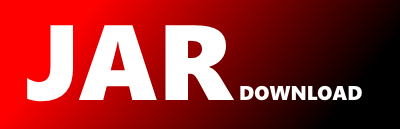
Junit_tests.Gateway.GatewayRPCRequestMessageUnitTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rfppf Show documentation
Show all versions of rfppf Show documentation
RF-Networks Protocol Parser Framework
/*
* Copyright (c) 2019, RF-Networks Ltd.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code are not permitted.
*
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* * Neither the name of RF Networks Ltd. nor the names of
* its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR
* OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package Junit_tests.Gateway;
import static org.junit.Assert.assertArrayEquals;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.fail;
import java.math.BigInteger;
import java.util.UUID;
import org.bitbucket.rfnetwork.rfppf.common.RFPPFHelper;
import org.bitbucket.rfnetwork.rfppf.messages.MessageFactory;
import org.bitbucket.rfnetwork.rfppf.messages.gprs.gateway.GatewayRPCRequestMessage;
import org.junit.Test;
public class GatewayRPCRequestMessageUnitTest {
@Test
public void TestParseNoDataRPCRequestMessage() {
UUID uuid = UUID.fromString("4865e243-fd18-4d65-ad7a-18903c2f9c4d");
BigInteger guidint = new BigInteger(1, RFPPFHelper.UUIDtoBytesArray(uuid));
byte[] msg_bytes = { 0x03, 0x43, (byte)0xE2, 0x65, 0x48, 0x18, (byte)0xFD, 0x65, 0x4D, (byte)0xAD, 0x7A, 0x18, (byte)0x90, 0x3C, 0x2F, (byte)0x9C, 0x4D, (byte)0xBF };
try {
// Test building
GatewayRPCRequestMessage msg = (GatewayRPCRequestMessage)MessageFactory.CreateMessage(GatewayRPCRequestMessage.class);
msg.setRPCID(guidint);
msg.setPayload(new byte[] {});
assertArrayEquals(msg.GetBytes(), msg_bytes);
// Test parsing
msg.Parse(msg_bytes);
assertEquals(msg.getRPCID(), guidint);
assertArrayEquals(msg.getPayload(), new byte[] {});
assertArrayEquals(msg.GetBytes(), msg_bytes);
} catch (Exception e) {
fail();
}
}
@Test
public void TestParseRPCRequestMessage() {
UUID uuid = UUID.fromString("4865e243-fd18-4d65-ad7a-18903c2f9c4d");
BigInteger guidint = new BigInteger(1, RFPPFHelper.UUIDtoBytesArray(uuid));
byte[] msg_bytes = { 0x03, 0x43, (byte)0xE2, 0x65, 0x48, 0x18, (byte)0xFD, 0x65, 0x4D, (byte)0xAD, 0x7A, 0x18, (byte)0x90, 0x3C, 0x2F, (byte)0x9C, 0x4D, 0x01, (byte)0xC0 };
try {
// Test building
GatewayRPCRequestMessage msg = (GatewayRPCRequestMessage)MessageFactory.CreateMessage(GatewayRPCRequestMessage.class);
msg.setRPCID(guidint);
msg.setPayload(new byte[] { 0x01 });
assertArrayEquals(msg.GetBytes(), msg_bytes);
// Test parsing
msg.Parse(msg_bytes);
assertEquals(msg.getRPCID(), guidint);
assertArrayEquals(msg.getPayload(), new byte[] { 0x01 });
assertArrayEquals(msg.GetBytes(), msg_bytes);
} catch (Exception e) {
fail();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy