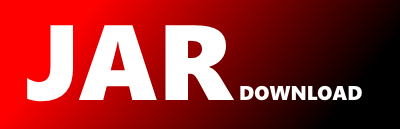
junit.extensions.xml.XMLException Maven / Gradle / Ivy
The newest version!
package junit.extensions.xml;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.PrintWriter;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
/**
* This exception is thrown when the XML cannot be parsed properly.
*
* @author Kevin Wilson
*/
public class XMLException extends RuntimeException {
/**
* Element which could not be processed.
*/
private Element m_element;
/**
* Exception which caused the error.
*/
private Throwable m_cause;
/**
* Properties which may have been passed to the element
* which could not be processed.
*/
private XMLObjectCache m_properties;
/**
* Constructor accepting a String message.
* @param msg Message of the exception.
* @see java.lang.Throwable#Throwable(String)
*/
public XMLException(final String msg) {
this(msg, null, null, null);
}
/**
* Constructor accepting a String message.
* @param msg Message of the exception.
* @param e Element which throw the exception.
* @see java.lang.Throwable#Throwable(String)
*/
public XMLException(final String msg, final Element e) {
this(msg, null, e, null);
}
/**
* Constructor accepting a String message.
* @param msg Message of the exception.
* @param cause Exception or error which caused this exception
* to be thrown.
* @param element Element which throw the exception.
* @param properties Properties cache containing the values
* which were passed to the tag handler.
* @see java.lang.Throwable#Throwable(String)
*/
public XMLException(final String msg, final Throwable cause,
final Element element, final XMLObjectCache properties) {
/** @todo when depricating 1.3 */
// super(msg, cause);
super(msg);
/** @todo remove when depricating 1.3 and implementing the above. */
m_cause = cause;
m_element = element;
m_properties = properties;
}
/**
* Get the element which through the exception.
* @return Element which through the exception.
*/
public Element getElement() {
return m_element;
}
/**
* Add context from the element.
* @return String representing the context of the exception.
*/
public String toString() {
try {
ByteArrayOutputStream os = new ByteArrayOutputStream(50000);
PrintWriter pw = new PrintWriter(os);
pw.println(super.toString());
XMLWriter xmlw = new XMLWriter(true);
xmlw.setOutput(pw);
Element e = getElement();
if (e != null) {
pw.println("While processing element:");
xmlw.write(e);
pw.println();
Node parent = e.getParentNode();
if (parent != null) {
pw.println("Within:");
xmlw.write(parent);
pw.println();
}
if (m_properties != null) {
String[] names = m_properties.getNames();
boolean first = true;
for (int i = 0; i < names.length; i++) {
if ((names[i] != null) && !names[i].startsWith("../")) {
if (first) {
pw.println("Local Parameters:");
first = false;
}
Object value = m_properties.get(names[i]);
pw.println(names[i] + " = " + value);
}
}
}
}
pw.flush();
os.close();
return os.toString();
} catch (IOException ioe) {
ioe.printStackTrace();
}
return super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy