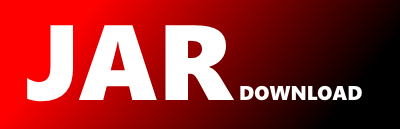
Junit_tests.RFMMessageUnitTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rfppf Show documentation
Show all versions of rfppf Show documentation
RF-Networks Protocol Parser Framework
The newest version!
/*
* Copyright (c) 2019, RF-Networks Ltd.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code are not permitted.
*
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* * Neither the name of RF Networks Ltd. nor the names of
* its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR
* OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package Junit_tests;
import static org.junit.Assert.*;
import org.junit.Test;
import org.bitbucket.rfnetwork.rfppf.common.RFPPFHelper;
import org.bitbucket.rfnetwork.rfppf.messages.MessageFactory;
import org.bitbucket.rfnetwork.rfppf.messages.rfm.*;
import org.bitbucket.rfnetwork.rfppf.messages.rfm.data.sensors.DS18B20SensorMessage;
public class RFMMessageUnitTest {
@Test
public void TestEmptyMessage() {
byte[] msg_bytes = new byte[] { (byte)0x96, 0x00, 0x00, (byte)0xFF, 0x00, (byte)0xF0, (byte)0xE8 };
RFMMessage msg = (RFMMessage)MessageFactory.CreateMessage(RFMMessage.class);
assertArrayEquals(msg.GetBytes(), msg_bytes);
msg.Parse(msg_bytes);
assertArrayEquals(msg.GetBytes(), msg_bytes);
}
@Test
public void TestMessageBuilder() {
RFMMessageBuilder builder = new RFMMessageBuilder();
builder.MessageType = RFMMessage.MessageTypes.Unknown;
builder.MessageNumber = 1;
builder.Version = 1;
byte[] msg_bytes = new byte[] { (byte)0x96, 0x01, 0x00, (byte)0xFF, 0x01, 0x65, (byte)0x8E };
RFMMessage msg = (RFMMessage)MessageFactory.CreateMessage(RFMMessage.class, builder);
assertArrayEquals(msg.GetBytes(), msg_bytes);
msg.Parse(msg_bytes);
assertArrayEquals(msg.GetBytes(), msg_bytes);
}
@Test
public void TestCRC() {
RFMMessage meshMessage = null;
byte[] msg_bytes = new byte[] { (byte)0x96, 0x01, 0x10, 0x21, 0x2D, 0x2D, 0x0E, 0x66, (byte)0xCA, 0x1B, (byte)0xF0, (byte)0xF1, (byte)0xCC, 0x5F, 0x1B, (byte)0xF2, (byte)0xCC, 0x5F, 0x30, 0x68, 0x01, 0x06, (byte)0xF1 };
meshMessage = (RFMMessage) MessageFactory.ParseMeshMessage(msg_bytes);
String before = RFPPFHelper.ByteArrayToHexString(meshMessage.GetBytes());
((DS18B20SensorMessage)meshMessage).setTemperature(23.5f);
String after = RFPPFHelper.ByteArrayToHexString(meshMessage.GetBytes());
msg_bytes = new byte[] { (byte)0x96, 0x01, 0x0E, 0x43, (byte)0xF7, 0x5F, (byte)0x9C, 0x44, 0x1E, 0x01, 0x00, 0x2A, (byte)0xF9, (byte)0xCC, 0x5F, 0x01, 0x01, 0x00, 0x00, 0x02, (byte)0xEA };
meshMessage = (RFMMessage) MessageFactory.ParseMeshMessage(msg_bytes);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy