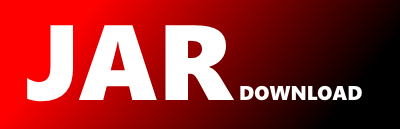
junit.extensions.jfcunit.eventdata.AbstractKeyEventData Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.eventdata;
import java.awt.AWTEvent;
import java.awt.Component;
import java.awt.Toolkit;
import java.awt.event.InputEvent;
import java.awt.event.KeyEvent;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import junit.extensions.jfcunit.JFCUnit;
import junit.extensions.jfcunit.TestHelper;
import junit.extensions.jfcunit.keyboard.JFCKeyStroke;
import junit.extensions.jfcunit.keyboard.KeyMapping;
/**
* Abstract data container class that holds most of the data necessary for
* jfcUnit to fire key events.
*
* @author Vijay Aravamudhan :
* ThoughtWorks Inc.
*/
public abstract class AbstractKeyEventData extends AbstractEventData
{
/**
* Key Strokes to be simulated.
*/
private final List m_keyStrokes = new ArrayList();
/**
* Get the key strokes for this event.
*
* @return JFCKeyStroke[] to be simulated by this event.
*/
public final JFCKeyStroke[] getKeyStrokes()
{
return (JFCKeyStroke[]) this.m_keyStrokes.toArray(new JFCKeyStroke[0]);
}
/**
* Set the modifiers on the event.
*
* @param modifiers
* modifiers to be set.
*/
@Override
public final void setModifiers(final int modifiers)
{
super.setModifiers(modifiers);
setupKeyStrokes();
}
/**
* Get the default modifiers.
*
* @return Default key modiers.
*/
@Override
public int getDefaultModifiers()
{
return EventDataConstants.DEFAULT_KEY_MODIFIERS;
}
/**
* Set the attribute value.
*
* @param km
* The new KeyMapping
*/
public static void setKeyMapping(final KeyMapping km)
{
TestHelper.setKeyMapping(km);
}
/**
* Get the attribute value.
*
* @return KeyMapping The KeyMapping that is being currently used.
*/
public static KeyMapping getKeyMapping()
{
return TestHelper.getKeyMapping();
}
/**
* Get the current modifier text.
*
* @return String containing the text form of the modifiers.
*/
@Override
public final String getModifierText()
{
final StringBuffer buf = new StringBuffer();
final int modifiers = getModifiers();
if ((modifiers & InputEvent.ALT_MASK) != 0)
{
buf.append(Toolkit.getProperty("AWT.alt", "Alt"));
buf.append("+");
}
if ((modifiers & InputEvent.META_MASK) != 0)
{
buf.append(Toolkit.getProperty("AWT.meta", "Meta"));
buf.append("+");
}
if ((modifiers & InputEvent.CTRL_MASK) != 0)
{
buf.append(Toolkit.getProperty("AWT.control", "Ctrl"));
buf.append("+");
}
if ((modifiers & InputEvent.SHIFT_MASK) != 0)
{
buf.append(Toolkit.getProperty("AWT.shift", "Shift"));
buf.append("+");
}
if ((modifiers & InputEvent.ALT_GRAPH_MASK) != 0)
{
buf.append(Toolkit.getProperty("AWT.altGraph", "Alt Graph"));
buf.append("+");
}
if (buf.length() > 0)
{
buf.setLength(buf.length() - 1); // remove trailing '+'
}
return buf.toString();
}
/**
* Check if this event can consume the {@link java.awt.AWTEvent}.
*
* @param ae
* {@link java.awt.AWTEvent} to be consumed.
* @return boolean true if the event can be consumed.
*/
@Override
public boolean canConsume(final AWTEvent ae)
{
if (!isValid())
{
return true;
}
return ae instanceof KeyEvent && getRoot((Component) ae.getSource()).equals(getRoot());
}
/**
* Get the attribute value.
*
* @param ae
* {@link java.awt.AWTEvent} to be processed.
* @return boolean The value of the attribute
*/
@Override
public boolean consume(final AWTEvent ae)
{
if (!(ae instanceof KeyEvent))
{
return false;
}
final KeyEvent ke = (KeyEvent) ae;
final int id = ke.getID();
if (id == KeyEvent.KEY_TYPED || id == KeyEvent.KEY_RELEASED || isMetaChar(ke.getKeyCode())
|| ae.getSource().equals(getRoot()))
{
// Ignore the event.
return true;
}
return false;
}
/**
* Compare to event datas and deterime if they are equal.
*
* @param o
* Object to be compared.
* @return true if the events are the same.
*/
@Override
public boolean equals(final Object o)
{
if (!super.equals(o))
{
return false;
}
// isValid
final AbstractKeyEventData data = (AbstractKeyEventData) o;
final JFCKeyStroke[] dataStrokes = data.getKeyStrokes();
final JFCKeyStroke[] thisStrokes = getKeyStrokes();
return Arrays.equals(dataStrokes, thisStrokes);
}
/**
* Return the hashCode of the object.
*
* @return hashCode of the super class.
*/
@Override
public int hashCode()
{
return super.hashCode();
}
/**
* Prepare the text component.
*
* @return boolean true if the component is ready.
*/
@Override
public boolean prepareComponent()
{
if (super.prepareComponent())
{
if (!getComponent().hasFocus())
{
getComponent().requestFocus();
JFCUnit.flushAWT();
}
return true;
} else
{
return false;
}
}
/**
* Each implementing class needs to provide a method to translate the string
* into the keystorkes required to produce the string.
*/
protected abstract void setupKeyStrokes();
/**
* Add key strokes to this event.
*
* @param strokes
* Key strokes to be added.
*/
protected final void addKeyStrokes(final JFCKeyStroke[] strokes)
{
for (int i = 0; i < strokes.length; i++)
{
this.m_keyStrokes.add(strokes[i]);
}
}
/**
* Apply the modifiers specified to all of the key strokes.
*
* @param modifiers
* Modifiers to be applied.
*/
protected final void applyModifier(final int modifiers)
{
for (int i = 0; i < this.m_keyStrokes.size(); i++)
{
final JFCKeyStroke s = (JFCKeyStroke) this.m_keyStrokes.get(i);
final int mods = s.getModifiers() | modifiers;
s.setModifiers(mods);
}
}
/**
* Clear key Strokes.
*/
protected final void clearKeyStrokes()
{
this.m_keyStrokes.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy