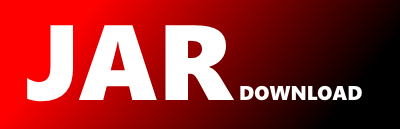
junit.extensions.jfcunit.tools.ComponentNode Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.tools;
import java.awt.Component;
import java.awt.Container;
import java.awt.Frame;
import java.awt.Window;
import java.util.Enumeration;
import java.util.Vector;
import javax.swing.tree.TreeNode;
/**
* Tree node for the ComponentBrowser application.
*
* @author Vijay Aravamudhan : ThoughtWorks Inc.
*/
public class ComponentNode implements TreeNode {
/**
* Component.
*/
private Component m_component;
/**
* Parent node.
*/
private ComponentNode m_parent;
/**
* Constructor.
*
* @param parent node of parent.
* @param comp Component to be held by the node.
*/
public ComponentNode(final ComponentNode parent, final Component comp) {
m_parent = parent;
m_component = comp;
}
/**
* Can the node support children.
*
* @return true if the node Component is a Container.
*/
public boolean getAllowsChildren() {
return (m_component == null) || m_component instanceof Container;
}
/**
* Get the specified child.
*
* @param childIndex The index of the child node to be returned.
* @return TreeNode The child node in the tree.
*/
public TreeNode getChildAt(final int childIndex) {
Component child;
if (m_component == null) {
child = (Window) getAllWindows().elementAt(childIndex);
} else if (m_component instanceof Container) {
Container container = (Container) m_component;
int cnt = container.getComponentCount();
if ((childIndex >= cnt) && container instanceof Window) {
Window[] windows = ((Window) container).getOwnedWindows();
child = windows[childIndex - cnt];
} else {
child = container.getComponent(childIndex);
}
} else {
throw new RuntimeException("no child with index " + childIndex);
}
return new ComponentNode(this, child);
}
/**
* Get the number of children.
*
* @return int Number of children.
*/
public int getChildCount() {
int num = 0;
if (m_component == null) {
num = getAllWindows().size();
} else if (m_component instanceof Container) {
Container container = (Container) m_component;
num = container.getComponentCount();
if (container instanceof Window) {
num += ((Window) container).getOwnedWindows().length;
}
}
return num;
}
/**
* Get the component held by this node of the tree.
*
* @return Component
*/
public Component getComponent() {
return m_component;
}
/**
* Get the index of the node given.
*
* @param node TreeNode for which the index should be given.
* @return int Returns the index of the node or -1 if the
* node is not found.
*/
public int getIndex(final TreeNode node) {
int index = -1;
int num = getChildCount();
for (int i = 0; i < num; i++) {
if (node.equals(getChildAt(i))) {
index = i;
break;
}
}
return index;
}
/**
* Is the node a leaf.
*
* @return boolean True if the node has no children.
*/
public boolean isLeaf() {
return !getAllowsChildren() || (getChildCount() == 0);
}
/**
* Get the parent node.
*
* @return TreeNode Reference to parent.
*/
public TreeNode getParent() {
return m_parent;
}
/**
* Get the children of this node.
*
* @return Enumeration of the children of type ComponentNode.
*/
public Enumeration children() {
Vector kids = new Vector();
int num = getChildCount();
for (int i = 0; i < num; i++) {
kids.add(getChildAt(i));
}
return kids.elements();
}
/**
* Check equality.
*
* @param other Object to be compared.
* @return boolean true if the objects are equal.
*/
public boolean equals(final Object other) {
return ((other instanceof ComponentNode)
&& (m_component == ((ComponentNode) other).m_component));
}
/**
* The hashCode of this node.
*
* @return int hashCode of the referenced Component or 1
* if no component is referenced (ex. Top of Tree).
*/
public int hashCode() {
if (m_component == null) {
return 1;
}
return m_component.hashCode();
}
/**
* Generate a description of the object.
*
* @return String description of this object.
*/
public String toString() {
if (m_component == null) {
return "All Frames";
}
// mComponent is not null
if (m_component.getName() == null) {
return (m_component.getClass().getName() + " : "
+ m_component.hashCode());
}
return m_component.getName();
}
/**
* This method is used to build a Vector of all open windows (Frames)
* after filtering the ComponentBrowser window.
*
* @return Vector A list of all windows excluding the ComponentBrowser window
*/
private Vector getAllWindows() {
Window[] allWindows = Frame.getFrames();
Vector filteredWindows = new Vector(allWindows.length);
if ((allWindows == null) || (allWindows.length == 0)) {
filteredWindows.trimToSize();
return filteredWindows;
}
for (int i = 0; i < allWindows.length; i++) {
if ((allWindows[i] != null)
&& allWindows[i] instanceof Frame
&& allWindows[i].isShowing()
&& (!ComponentBrowser.TITLE.equals(
((Frame) allWindows[i]).getTitle()))) {
filteredWindows.addElement(allWindows[i]);
}
}
filteredWindows.trimToSize();
return filteredWindows;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy