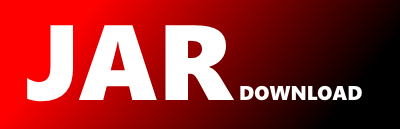
junit.extensions.jfcunit.eventdata.MouseWheelEventDataEx Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.eventdata;
import java.awt.AWTEvent;
import java.awt.Component;
import java.awt.Container;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.event.MouseWheelEvent;
import javax.swing.JViewport;
import junit.extensions.jfcunit.JFCUnit;
/**
* Data container class that holds all the data necessary for jfcUnit to fire
* mouse events.
*
* @author Vijay Aravamudhan :
* ThoughtWorks Inc.
*/
public class MouseWheelEventDataEx extends MouseEventDataEx
{
/**
* The amount to scroll by default.
*/
public static final int DEFAULT_MOUSE_CLICKS = 0;
/**
* The Component on which to trigger the event.
*/
private Component m_comp;
/**
* Amount to scroll for each wheel rotation.
*/
private int m_scrollAmount = 0;
/**
* Number of wheel clicks to rotate.
*/
private int m_wheelRotation = 0;
/**
* Default Constructor.
*/
public MouseWheelEventDataEx()
{
super();
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
*/
public MouseWheelEventDataEx(final Component comp)
{
this(comp, DEFAULT_SCROLL_AMOUNT, DEFAULT_WHEEL_ROTATION);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
*/
public MouseWheelEventDataEx(final Component comp, final int wheelRotation)
{
this(comp, DEFAULT_SCROLL_AMOUNT, wheelRotation, DEFAULT_MOUSE_CLICKS);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation)
{
this(comp, amount, wheelRotation, DEFAULT_MOUSE_CLICKS);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation, final int numberOfClicks)
{
this(comp, amount, wheelRotation, numberOfClicks, DEFAULT_MOUSE_MODIFIERS);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers)
{
this(comp, amount, wheelRotation, numberOfClicks, modifiers, DEFAULT_ISPOPUPTRIGGER);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final boolean isPopupTrigger)
{
this(comp, amount, wheelRotation, numberOfClicks, getDefaultModifiers(isPopupTrigger), isPopupTrigger);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param sleepTime
* The wait time in ms between each event.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final long sleepTime)
{
this(comp, amount, wheelRotation, numberOfClicks, DEFAULT_MOUSE_MODIFIERS, DEFAULT_ISPOPUPTRIGGER, sleepTime);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers, final boolean isPopupTrigger)
{
this(comp, amount, wheelRotation, numberOfClicks, modifiers, isPopupTrigger, DEFAULT_SLEEPTIME);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final boolean isPopupTrigger, final long sleepTime)
{
this(comp, amount, wheelRotation, numberOfClicks, getDefaultModifiers(isPopupTrigger), isPopupTrigger,
sleepTime);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers, final boolean isPopupTrigger, final long sleepTime)
{
this(comp, amount, wheelRotation, numberOfClicks, modifiers, isPopupTrigger, sleepTime, DEFAULT_POSITION, null);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param position
* The relative mouse position within the cell.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers, final boolean isPopupTrigger, final long sleepTime,
final int position)
{
this(comp, amount, wheelRotation, numberOfClicks, modifiers, isPopupTrigger, sleepTime, position, null);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param referencePoint
* The CUSTOM mouse position within the cell.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers, final boolean isPopupTrigger, final long sleepTime,
final Point referencePoint)
{
this(comp, amount, wheelRotation, numberOfClicks, modifiers, isPopupTrigger, sleepTime, CUSTOM, referencePoint);
}
/**
* Constructor.
*
* @param testCase
* The Object on whose thread awtSleep()
has to be
* invoked.
* @param comp
* The component on which to trigger the event.
* @param amount
* The amount to scroll for each rotation.
* @param wheelRotation
* The amount to rotate the wheel positive for values towards the
* user. Negative for away from the user.
* @param numberOfClicks
* Number of clicks in the MouseEvent (1 for single-click, 2 for
* double clicks)
* @param modifiers
* The modifier key values that need to be passed onto the event.
* @param isPopupTrigger
* boolean specifying whether this event will show a popup.
* @param sleepTime
* The wait time in ms between each event.
* @param position
* The relative mouse position within the cell.
* @param referencePoint
* If position is CUSTOM then the point is a offset from the
* location of the component. If the position is PERCENT then the
* location is a percentage offset of the hight and width.
* Otherwise, the referencePoint is unused.
*/
public MouseWheelEventDataEx(final Component comp, final int amount, final int wheelRotation,
final int numberOfClicks, final int modifiers, final boolean isPopupTrigger, final long sleepTime,
final int position, final Point referencePoint)
{
setSource(comp);
setScrollAmount(amount);
setWheelRotation(wheelRotation);
setNumberOfClicks(numberOfClicks);
setModifiers(modifiers);
setPopupTrigger(isPopupTrigger);
setSleepTime(sleepTime);
setPosition(position);
setReferencePoint(referencePoint);
setValid(true);
}
/**
* Set the attribute value.
*
* @param comp
* The new value of the attribute.
*/
@Override
public final void setSource(final Component comp)
{
this.m_comp = comp;
}
/**
* Get the attribute value.
*
* @return Component The value of the attribute.
*/
@Override
public final Component getSource()
{
return this.m_comp;
}
/**
* The component on which the event has to be fired.
*
* @return The component.
*/
@Override
public Component getComponent()
{
// by default, the component is the same as the source
return getSource();
}
/**
* Set the scroll amount.
*
* @param scrollAmount
* Amount to scroll for each wheel click.
*/
public final void setScrollAmount(final int scrollAmount)
{
this.m_scrollAmount = scrollAmount;
}
/**
* Get the scroll amount.
*
* @return int scroll amount.
*/
public final int getScrollAmount()
{
return this.m_scrollAmount;
}
/**
* Set the wheel rotation.
*
* @param wheelRotation
* amount to rotate the wheel.
*/
public final void setWheelRotation(final int wheelRotation)
{
this.m_wheelRotation = wheelRotation;
}
/**
* Get the wheel rotation.
*
* @return int number of wheel rotations.
*/
public final int getWheelRotation()
{
return this.m_wheelRotation;
}
/**
* Returns true if the event can be consumed by this instnace of event data.
*
* @param ae
* Event to be consumed.
* @return true if the event was consumed.
*/
@Override
public boolean canConsume(final AWTEvent ae)
{
// Cannot consume if the event ID cannot be accessed
// or the event is not a mouse wheel event.
if (!(ae instanceof MouseWheelEvent))
{
return false;
}
final MouseWheelEvent mouseWheelEvent = (MouseWheelEvent) ae;
if (isValid())
{
final int modifiers = mouseWheelEvent.getModifiers();
if (ae.getSource() != getSource())
{
return false;
}
if (modifiers != getModifiers())
{
return false;
}
try
{
final int wheelRotation = mouseWheelEvent.getWheelRotation();
// Change of direction if result is negative.
if (wheelRotation * getWheelRotation() < 0)
{
return false;
}
} catch (final Exception e)
{
e.printStackTrace();
}
}
return true;
}
/**
* Consume the event.
*
* @param ae
* AWTEvent to be consumed.
* @return boolean true if the event was consumed.
*/
@Override
public boolean consume(final AWTEvent ae)
{
final MouseWheelEvent me = (MouseWheelEvent) ae;
try
{
final int amount = me.getScrollAmount();
setScrollAmount(amount);
final int wheelRotation = me.getWheelRotation();
setWheelRotation(wheelRotation + getWheelRotation());
} catch (final Exception e)
{
e.printStackTrace();
}
// If the event is valid then we do not need to
// reprocess the rest of the event.
if (isValid())
{
return true;
}
final Component source = (Component) me.getSource();
setSource(source);
setModifiers(me.getModifiers());
setNumberOfClicks(me.getClickCount());
setPopupTrigger(me.isPopupTrigger());
final Point p = new Point(me.getX(), me.getY());
setSleepTime(getDefaultSleepTime());
final Point screen = source.getLocationOnScreen();
screen.translate(p.x, p.y);
setLocationOnScreen(screen);
setPosition(CENTER);
setReferencePoint(null);
setValid(true);
return true;
}
/**
* Compare to event data and deterime if they are equal.
*
* @param o
* Object to be compared.
* @return true if the events are the same.
*/
@Override
public boolean equals(final Object o)
{
if (!super.equals(o))
{
return false;
}
final MouseWheelEventDataEx data = (MouseWheelEventDataEx) o;
return data.getWheelRotation() == getWheelRotation() && data.getScrollAmount() == getScrollAmount();
}
/**
* Get the hashCode for this object.
*
* @return int hashCode.
*/
@Override
public int hashCode()
{
return super.hashCode();
}
/**
* Prepare the component for firing the mouse event.
*
* @return boolean true if the component is ready.
*/
@Override
public boolean prepareComponent()
{
if (!isValidForProcessing(getSource()))
{
return false;
}
// Locate the ViewPort
Container parent = null;
if (getSource() instanceof Container)
{
parent = (Container) getSource();
} else
{
parent = getSource().getParent();
}
while (parent.getParent() != null && !(parent instanceof JViewport))
{
parent = parent.getParent();
}
if (!(parent instanceof JViewport))
{
return false;
}
JFCUnit.awtSleep(getSleepTime());
final Rectangle bounds = parent.getBounds();
final Point location = parent.getLocationOnScreen();
bounds.x += location.x;
bounds.y += location.y;
setLocationOnScreen(calculatePoint(bounds));
JFCUnit.flushAWT();
return true;
}
/**
* Generate a string representation of the event.
*
* @return String form of the event data.
*/
@Override
public String toString()
{
final StringBuffer buf = new StringBuffer(1000);
buf.append(super.toString());
buf.append(" amount:" + getScrollAmount());
buf.append(" rotation:" + getWheelRotation());
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy