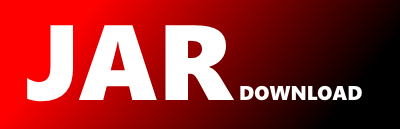
org.swat.json.utils.JsonUtilCH Maven / Gradle / Ivy
/*
* Copyright © 2018 Swatantra Agrawal. All rights reserved.
*/
package org.swat.json.utils;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.map.type.CollectionType;
import org.codehaus.jackson.map.type.MapType;
import org.codehaus.jackson.map.type.TypeFactory;
import org.swat.core.utils.CoreRtException;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* The Utilities for Json. In encapsulates ObjectMapper and provides additional method to deal with Json
* It is immutable
*
* @author Swatantra Agrawal on 02-JUN-2018
*/
public class JsonUtilCH implements JsonUtil {
private final ObjectMapper OBJECT_MAPPER;
/**
* Instantiates a new Json util.
*/
public JsonUtilCH() {
this(new ObjectMapper());
}
/**
* Instantiates a new Json util ch.
*
* @param objectMapper the object mapper
*/
public JsonUtilCH(ObjectMapper objectMapper) {
OBJECT_MAPPER = objectMapper;
}
@Override
public T readObject(InputStream jsonStream, Class clazz) throws CoreRtException {
try {
return OBJECT_MAPPER.readValue(jsonStream, clazz);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
@Override
public List readList(InputStream stream, Class clazz) throws CoreRtException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
CollectionType collectionType = typeFactory.constructCollectionType(ArrayList.class, clazz);
try {
return OBJECT_MAPPER.readValue(stream, collectionType);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
@Override
public List readList(String json, Class clazz) throws CoreRtException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
CollectionType collectionType = typeFactory.constructCollectionType(ArrayList.class, clazz);
try {
return OBJECT_MAPPER.readValue(json, collectionType);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
@Override
public Map readMap(String json, Class keyClass, Class valueClass) throws CoreRtException {
TypeFactory typeFactory = TypeFactory.defaultInstance();
MapType mapType = typeFactory.constructMapType(HashMap.class, keyClass, valueClass);
try {
return OBJECT_MAPPER.readValue(json, mapType);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
@Override
public String toJsonString(Object object) throws CoreRtException {
try {
return OBJECT_MAPPER.writeValueAsString(object);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
/**
* Reads json as Object
*
* @param Generics
* @param json The json
* @param clazz The class of Object
* @return The object
* @throws CoreRtException in case of any Exception
*/
public T readObject(String json, Class clazz) throws CoreRtException {
try {
return OBJECT_MAPPER.readValue(json, clazz);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
/**
* Returns prettified json of the object
*
* @param object The object
* @return The prettified json
* @throws CoreRtException in case of any Exception
*/
public String pretty(Object object) throws CoreRtException {
try {
return OBJECT_MAPPER.writerWithDefaultPrettyPrinter().writeValueAsString(object);
} catch (IOException e) {
throw new CoreRtException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy