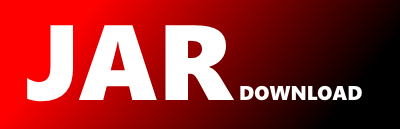
chainrpc.ChainNotifier.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package chainrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* ChainNotifier is a service that can be used to get information about the
* chain backend by registering notifiers for chain events.
*/
@PekkoGrpcGenerated
trait ChainNotifier {
/**
* RegisterConfirmationsNtfn is a synchronous response-streaming RPC that
* registers an intent for a client to be notified once a confirmation request
* has reached its required number of confirmations on-chain.
* A confirmation request must have a valid output script. It is also possible
* to give a transaction ID. If the transaction ID is not set, a notification
* is sent once the output script confirms. If the transaction ID is also set,
* a notification is sent once the output script confirms in the given
* transaction.
*/
def registerConfirmationsNtfn(in: chainrpc.ConfRequest): org.apache.pekko.stream.scaladsl.Source[chainrpc.ConfEvent, org.apache.pekko.NotUsed]
/**
* RegisterSpendNtfn is a synchronous response-streaming RPC that registers an
* intent for a client to be notification once a spend request has been spent
* by a transaction that has confirmed on-chain.
* A client can specify whether the spend request should be for a particular
* outpoint or for an output script by specifying a zero outpoint.
*/
def registerSpendNtfn(in: chainrpc.SpendRequest): org.apache.pekko.stream.scaladsl.Source[chainrpc.SpendEvent, org.apache.pekko.NotUsed]
/**
* RegisterBlockEpochNtfn is a synchronous response-streaming RPC that
* registers an intent for a client to be notified of blocks in the chain. The
* stream will return a hash and height tuple of a block for each new/stale
* block in the chain. It is the client's responsibility to determine whether
* the tuple returned is for a new or stale block in the chain.
* A client can also request a historical backlog of blocks from a particular
* point. This allows clients to be idempotent by ensuring that they do not
* missing processing a single block within the chain.
*/
def registerBlockEpochNtfn(in: chainrpc.BlockEpoch): org.apache.pekko.stream.scaladsl.Source[chainrpc.BlockEpoch, org.apache.pekko.NotUsed]
}
@PekkoGrpcGenerated
object ChainNotifier extends pekko.grpc.ServiceDescription {
val name = "chainrpc.ChainNotifier"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
chainrpc.ChainnotifierProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val ConfRequestSerializer = new ScalapbProtobufSerializer(chainrpc.ConfRequest.messageCompanion)
val SpendRequestSerializer = new ScalapbProtobufSerializer(chainrpc.SpendRequest.messageCompanion)
val BlockEpochSerializer = new ScalapbProtobufSerializer(chainrpc.BlockEpoch.messageCompanion)
val ConfEventSerializer = new ScalapbProtobufSerializer(chainrpc.ConfEvent.messageCompanion)
val SpendEventSerializer = new ScalapbProtobufSerializer(chainrpc.SpendEvent.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val registerConfirmationsNtfnDescriptor: MethodDescriptor[chainrpc.ConfRequest, chainrpc.ConfEvent] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainNotifier", "RegisterConfirmationsNtfn"))
.setRequestMarshaller(new Marshaller(ConfRequestSerializer))
.setResponseMarshaller(new Marshaller(ConfEventSerializer))
.setSampledToLocalTracing(true)
.build()
val registerSpendNtfnDescriptor: MethodDescriptor[chainrpc.SpendRequest, chainrpc.SpendEvent] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainNotifier", "RegisterSpendNtfn"))
.setRequestMarshaller(new Marshaller(SpendRequestSerializer))
.setResponseMarshaller(new Marshaller(SpendEventSerializer))
.setSampledToLocalTracing(true)
.build()
val registerBlockEpochNtfnDescriptor: MethodDescriptor[chainrpc.BlockEpoch, chainrpc.BlockEpoch] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("chainrpc.ChainNotifier", "RegisterBlockEpochNtfn"))
.setRequestMarshaller(new Marshaller(BlockEpochSerializer))
.setResponseMarshaller(new Marshaller(BlockEpochSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy