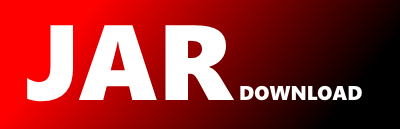
junit.extensions.jfcunit.eventdata.DragEventDataEx Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.eventdata;
import java.awt.AWTEvent;
import java.awt.Component;
import java.awt.Point;
import java.awt.event.MouseEvent;
import java.util.ArrayList;
import java.util.List;
import org.w3c.dom.Element;
/**
* DragEventSource is a wrapper for Drag Events. The event encapsulates the
* source and destination locations for the drag with xxxMouseEventData types.
*
* @author Vijay Aravamudhan :
* ThoughtWorks Inc.
*/
public class DragEventDataEx extends AbstractEventData
{
public static final class Builder
{
private AbstractMouseEventData source;
private AbstractMouseEventData dest;
private long delay = DEFAULT_SLEEPTIME;
public Builder()
{
// no impl
}
public Builder withDelay(final long delay)
{
this.delay = delay;
return this;
}
public Builder withDest(final AbstractMouseEventData dest)
{
this.dest = dest;
return this;
}
public Builder withSource(final AbstractMouseEventData source)
{
this.source = source;
return this;
}
public DragEventDataEx build()
{
return new DragEventDataEx(this.source, this.dest, this.delay);
}
}
/**
* Destination of the drag event.
*/
private AbstractMouseEventData m_dest;
/**
* Source of the drag event.
*/
private AbstractMouseEventData m_source;
/**
* List of points in the drag path.
*/
private final List m_points = new ArrayList();
/**
* Original reference location of component.
*/
private Point m_origPoint = null;
/**
* Constructor for a drag event.
*
* @param testCase
* TestCase.
* @param source
* {@link AbstractMouseEventData} indicating the starting
* location of the drag event.
* @param dest
* {@link AbstractMouseEventData} indicating the ending location
* of the drag event.
* @param delay
* sleepTime of the event.
*/
protected DragEventDataEx(final AbstractMouseEventData source, final AbstractMouseEventData dest, final long delay)
{
setSource(source);
setDest(dest);
setSleepTime(delay);
if (getSource() != null && getSource().isValid())
{
setValid(true);
}
}
/**
* This method is provided here to close the abstract base class.
*
* @return null This method always returns null.
*/
@Override
public final Component getComponent()
{
return null;
}
/**
* Get the default modifiers.
*
* @return default modifiers for the drag event.
*/
@Override
public final int getDefaultModifiers()
{
return this.m_source.getDefaultModifiers();
}
/**
* Set the Destination of the drag event.
*
* @param dest
* destination {@link AbstractMouseEventData}.
*/
public final void setDest(final AbstractMouseEventData dest)
{
this.m_dest = dest;
}
/**
* Get the destination MouseEventData.
*
* @return The destination {@link AbstractMouseEventData}.
*/
public final AbstractMouseEventData getDest()
{
return this.m_dest;
}
/**
* Get the modifier text for the current.
*
* @return String modifier text.
*/
@Override
public final String getModifierText()
{
return this.m_source.getModifierText();
}
/**
* Set the points in the drag path.
*
* @param points
* Set of points to be hit in the drag path.
*/
public final void setPoints(final Point[] points)
{
this.m_points.clear();
for (int i = 0; i < points.length; i++)
{
this.m_points.add(points[i]);
}
}
/**
* Get the list of points in the drag path.
*
* @return {@link java.awt.Point}[] list of points.
*/
public final Point[] getPoints()
{
return this.m_points.toArray(new Point[0]);
}
/**
* Set the Source of the drag event.
*
* @param source
* {@link AbstractMouseEventData} indicating the starting
* location of the drag event.
*/
public final void setSource(final AbstractMouseEventData source)
{
this.m_source = source;
if (this.m_source != null && this.m_source.getComponent() != null && this.m_source.getComponent().isVisible())
{
this.m_origPoint = this.m_source.getLocationOnScreen();
}
}
/**
* Get the Source of the drag event.
*
* @return The source {@link AbstractMouseEventData}.
*/
public final AbstractMouseEventData getSource()
{
return this.m_source;
}
/**
* Add a {@link java.awt.Point} to the drag path.
*
* @param p
* {@link java.awt.Point} to be hit along the drag path.
*/
public final void addPoint(final Point p)
{
this.m_points.add(p);
}
/**
* This prepares the source and dest components if set, and inserts/appends
* the screen locations onto the list of points.
*
* @return boolean true if the source and destination events were able to
* "prepare" their components.
*/
@Override
public final boolean prepareComponent()
{
boolean results = true;
boolean resultd = true;
if (this.m_source != null)
{
results = this.m_source.prepareComponent();
this.m_points.add(0, this.m_source.getLocationOnScreen());
}
final Point compLocation = this.m_source.getComponent().getLocationOnScreen();
if (this.m_points.size() > 1)
{
for (int i = 1; i < this.m_points.size(); i++)
{
final Point p = this.m_points.get(i);
p.translate(compLocation.x, compLocation.y);
}
}
if (this.m_dest != null)
{
resultd = this.m_dest.prepareComponent();
this.m_points.add(this.m_dest.getLocationOnScreen());
}
return results & resultd;
}
/**
* Check if this event can consume the {@link java.awt.AWTEvent}.
*
* @param ae
* {@link java.awt.AWTEvent} to be consumed.
* @return boolean true if the event can be consumed.
*/
@Override
public boolean canConsume(final AWTEvent ae)
{
if (!(ae instanceof MouseEvent))
{
return false;
}
final MouseEvent me = (MouseEvent) ae;
if (me.getID() == MouseEvent.MOUSE_DRAGGED || me.getID() == MouseEvent.MOUSE_EXITED
|| me.getID() == MouseEvent.MOUSE_ENTERED)
{
return true;
}
if (isValid())
{
return getSource().canConsume(ae);
}
return false;
}
/**
* Consume the {@link java.awt.AWTEvent}.
*
* @param ae
* Event to be consumed.
* @return boolean true if the event was consumed.
*/
@Override
public boolean consume(final AWTEvent ae)
{
if (!(ae instanceof MouseEvent))
{
return false;
}
final MouseEvent me = (MouseEvent) ae;
if (me.getID() == MouseEvent.MOUSE_ENTERED || me.getID() == MouseEvent.MOUSE_EXITED)
{
return true;
}
if (me.getID() == MouseEvent.MOUSE_DRAGGED)
{
final Point p = convertToSource(me.getComponent(), me.getPoint());
addPoint(p);
} else
{
return getSource().consume(ae);
}
return true;
}
/**
* Equals comparison.
*
* @param o
* Object to be compared.
* @return true if the objects are the same.
*/
@Override
public boolean equals(final Object o)
{
if (!(o instanceof DragEventDataEx))
{
return false;
}
final DragEventDataEx ded = (DragEventDataEx) o;
if (this.m_source == null)
{
if (ded.getSource() != null)
{
return false;
}
} else
{
if (!this.m_source.equals(ded.getSource()))
{
return false;
}
}
if (this.m_dest == null)
{
if (ded.getDest() != null)
{
return false;
}
} else
{
if (!this.m_dest.equals(ded.getDest()))
{
return false;
}
}
return true;
}
/**
* Calculate a hashcode based upon the source and the dest of this class.
*
* @return int hashcode.
*/
@Override
public int hashCode()
{
int hc = super.hashCode();
if (this.m_source != null)
{
hc += this.m_source.hashCode();
}
if (this.m_dest != null)
{
hc += this.m_dest.hashCode();
}
return hc;
}
/**
* Populate the element wiht the data.
*
* @param e
* element to be populated.
*/
@Override
public void populate(final Element e)
{
}
/**
* Return a string representing the eventdata.
*
* @return String description of the event data.
*/
@Override
public String toString()
{
final StringBuffer buf = new StringBuffer(1000);
buf.append("DragEventData:");
if (!isValid())
{
buf.append(" invalid");
return buf.toString();
}
buf.append("(Source:" + getSource().toString() + ")");
buf.append("Points:");
for (int i = 0; i < this.m_points.size(); i++)
{
if (i != 0)
{
buf.append(",");
}
buf.append(this.m_points.get(i));
}
return buf.toString();
}
/**
* Convert the point to the source coordinate system.
*
* @param comp
* Souce component.
* @param p
* Point to be converted.
* @return point of p related to source coordinates.
*/
private Point convertToSource(final Component comp, final Point p)
{
final Point screen = comp.getLocationOnScreen();
screen.translate(p.x, p.y);
if (this.m_origPoint == null)
{
return p;
}
final int dx = screen.x - this.m_origPoint.x;
final int dy = screen.y - this.m_origPoint.y;
final Point delta = new Point(p);
delta.translate(dx, dy);
return delta;
}
public static DragEventDataEx create(final AbstractMouseEventData source, final AbstractMouseEventData dest)
{
return new Builder().withSource(source).withDest(dest).build();
}
public static DragEventDataEx create(final AbstractMouseEventData source)
{
return new Builder().withSource(source).build();
}
public static DragEventDataEx create(final AbstractMouseEventData source, final AbstractMouseEventData dest,
final int i)
{
return new Builder().withSource(source).withDest(dest).withDelay(i).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy