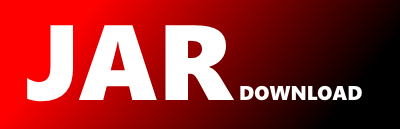
junit.extensions.jfcunit.tools.JFCUtilities Maven / Gradle / Ivy
The newest version!
package junit.extensions.jfcunit.tools;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Toolkit;
import java.awt.Window;
import javax.swing.SwingUtilities;
/**
* JFCUtilities provides convenience methods for frequently needed GUI programming operations.
*
* @author Vijay Aravamudhan : ThoughtWorks Inc.
*/
public final class JFCUtilities {
/**
* Hide the constructor.
*/
private JFCUtilities() {
}
/**
* A utility method to center a Window
.
*
* @param win The Window to be centered.
*/
public static void center(final Window win) {
center(win, null);
}
/**
* A utility method to center a Window
with respect to its parent Window
.
*
* @param win The Window to be centered.
* @param comp The parent Component
of the above Window
.
*/
public static void center(final Window win, final Component comp) {
Window parentWin = null;
if (comp != null) {
parentWin = SwingUtilities.windowForComponent(comp);
}
Dimension winSize = win.getSize();
Dimension parentSize = Toolkit.getDefaultToolkit().getScreenSize();
int startX = 0;
int startY = 0;
if (parentWin != null) {
startX = parentWin.getLocation().x;
startY = parentWin.getLocation().y;
parentSize = parentWin.getSize();
}
// If child window is larger than parent window, startX or startY could be negative
// If the calculated value is negative, use 0 instead
win.setLocation(
Math.max(0, startX + ((parentSize.width - winSize.width) / 2)),
Math.max(0, startY + ((parentSize.height - winSize.height) / 2)));
}
/**
* A utility method to find a Component in the component's parent hierarchy which is of the type
* specified.
*
* @param comp The Component whose hierarchy has to be searched for the JViewport.
* @param clazz The type of the required parent
* @return The parent that contains the component or one of its parents, null if not found.
*/
public static Component findComponentInHierarchy(final Component comp,
final Class clazz) {
if (comp == null) {
return null;
}
Component parent = comp.getParent();
while ((parent != null) && !(clazz.isInstance(parent))) {
parent = parent.getParent();
}
if (clazz.isInstance(parent)) {
return parent;
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy