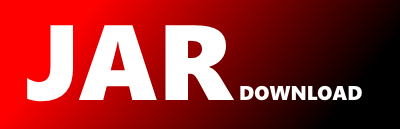
junit.extensions.jfcunit.finder.JMenuItemFinder Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.finder;
import java.awt.Component;
import javax.swing.Icon;
import javax.swing.JMenuItem;
/**
* A generic component finder which uses just the type (class) for filtering.
* The pattern syntax can be found at the Jakarta RegExp API Documentation in
* {@link org.apache.regexp.RE}. This class delegates the matching of the Icon
* to an instance of {@link junit.extensions.jfcunit.finder.IconMatcher}
*
* @author Vijay Aravamudhan :
* ThoughtWorks Inc.
*/
public class JMenuItemFinder extends Finder
{
/**
* The matcher for the icon of the {@link javax.swing.AbstractButton}
* component.
*/
private IconMatcher m_iconMatcher;
/**
* The text of the component.
*/
private String m_text = null;
/**
* A boolean specifying whether the filtration is case insensitive.
*/
private boolean m_caseIndependent = false;
/**
* Constructor accepting all arguments needed to filter the component.
*
* @param text
* The desired pattern for the text of the component.
*/
public JMenuItemFinder(final String text)
{
this(text, null, false);
}
/**
* Constructor accepting all arguments needed to filter the component.
*
* @param text
* The desired pattern for the text of the component.
* @param caseIndependent
* Whether the match should be case independent (true) or not
* (false)
*/
public JMenuItemFinder(final String text, final boolean caseIndependent)
{
this(text, null, caseIndependent);
}
/**
* Constructor accepting all arguments needed to filter the component.
*
* @param icon
* The desired pattern for the icon of the component.
*/
public JMenuItemFinder(final Icon icon)
{
this(null, icon, false);
}
/**
* Constructor accepting all arguments needed to filter the component.
*
* @param text
* The desired pattern for the text of the component.
* @param icon
* The desired pattern for the icon of the component.
*/
public JMenuItemFinder(final String text, final Icon icon)
{
this(text, icon, false);
}
/**
* Constructor accepting all arguments needed to filter the component.
*
* @param text
* The desired pattern for the text of the component.
* @param icon
* The desired pattern for the icon of the component.
* @param caseIndependent
* Whether the match should be case independent (true) or not
* (false)
*/
public JMenuItemFinder(final String text, final Icon icon, final boolean caseIndependent)
{
setText(text);
setIcon(icon);
this.m_caseIndependent = caseIndependent;
recreatePatternMatcher(this.m_text, caseIndependent);
}
/**
* Set the finder into a case independent mode.
*
* @param ignoreCase
* true if case should be ignored.
*/
@Override
public void setCaseIndependent(final boolean ignoreCase)
{
super.setCaseIndependent(ignoreCase);
this.m_caseIndependent = ignoreCase;
recreatePatternMatcher(this.m_text, this.m_caseIndependent);
}
/**
* Set the icon to be matched.
*
* @param icon
* Icon to be matched.
*/
public final void setIcon(final Icon icon)
{
try
{
if (icon == null)
{
this.m_iconMatcher = null;
} else
{
this.m_iconMatcher = new IconMatcher(icon);
}
} catch (final InterruptedException ie)
{
this.m_iconMatcher = null;
}
}
/**
* Get the icon to be matched.
*
* @return Icon to be matched.
*/
public final Icon getIcon()
{
return this.m_iconMatcher.getIcon();
}
/**
* Set the text to be matched.
*
* @param text
* to be matched to the menu item.
*/
public final void setText(final String text)
{
this.m_text = text;
recreatePatternMatcher(this.m_text, this.m_caseIndependent);
}
/**
* Get the text to be matched.
*
* @return String text for the menu item.
*/
public final String getText()
{
return this.m_text;
}
/**
* Method that returns true if the given component matches the search
* criteria.
*
* @param comp
* The component to test
* @return true if this component is a match
*/
@Override
public boolean testComponent(final Component comp)
{
// since menuItem might not be visible, we cannot use
// isValidForProcessing()
return comp != null && comp instanceof JMenuItem
&& (this.m_text == null || evaluate(((JMenuItem) comp).getText(), this.m_text))
&& (this.m_iconMatcher == null || this.m_iconMatcher.matches(((JMenuItem) comp).getIcon()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy