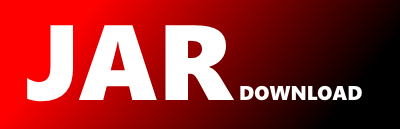
org.bibucket.village.land.CadastralNumber Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of village-model Show documentation
Show all versions of village-model Show documentation
This is village model classes
The newest version!
package org.bibucket.village.land;
import javax.annotation.Nonnull;
import java.text.MessageFormat;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Кадастровый номер участка
*/
public final class CadastralNumber {
private static final Pattern PATTERN =
Pattern.compile(
"^([0-9]+):([0-9]+):([0-9]{6,7}):([0-9]+)$"
);
/**
* кадастровый округ
*/
private final String canton;
/**
* кадастровый район
*/
private final String district;
/**
* кадастровый квартал
*/
private final String quarter;
/**
* номер объекта недвижимости
*/
private final String number;
public CadastralNumber(String number) {
Matcher matcher = PATTERN.matcher(number);
if (!matcher.matches()) {
throw new IllegalArgumentException(
MessageFormat.format("{0} - не кадастровый номер", number)
);
}
canton = matcher.group(1);
district = matcher.group(2);
quarter = matcher.group(3);
this.number = matcher.group(4);
}
@Nonnull
public String canton() {
return canton;
}
@Nonnull
public String district() {
return district;
}
@Nonnull
public String quarter() {
return quarter;
}
@Nonnull
public String number() {
return number;
}
@Override
public int hashCode() {
return number.hashCode();
}
@Override
public boolean equals(Object other) {
return other == this || (
other != null
&& other.getClass() == CadastralNumber.class
&& ((CadastralNumber) other).canton.equals(this.canton)
&& ((CadastralNumber) other).district.equals(this.district)
&& ((CadastralNumber) other).quarter.equals(this.quarter)
&& ((CadastralNumber) other).number.equals(this.number)
);
}
@Override
public String toString() {
return canton + ":" + district + ":" + quarter + ":" + number;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy