JEdit.
The newest version!
/**
* GOAL interpreter that facilitates developing and executing GOAL multi-agent
* programs. Copyright (C) 2011 K.V. Hindriks, W. Pasman
*
* This program is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License for more
* details.
*
* You should have received a copy of the GNU General Public License along with
* this program. If not, see .
*/
package nl.tudelft.goal.SimpleIDE.prefgui;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JCheckBox;
import javax.swing.JPanel;
import javax.swing.JPopupMenu;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import goal.preferences.CorePreferences;
import goal.preferences.Preferences;
import nl.tudelft.goal.SimpleIDE.preferences.IDEPreferences;
/**
* The panel is a GUI for the user to edit IDE preferences. We set the
* preferences through {@link goal.preferences.PMPreferences}.
*
* @author W.Pasman 24mar09
* @modified V.Koeman 12jun13 refactoring preferences: management separated from
* display through new class
* @modified K.Hindriks Layout and naming.
*/
public class RuntimePrefPanel extends JPanel implements ActionListener, ChangeListener {
private static final long serialVersionUID = 9138030922232947696L;
// Debugging.
private final JCheckBox openDebugTraceTabAtAgentLaunch = new JCheckBox(
"Open debug trace tab when agent is launched");
// High Performance section.
private final JCheckBox sleepRepeatingAgent = new JCheckBox("Sleep agents when they do no actions");
private final JCheckBox removeKilledAgent = new JCheckBox("Remove agents when they are killed");
// Environment section.
private final JCheckBox printEntities = new JCheckBox("print message when new entity appears");
private final JCheckBox agentCopyEnvRunState = new JCheckBox(
"new agents copy the environment run state (or run if no environment available)");
// Learning section.
// private final JCheckBox enablelearn = new JCheckBox("enable learning");
// private final JTextField agentsbrowsedir = new JTextField("/");
// private final JLabel agentsBrowseDirExplanation = new JLabel("Use this
// learned-behaviour file:");
// private final JButton agentBrowseButton = new JButton("Browse...");
/**
* Creates the Platform and Runtime tab in the GOAL Preferences panel.
*/
public RuntimePrefPanel() {
// Initialize settings of check boxes etc.
initSettings();
// Define layout.
setLayout(new GridLayout(0, 1));
// Debugging section.
add(PreferencesPanel.getBoldFontJLabel("Debugging"));
add(this.openDebugTraceTabAtAgentLaunch);
this.openDebugTraceTabAtAgentLaunch.addChangeListener(this);
// Performance section.
add(new JPopupMenu.Separator());
add(PreferencesPanel.getBoldFontJLabel("High Performance"));
add(this.sleepRepeatingAgent);
add(this.removeKilledAgent);
// Environment section.
add(new JPopupMenu.Separator());
add(PreferencesPanel.getBoldFontJLabel("Environment"));
add(this.printEntities);
add(this.agentCopyEnvRunState);
// Learning section.
// add(new JPopupMenu.Separator());
// add(PreferencesPanel.getBoldFontJLabel("Learning"));
// add(this.enablelearn);
// add(this.agentsBrowseDirExplanation);
// add(agentbrowsepanel);
// this.enablelearn.addActionListener(this);
this.sleepRepeatingAgent.addActionListener(this);
this.removeKilledAgent.addActionListener(this);
this.printEntities.addActionListener(this);
this.agentCopyEnvRunState.addActionListener(this);
}
/**
* Copies settings from preferences to the check boxes
*/
private void initSettings() {
this.openDebugTraceTabAtAgentLaunch.setSelected(IDEPreferences.getOpenDebugTraceTabAtAgentLaunch());
this.sleepRepeatingAgent.setSelected(CorePreferences.getSleepRepeatingAgent());
this.removeKilledAgent.setSelected(CorePreferences.getRemoveKilledAgent());
this.printEntities.setSelected(CorePreferences.getPrintEntities());
this.agentCopyEnvRunState.setSelected(CorePreferences.getAgentCopyEnvRunState());
// this.enablelearn.setSelected(CorePreferences.isLearning());
// this.agentsbrowsedir.setText(CorePreferences.getLearnFile());
}
@Override
public void actionPerformed(ActionEvent event) {
CorePreferences.setSleepRepeatingAgent(this.sleepRepeatingAgent.isSelected());
CorePreferences.setRemoveKilledAgent(this.removeKilledAgent.isSelected());
CorePreferences.setPrintEntities(this.printEntities.isSelected());
CorePreferences.setAgentCopyEnvRunState(this.agentCopyEnvRunState.isSelected());
// CorePreferences.setLearning(this.enablelearn.isSelected());
// CorePreferences.setLearnFile(this.agentsbrowsedir.getText());
Preferences.persistAllPrefs();
}
@Override
public void stateChanged(ChangeEvent e) {
IDEPreferences.setOpenDebugTraceTabAtAgentLaunch(this.openDebugTraceTabAtAgentLaunch.isSelected());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy