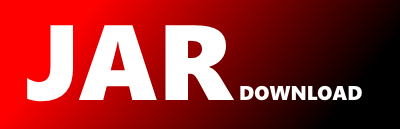
junit.extensions.jfcunit.eventdata.JTextComponentMouseEventDataEx Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.eventdata;
import java.awt.AWTEvent;
import java.awt.Component;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.event.MouseEvent;
import javax.swing.text.BadLocationException;
import javax.swing.text.JTextComponent;
import junit.extensions.jfcunit.JFCUnit;
import junit.extensions.xml.XMLConstants;
/**
* Data container class that holds all the data necessary for jfcUnit to fire
* mouse events.
*
* @author Vijay Aravamudhan :
* ThoughtWorks Inc.
*/
public class JTextComponentMouseEventDataEx extends AbstractMouseEventData
{
/**
* The Component on which to trigger the event.
*/
private JTextComponent m_comp;
/**
* Offset into the text component in characters.
*/
private int m_offset = INVALID_TEXT_OFFSET;
/**
* Constructor.
*/
public JTextComponentMouseEventDataEx()
{
super();
setValid(false);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp)
{
this(comp, DEFAULT_NUMBEROFCLICKS);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks)
{
this(comp, numberOfClicks, DEFAULT_MOUSE_MODIFIERS);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param sleepTime
* The wait time in ms between each event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final long sleepTime)
{
this(comp, DEFAULT_NUMBEROFCLICKS);
setSleepTime(sleepTime);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers)
{
this(comp, numberOfClicks, modifiers, DEFAULT_ISPOPUPTRIGGER);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks,
final boolean isPopupTrigger)
{
this(comp, numberOfClicks, getDefaultModifiers(isPopupTrigger), isPopupTrigger);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param sleepTime
* The wait time in ms between each event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final long sleepTime)
{
this(comp, numberOfClicks, DEFAULT_MOUSE_MODIFIERS, DEFAULT_ISPOPUPTRIGGER, sleepTime);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger)
{
this(comp, numberOfClicks, modifiers, isPopupTrigger, DEFAULT_SLEEPTIME);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks,
final boolean isPopupTrigger, final long sleepTime)
{
this(comp, numberOfClicks, getDefaultModifiers(isPopupTrigger), isPopupTrigger, sleepTime);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger, final long sleepTime)
{
this(comp, numberOfClicks, modifiers, isPopupTrigger, sleepTime, DEFAULT_POSITION, null, INVALID_TEXT_OFFSET);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param position
* The relative mouse position within the cell.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger, final long sleepTime, final int position)
{
this(comp, numberOfClicks, modifiers, isPopupTrigger, sleepTime, position, null, INVALID_TEXT_OFFSET);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param position
* This parameter will be ignored. It will be set to OFFSET.
* @param offset
* The offset into the text component.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger, final long sleepTime, final int position, final int offset)
{
this(comp, numberOfClicks, modifiers, isPopupTrigger, sleepTime, OFFSET, null, offset);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param referencePoint
* The CUSTOM mouse position within the cell.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger, final long sleepTime, final Point referencePoint)
{
this(comp, numberOfClicks, modifiers, isPopupTrigger, sleepTime, CUSTOM, referencePoint, INVALID_TEXT_OFFSET);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param position
* The relative mouse position within the cell.
* @param referencePoint
* If position is CUSTOM then the point is a offset from the
* location of the component. If the position is PERCENT then the
* location is a percentage offset of the hight and width.
* Otherwise, the referencePoint is unused.
* @param offset
* The character offset into the text component.
*/
public JTextComponentMouseEventDataEx(final JTextComponent comp, final int numberOfClicks, final int modifiers,
final boolean isPopupTrigger, final long sleepTime, final int position, final Point referencePoint,
final int offset)
{
setSource(comp);
setNumberOfClicks(numberOfClicks);
setModifiers(modifiers);
setPopupTrigger(isPopupTrigger);
setSleepTime(sleepTime);
setPosition(position);
setReferencePoint(referencePoint);
setOffset(offset);
setValid(true);
}
/**
* Set the offset.
*
* @param offset
* The new value of the offset
*/
public final void setOffset(final int offset)
{
this.m_offset = offset;
}
/**
* Get the offset.
*
* @return int The value of the offset
*/
public final int getOffset()
{
return this.m_offset;
}
/**
* Set the attribute value.
*
* @param comp
* The new value of the attribute
*/
public final void setSource(final JTextComponent comp)
{
this.m_comp = comp;
}
/**
* Get the attribute value.
*
* @return Component The value of the attribute
*/
public final JTextComponent getSource()
{
return this.m_comp;
}
/**
* The component on which the event has to be fired.
*
* @return The component
*/
@Override
public Component getComponent()
{
// by default, the component is the same as the source
return getSource();
}
/**
* Check if this event can consume the event given.
*
* @param ae
* AWTEvent to be consumed.
* @return true if the event may be consumed.
*/
@Override
public boolean canConsume(final AWTEvent ae)
{
if (ae.getSource() instanceof JTextComponent && super.canConsume(ae) && sameSource(ae))
{
return true;
}
return false;
}
/**
* Consume the event.
*
* @param ae
* AWTEvent to be consumed.
* @return boolean true if the event was consumed.
*/
@Override
public boolean consume(final AWTEvent ae)
{
if (super.consume(ae))
{
return true;
}
final MouseEvent me = (MouseEvent) ae;
final JTextComponent source = (JTextComponent) me.getSource();
setSource(source);
setModifiers(me.getModifiers());
setNumberOfClicks(me.getClickCount());
setPopupTrigger(me.isPopupTrigger());
final Point p = new Point(me.getX(), me.getY());
final Point screen = source.getLocationOnScreen();
screen.translate(p.x, p.y);
setLocationOnScreen(screen);
final int offset = source.viewToModel(p);
setOffset(offset);
setPosition(CENTER);
setReferencePoint(null);
setSleepTime(getDefaultSleepTime());
setValid(true);
return true;
}
/**
* Compare to event datas and deterime if they are equal.
*
* @param o
* Object to be compared.
* @return true if the events are the same.
*/
@Override
public boolean equals(final Object o)
{
if (!super.equals(o))
{
return false;
}
final JTextComponentMouseEventDataEx data = (JTextComponentMouseEventDataEx) o;
return data.getOffset() == getOffset();
}
/**
* Get the hashCode.
*
* @return int hashCode.
*/
@Override
public int hashCode()
{
return super.hashCode() + this.m_offset;
}
/**
* Populate the XML Element with this objects attributes.
*
* @param e
* Element to be populated.
*/
@Override
public void populate(final org.w3c.dom.Element e)
{
super.populate(e);
e.setAttribute(XMLConstants.TYPE, "JTextComponentMouseEventData");
e.setAttribute(XMLConstants.INDEX, "" + getOffset());
}
/**
* Prepare the component to receive the event.
*
* @return true if the component is ready to receive the event.
*/
@Override
public boolean prepareComponent()
{
if (!isValidForProcessing(getSource()))
{
return false;
}
Point p = null;
JFCUnit.flushAWT();
JFCUnit.pauseAWT();
if (getPosition() == OFFSET)
{
setPosition(EAST);
if (this.m_offset == -1 || this.m_offset > this.m_comp.getDocument().getLength())
{
this.m_offset = this.m_comp.getDocument().getLength();
}
Rectangle rect = null;
try
{
rect = this.m_comp.modelToView(this.m_offset);
} catch (final BadLocationException ex)
{
throw new RuntimeException("Invalid Offset:" + this.m_offset + ":" + ex);
}
p = calculatePoint(rect);
} else
{
p = calculatePoint(this.m_comp.getBounds());
}
final Point screen = this.m_comp.getLocationOnScreen();
screen.translate(p.x, p.y);
setLocationOnScreen(screen);
return true;
}
/**
* Get string description of event.
*
* @return String description of event.
*/
@Override
public String toString()
{
if (!isValid())
{
return super.toString();
}
final StringBuffer buf = new StringBuffer(1000);
buf.append(super.toString());
buf.append(" offset: " + getOffset());
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy