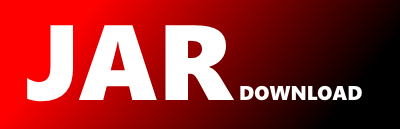
org.swat.json.utils.JsonUtil Maven / Gradle / Ivy
/*
* Copyright 2017 Swatantra Agrawal. All rights reserved.
*/
package org.swat.json.utils;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.CollectionType;
import com.fasterxml.jackson.databind.type.MapType;
import com.fasterxml.jackson.databind.type.TypeFactory;
import org.swat.core.utils.CoreInterceptor;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by Swatantra Agrawal
* on 27-AUG-2017
*/
public final class JsonUtil {
public static final JsonUtil JSON_UTIL = new JsonUtil();
public static final JsonUtil JSON_SILENT = CoreInterceptor.silent(JSON_UTIL);
public static final JsonUtil JSON_LOGGED = CoreInterceptor.logged(JSON_UTIL);
public static final JsonUtil JSON_RUNTIME = CoreInterceptor.runtime(JSON_UTIL);
private final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
private JsonUtil() {
OBJECT_MAPPER.setSerializationInclusion(JsonInclude.Include.NON_NULL);
}
/**
* Parses the json to desired type
*
* @param jsonStream The input stream
* @param clazz The Object type
* @param Generics
* @return The parsed Object.
* @throws IOException in case of any Exception
*/
public T readObject(InputStream jsonStream, Class clazz) throws IOException {
return OBJECT_MAPPER.readValue(jsonStream, clazz);
}
/**
* Parses the json to List of desired type
*
* @param stream The JSON stream
* @param clazz The Object type
* @param Generics
* @return The parsed List.
* @throws IOException in case of any Exception
*/
public List readList(InputStream stream, Class clazz) throws IOException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
CollectionType collectionType = typeFactory.constructCollectionType(ArrayList.class, clazz);
return OBJECT_MAPPER.readValue(stream, collectionType);
}
/**
* Parses the json to List of desired type
*
* @param json The JSON
* @param clazz The Object type
* @param Generics
* @return The parsed List.
* @throws IOException in case of any Exception
*/
public List readList(String json, Class clazz) throws IOException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
CollectionType collectionType = typeFactory.constructCollectionType(ArrayList.class, clazz);
return OBJECT_MAPPER.readValue(json, collectionType);
}
/**
* Parses the json to Map of desired type
*
* @param json The JSON
* @param keyClass The Key Type
* @param valueClass The Value Type
* @param Generics
* @param Generics
* @return The parsed Map.
* @throws IOException in case of any Exception
*/
public Map readMap(String json, Class keyClass, Class valueClass) throws IOException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
MapType mapType = typeFactory.constructMapType(HashMap.class, keyClass, valueClass);
return OBJECT_MAPPER.readValue(json, mapType);
}
/**
* Method convert to json string for the input object
*
* @param object {@link Object} - The input object
* @return {@link String} - The output json string
* @throws JsonProcessingException
*/
public String toJsonString(Object object) throws JsonProcessingException {
return OBJECT_MAPPER.writeValueAsString(object);
}
/**
* Reads json as Object
*
* @param json The json
* @param clazz The class of Object
* @param The type of Object
* @return The object
* @throws IOException in case of any Exception
*/
public T readObject(String json, Class clazz) throws IOException {
return OBJECT_MAPPER.readValue(json, clazz);
}
/**
* Returns prettified json of the object
*
* @param object The object
* @return The prettified json
* @throws IOException in case of any Exception
*/
public String pretty(Object object) throws IOException {
return OBJECT_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy