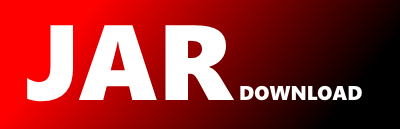
chainrpc.ChainKit.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package chainrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* ChainKit is a service that can be used to get information from the
* chain backend.
*/
@PekkoGrpcGenerated
trait ChainKit {
/**
* lncli: `chain getblock`
* GetBlock returns a block given the corresponding block hash.
*/
def getBlock(in: chainrpc.GetBlockRequest): scala.concurrent.Future[chainrpc.GetBlockResponse]
/**
* lncli: `chain getblockheader`
* GetBlockHeader returns a block header with a particular block hash.
*/
def getBlockHeader(in: chainrpc.GetBlockHeaderRequest): scala.concurrent.Future[chainrpc.GetBlockHeaderResponse]
/**
* lncli: `chain getbestblock`
* GetBestBlock returns the block hash and current height from the valid
* most-work chain.
*/
def getBestBlock(in: chainrpc.GetBestBlockRequest): scala.concurrent.Future[chainrpc.GetBestBlockResponse]
/**
* lncli: `chain getblockhash`
* GetBlockHash returns the hash of the block in the best blockchain
* at the given height.
*/
def getBlockHash(in: chainrpc.GetBlockHashRequest): scala.concurrent.Future[chainrpc.GetBlockHashResponse]
}
@PekkoGrpcGenerated
object ChainKit extends pekko.grpc.ServiceDescription {
val name = "chainrpc.ChainKit"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
chainrpc.ChainkitProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val GetBlockRequestSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockRequest.messageCompanion)
val GetBlockHeaderRequestSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockHeaderRequest.messageCompanion)
val GetBestBlockRequestSerializer = new ScalapbProtobufSerializer(chainrpc.GetBestBlockRequest.messageCompanion)
val GetBlockHashRequestSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockHashRequest.messageCompanion)
val GetBlockResponseSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockResponse.messageCompanion)
val GetBlockHeaderResponseSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockHeaderResponse.messageCompanion)
val GetBestBlockResponseSerializer = new ScalapbProtobufSerializer(chainrpc.GetBestBlockResponse.messageCompanion)
val GetBlockHashResponseSerializer = new ScalapbProtobufSerializer(chainrpc.GetBlockHashResponse.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val getBlockDescriptor: MethodDescriptor[chainrpc.GetBlockRequest, chainrpc.GetBlockResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainKit", "GetBlock"))
.setRequestMarshaller(new Marshaller(GetBlockRequestSerializer))
.setResponseMarshaller(new Marshaller(GetBlockResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getBlockHeaderDescriptor: MethodDescriptor[chainrpc.GetBlockHeaderRequest, chainrpc.GetBlockHeaderResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainKit", "GetBlockHeader"))
.setRequestMarshaller(new Marshaller(GetBlockHeaderRequestSerializer))
.setResponseMarshaller(new Marshaller(GetBlockHeaderResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getBestBlockDescriptor: MethodDescriptor[chainrpc.GetBestBlockRequest, chainrpc.GetBestBlockResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainKit", "GetBestBlock"))
.setRequestMarshaller(new Marshaller(GetBestBlockRequestSerializer))
.setResponseMarshaller(new Marshaller(GetBestBlockResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getBlockHashDescriptor: MethodDescriptor[chainrpc.GetBlockHashRequest, chainrpc.GetBlockHashResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainKit", "GetBlockHash"))
.setRequestMarshaller(new Marshaller(GetBlockHashRequestSerializer))
.setResponseMarshaller(new Marshaller(GetBlockHashResponseSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy