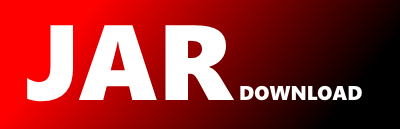
junit.extensions.jfcunit.eventdata.EventDataConstants Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.eventdata;
import java.awt.event.MouseEvent;
import javax.swing.SwingConstants;
/**
* An interface defining the default values of the common attributes used in all EventDataContainer classes.
* Package level access is enough for this class.
*
* @author Vijay Aravamudhan : ThoughtWorks Inc.
*/
public interface EventDataConstants {
/**
* String description of the positions.
*/
String[] POSITIONSTRINGS = {
"center", "north", "northeast", "east", "southeast", "south",
"southwest", "west", "northwest", "", "", "", "custom", "percent",
"offset"
};
/**
* Default value specifying whether the {@link MouseEvent} being fired
* would trigger a popup or not.
*/
boolean DEFAULT_ISPOPUPTRIGGER = false;
/**
* Identifier for the mouse position at the center of the {@link java.awt.Component}.
*/
int CENTER = SwingConstants.CENTER;
/**
* Identifier for the mouse position at a point in the component specified by the user.
* Skip other SwingConstants in case they are needed in future.
*/
int CUSTOM = 12;
/**
* Default value specifying the modifiers key values
* that need to be passed by {@link java.awt.event.KeyEvent}s.
*/
int DEFAULT_KEY_MODIFIERS = 0;
/**
* Default value specifying the modifier key values that need to be passed onto the {@link MouseEvent}.
*/
int DEFAULT_MOUSE_MODIFIERS = MouseEvent.BUTTON1_MASK;
/**
* Default value specifying the number of clicks for the {@link MouseEvent}s.
*/
int DEFAULT_NUMBEROFCLICKS = 1;
/**
* Default value specifying the modifier key values that need to be passed onto the {@link MouseEvent}.
*/
int DEFAULT_POPUP_MODIFIERS = MouseEvent.BUTTON3_MASK;
/**
* Default value specifying the position of the mouse relative to the {@link java.awt.Component}.
*/
int DEFAULT_POSITION = CENTER;
/* ---------------- Wheel support ------------------------*/
/**
* The amount to scroll by default.
*/
int DEFAULT_SCROLL_AMOUNT = 3;
/**
* The amount to scroll by default.
*/
int DEFAULT_WHEEL_ROTATION = 1;
/**
* Identifier used to refer to the down-arrow sub-component
* (used only for {@link javax.swing.JSpinner}).
*/
int DOWN_ARROW_SUBCOMPONENT = 2;
/**
* Identifier for the mouse position at the center of the right edge of the {@link java.awt.Component}.
*/
int EAST = SwingConstants.EAST;
/**
* Identifier used to refer to the editor sub-component
* (used only for {@link javax.swing.JSpinner}).
*/
int EDITOR_SUBCOMPONENT = 3;
/* ---------------- JSpinner support ------------------------*/
/**
* Identifier used to refer to an invalid sub-component
* (used only for {@link javax.swing.JSpinner}).
*/
int INVALID_SUBCOMPONENT = -1;
/**
* Invalid text offset.
*/
int INVALID_TEXT_OFFSET = -1;
/**
* Identifier for the mouse position at the center of the top edge {@link java.awt.Component}.
*/
int NORTH = SwingConstants.NORTH;
/**
* Identifier for the mouse position at the top right corner of the {@link java.awt.Component}.
*/
int NORTH_EAST = SwingConstants.NORTH_EAST;
/**
* Identifier for the mouse position at the top left corner of the {@link java.awt.Component}.
*/
int NORTH_WEST = SwingConstants.NORTH_WEST;
/**
* Identifier for the mouse position at a point specified by the offset.
*/
int OFFSET = 14;
/**
* Identifier for the mouse position at a point in the component specified by
* percentage of width and height. The point argument should
* contain the percentages. For example: to get a position
* in the center the arguments to the event for position and point
* would be PERCENT, new {@link java.awt.Point}(50, 50)
.
*/
int PERCENT = 13;
/**
* Identifier for the mouse position at the center of the bottom edge of the {@link java.awt.Component}.
*/
int SOUTH = SwingConstants.SOUTH;
/**
* Identifier for the mouse position at the bottom right corner of the {@link java.awt.Component}.
*/
int SOUTH_EAST = SwingConstants.SOUTH_EAST;
/**
* Identifier for the mouse position at the bottom left corner of the {@link java.awt.Component}.
*/
int SOUTH_WEST = SwingConstants.SOUTH_WEST;
/**
* Identifier used to refer to the up-arrow sub-component
* (used only for {@link javax.swing.JSpinner}).
*/
int UP_ARROW_SUBCOMPONENT = 1;
/**
* Identifier for the mouse position at the center of the left edge of the {@link java.awt.Component}.
*/
int WEST = SwingConstants.WEST;
/**
* Default value specifying the hold time in ms before ejecting a event
* from the event manager.
*/
long DEFAULT_HOLDTIME = 100L;
/**
* Default value specifying the wait time in ms between each event.
*/
long DEFAULT_SLEEPTIME = 300L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy