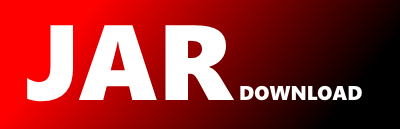
chainrpc.ChainKitClient.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package chainrpc
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.actor.ClassicActorSystemProvider
import pekko.grpc.GrpcChannel
import pekko.grpc.GrpcClientCloseException
import pekko.grpc.GrpcClientSettings
import pekko.grpc.scaladsl.PekkoGrpcClient
import pekko.grpc.internal.NettyClientUtils
import pekko.grpc.PekkoGrpcGenerated
import pekko.grpc.scaladsl.SingleResponseRequestBuilder
import pekko.grpc.internal.ScalaUnaryRequestBuilder
// Not sealed so users can extend to write their stubs
@PekkoGrpcGenerated
trait ChainKitClient extends ChainKit with ChainKitClientPowerApi with PekkoGrpcClient
@PekkoGrpcGenerated
object ChainKitClient {
def apply(settings: GrpcClientSettings)(implicit sys: ClassicActorSystemProvider): ChainKitClient =
new DefaultChainKitClient(GrpcChannel(settings), isChannelOwned = true)
def apply(channel: GrpcChannel)(implicit sys: ClassicActorSystemProvider): ChainKitClient =
new DefaultChainKitClient(channel, isChannelOwned = false)
private class DefaultChainKitClient(channel: GrpcChannel, isChannelOwned: Boolean)(implicit sys: ClassicActorSystemProvider) extends ChainKitClient {
import ChainKit.MethodDescriptors._
private implicit val ex: ExecutionContext = sys.classicSystem.dispatcher
private val settings = channel.settings
private val options = NettyClientUtils.callOptions(settings)
private def getBlockRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(getBlockDescriptor, channel, options, settings)
private def getBlockHeaderRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(getBlockHeaderDescriptor, channel, options, settings)
private def getBestBlockRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(getBestBlockDescriptor, channel, options, settings)
private def getBlockHashRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(getBlockHashDescriptor, channel, options, settings)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlock(chainrpc.GetBlockRequest) if possible.
*/
override def getBlock(): SingleResponseRequestBuilder[chainrpc.GetBlockRequest, chainrpc.GetBlockResponse] =
getBlockRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of getBlock
*/
def getBlock(in: chainrpc.GetBlockRequest): scala.concurrent.Future[chainrpc.GetBlockResponse] =
getBlock().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlockHeader(chainrpc.GetBlockHeaderRequest) if possible.
*/
override def getBlockHeader(): SingleResponseRequestBuilder[chainrpc.GetBlockHeaderRequest, chainrpc.GetBlockHeaderResponse] =
getBlockHeaderRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of getBlockHeader
*/
def getBlockHeader(in: chainrpc.GetBlockHeaderRequest): scala.concurrent.Future[chainrpc.GetBlockHeaderResponse] =
getBlockHeader().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBestBlock(chainrpc.GetBestBlockRequest) if possible.
*/
override def getBestBlock(): SingleResponseRequestBuilder[chainrpc.GetBestBlockRequest, chainrpc.GetBestBlockResponse] =
getBestBlockRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of getBestBlock
*/
def getBestBlock(in: chainrpc.GetBestBlockRequest): scala.concurrent.Future[chainrpc.GetBestBlockResponse] =
getBestBlock().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlockHash(chainrpc.GetBlockHashRequest) if possible.
*/
override def getBlockHash(): SingleResponseRequestBuilder[chainrpc.GetBlockHashRequest, chainrpc.GetBlockHashResponse] =
getBlockHashRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of getBlockHash
*/
def getBlockHash(in: chainrpc.GetBlockHashRequest): scala.concurrent.Future[chainrpc.GetBlockHashResponse] =
getBlockHash().invoke(in)
override def close(): scala.concurrent.Future[pekko.Done] =
if (isChannelOwned) channel.close()
else throw new GrpcClientCloseException()
override def closed: scala.concurrent.Future[pekko.Done] = channel.closed()
}
}
@PekkoGrpcGenerated
trait ChainKitClientPowerApi {
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlock(chainrpc.GetBlockRequest) if possible.
*/
def getBlock(): SingleResponseRequestBuilder[chainrpc.GetBlockRequest, chainrpc.GetBlockResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlockHeader(chainrpc.GetBlockHeaderRequest) if possible.
*/
def getBlockHeader(): SingleResponseRequestBuilder[chainrpc.GetBlockHeaderRequest, chainrpc.GetBlockHeaderResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBestBlock(chainrpc.GetBestBlockRequest) if possible.
*/
def getBestBlock(): SingleResponseRequestBuilder[chainrpc.GetBestBlockRequest, chainrpc.GetBestBlockResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer getBlockHash(chainrpc.GetBlockHashRequest) if possible.
*/
def getBlockHash(): SingleResponseRequestBuilder[chainrpc.GetBlockHashRequest, chainrpc.GetBlockHashResponse] = ???
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy