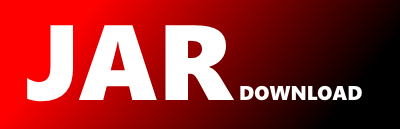
Junit_tests.RFMDiagnosticMessageUnitTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rfppf Show documentation
Show all versions of rfppf Show documentation
RF-Networks Protocol Parser Framework
The newest version!
/*
* Copyright (c) 2019, RF-Networks Ltd.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code are not permitted.
*
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* * Neither the name of RF Networks Ltd. nor the names of
* its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR
* OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package Junit_tests;
import static org.junit.Assert.*;
import java.nio.charset.StandardCharsets;
import org.junit.Test;
import org.bitbucket.rfnetwork.rfppf.messages.MessageFactory;
import org.bitbucket.rfnetwork.rfppf.messages.rfm.RFMMessage;
import org.bitbucket.rfnetwork.rfppf.messages.rfm.diagnostic.*;
public class RFMDiagnosticMessageUnitTest {
@Test
public void TestEmptyDiagnosticMessage() {
byte version = 1;
byte message_num = 2;
byte[] test_msg = new byte[] { (byte)0x96, version, 0x00, (byte)RFMMessage.MessageTypes.Diagnostic.getValue(), 0x02, (byte)0x6C, (byte)0xB8 };
DiagnosticMessageBuilder builder = new DiagnosticMessageBuilder();
builder.Version = version;
builder.MessageType = RFMMessage.MessageTypes.Diagnostic;
builder.MessageNumber = message_num;
DiagnosticMessage msg = (DiagnosticMessage)MessageFactory.CreateMessage(DiagnosticMessage.class, builder);
assertEquals(msg.getVersion(), version);
assertEquals(msg.getMessageNumber(), message_num);
assertEquals(msg.getMessageType(), RFMMessage.MessageTypes.Diagnostic);
assertArrayEquals(msg.GetBytes(), test_msg);
// Test message parsing
msg = (DiagnosticMessage)MessageFactory.CreateMessage(DiagnosticMessage.class);
msg.Parse(test_msg);
assertArrayEquals(msg.GetBytes(), test_msg);
}
@Test
public void TestDiagnosticMessage() {
byte version = 1;
byte message_num = 2;
String message = "Test.";
byte[] test_msg = new byte[] { (byte)0x96, version, 0x05, (byte)RFMMessage.MessageTypes.Diagnostic.getValue(), 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, (byte)0x87, (byte)0x8B };
System.arraycopy(message.getBytes(StandardCharsets.US_ASCII), 0, test_msg, 5, message.length());
DiagnosticMessageBuilder builder = new DiagnosticMessageBuilder();
builder.Version = version;
builder.MessageType = RFMMessage.MessageTypes.Diagnostic;
builder.MessageNumber = message_num;
builder.Message = message;
DiagnosticMessage msg = (DiagnosticMessage)MessageFactory.CreateMessage(DiagnosticMessage.class, builder);
assertEquals(msg.getVersion(), version);
assertEquals(msg.getMessageNumber(), message_num);
assertEquals(msg.getMessageType(), RFMMessage.MessageTypes.Diagnostic);
assertEquals(msg.getMessage(), message);
assertArrayEquals(msg.GetBytes(), test_msg);
// Test message parsing
msg = (DiagnosticMessage)MessageFactory.CreateMessage(DiagnosticMessage.class);
msg.Parse(test_msg);
assertArrayEquals(msg.GetBytes(), test_msg);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy