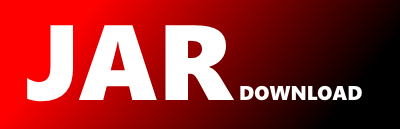
Junit_tests.oldprotocol.ReceiverModbusMeterMessageUnitTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rfppf Show documentation
Show all versions of rfppf Show documentation
RF-Networks Protocol Parser Framework
The newest version!
/*
* Copyright (c) 2019, RF-Networks Ltd.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code are not permitted.
*
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* * Neither the name of RF Networks Ltd. nor the names of
* its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR
* OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package Junit_tests.oldprotocol;
import static org.junit.Assert.assertArrayEquals;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.fail;
import org.bitbucket.rfnetwork.rfppf.messages.MessageFactory;
import org.bitbucket.rfnetwork.rfppf.messages.oldprotocol.ReceiverModbusMeterMessage;
import org.junit.Test;
public class ReceiverModbusMeterMessageUnitTest {
@Test
public void TestParseModbusMeterMessage()
{
byte[] msg_bytes = { 0x37, 0x1E, 0x4B, (byte)0x9A, 0x19, 0x5C, 0x43, 0x33, 0x33, 0x5C, 0x43, (byte)0xCD, 0x4C, 0x5C, 0x43, 0x5C, 0x0F, (byte)0xC8, 0x43, 0x71, 0x1D, (byte)0xC8, 0x43, (byte)0xAE, 0x27, (byte)0xC8, 0x43, 0x66, 0x66, 0x48, 0x42, (byte)0xCD, (byte)0xCC, 0x48, 0x42, 0x33, 0x33, 0x49, 0x42, 0x33, 0x33, (byte)0xD3, 0x40, (byte)0xCD, (byte)0xCC, (byte)0x8C, 0x3F, (byte)0xCD, (byte)0xCC, 0x0C, 0x40, 0x33, 0x33, 0x53, 0x40, (byte)0x8C };
ReceiverModbusMeterMessage msg = (ReceiverModbusMeterMessage)MessageFactory.CreateMessage(ReceiverModbusMeterMessage.class);
msg.setMessageNumber(75);
msg.setPhase1Voltage(220.1f);
msg.setPhase2Voltage(220.2f);
msg.setPhase3Voltage(220.3f);
msg.setLine1ToLine2Voltage(400.12f);
msg.setLine2ToLine3Voltage(400.23f);
msg.setLine3ToLine1Voltage(400.31f);
msg.setPhase1Current(50.1f);
msg.setPhase2Current(50.2f);
msg.setPhase3Current(50.3f);
msg.setTotalPower(6.6f);
msg.setPhase1Power(1.1f);
msg.setPhase2Power(2.2f);
msg.setPhase3Power(3.3f);
assertArrayEquals(msg.GetBytes(), msg_bytes);
try {
msg.Parse(msg_bytes);
} catch (Exception e) {
fail();
}
assertEquals(msg.getPhase1Voltage(), 220.1f, 0.1f);
assertEquals(msg.getPhase2Voltage(), 220.2f, 0.1f);
assertEquals(msg.getPhase3Voltage(), 220.3f, 0.1f);
assertEquals(msg.getLine1ToLine2Voltage(), 400.12f, 0.01f);
assertEquals(msg.getLine2ToLine3Voltage(), 400.23f, 0.01f);
assertEquals(msg.getLine3ToLine1Voltage(), 400.31f, 0.01f);
assertEquals(msg.getPhase1Current(), 50.1f, 0.1f);
assertEquals(msg.getPhase2Current(), 50.2f, 0.1f);
assertEquals(msg.getPhase3Current(), 50.3f, 0.1f);
assertEquals(msg.getTotalPower(), 6.6f, 0.1f);
assertEquals(msg.getPhase1Power(), 1.1f, 0.1f);
assertEquals(msg.getPhase2Power(), 2.2f, 0.1f);
assertEquals(msg.getPhase3Power(), 3.3f, 0.1f);
assertEquals(msg.getMessageNumber(), 75);
assertArrayEquals(msg.GetBytes(), msg_bytes);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy