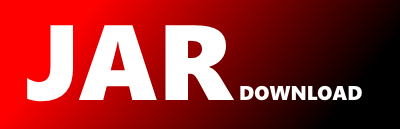
lnrpc.Invoice.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package lnrpc
import org.bitcoins.lnd.rpc.LndUtils._
/** @param memo
*
* An optional memo to attach along with the invoice. Used for record keeping
* purposes for the invoice's creator, and will also be set in the description
* field of the encoded payment request if the description_hash field is not
* being used.
* @param rPreimage
*
* The hex-encoded preimage (32 byte) which will allow settling an incoming
* HTLC payable to this preimage. When using REST, this field must be encoded
* as base64.
* @param rHash
*
* The hash of the preimage. When using REST, this field must be encoded as
* base64.
* Note: Output only, don't specify for creating an invoice.
* @param value
*
* The value of this invoice in satoshis
*
* The fields value and value_msat are mutually exclusive.
* @param valueMsat
*
* The value of this invoice in millisatoshis
*
* The fields value and value_msat are mutually exclusive.
* @param settled
*
* Whether this invoice has been fulfilled.
*
* The field is deprecated. Use the state field instead (compare to SETTLED).
* @param creationDate
*
* When this invoice was created.
* Measured in seconds since the unix epoch.
* Note: Output only, don't specify for creating an invoice.
* @param settleDate
*
* When this invoice was settled.
* Measured in seconds since the unix epoch.
* Note: Output only, don't specify for creating an invoice.
* @param paymentRequest
*
* A bare-bones invoice for a payment within the Lightning Network. With the
* details of the invoice, the sender has all the data necessary to send a
* payment to the recipient.
* Note: Output only, don't specify for creating an invoice.
* @param descriptionHash
*
* Hash (SHA-256) of a description of the payment. Used if the description of
* payment (memo) is too long to naturally fit within the description field
* of an encoded payment request. When using REST, this field must be encoded
* as base64.
* @param expiry
* Payment request expiry time in seconds. Default is 86400 (24 hours).
* @param fallbackAddr
* Fallback on-chain address.
* @param cltvExpiry
* Delta to use for the time-lock of the CLTV extended to the final hop.
* @param routeHints
*
* Route hints that can each be individually used to assist in reaching the
* invoice's destination.
* @param private
* Whether this invoice should include routing hints for private channels.
* Note: When enabled, if value and value_msat are zero, a large number of
* hints with these channels can be included, which might not be desirable.
* @param addIndex
*
* The "add" index of this invoice. Each newly created invoice will increment
* this index making it monotonically increasing. Callers to the
* SubscribeInvoices call can use this to instantly get notified of all added
* invoices with an add_index greater than this one.
* Note: Output only, don't specify for creating an invoice.
* @param settleIndex
*
* The "settle" index of this invoice. Each newly settled invoice will
* increment this index making it monotonically increasing. Callers to the
* SubscribeInvoices call can use this to instantly get notified of all
* settled invoices with an settle_index greater than this one.
* Note: Output only, don't specify for creating an invoice.
* @param amtPaid
* Deprecated, use amt_paid_sat or amt_paid_msat.
* @param amtPaidSat
*
* The amount that was accepted for this invoice, in satoshis. This will ONLY
* be set if this invoice has been settled or accepted. We provide this field
* as if the invoice was created with a zero value, then we need to record what
* amount was ultimately accepted. Additionally, it's possible that the sender
* paid MORE that was specified in the original invoice. So we'll record that
* here as well.
* Note: Output only, don't specify for creating an invoice.
* @param amtPaidMsat
*
* The amount that was accepted for this invoice, in millisatoshis. This will
* ONLY be set if this invoice has been settled or accepted. We provide this
* field as if the invoice was created with a zero value, then we need to
* record what amount was ultimately accepted. Additionally, it's possible that
* the sender paid MORE that was specified in the original invoice. So we'll
* record that here as well.
* Note: Output only, don't specify for creating an invoice.
* @param state
*
* The state the invoice is in.
* Note: Output only, don't specify for creating an invoice.
* @param htlcs
*
* List of HTLCs paying to this invoice [EXPERIMENTAL].
* Note: Output only, don't specify for creating an invoice.
* @param features
*
* List of features advertised on the invoice.
* Note: Output only, don't specify for creating an invoice.
* @param isKeysend
*
* Indicates if this invoice was a spontaneous payment that arrived via keysend
* [EXPERIMENTAL].
* Note: Output only, don't specify for creating an invoice.
* @param paymentAddr
*
* The payment address of this invoice. This value will be used in MPP
* payments, and also for newer invoices that always require the MPP payload
* for added end-to-end security.
* Note: Output only, don't specify for creating an invoice.
* @param isAmp
*
* Signals whether or not this is an AMP invoice.
* @param ampInvoiceState
*
* [EXPERIMENTAL]:
*
* Maps a 32-byte hex-encoded set ID to the sub-invoice AMP state for the
* given set ID. This field is always populated for AMP invoices, and can be
* used along side LookupInvoice to obtain the HTLC information related to a
* given sub-invoice.
* Note: Output only, don't specify for creating an invoice.
*/
@SerialVersionUID(0L)
final case class Invoice(
memo: _root_.scala.Predef.String = "",
rPreimage: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
rHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
value: _root_.scala.Long = 0L,
valueMsat: _root_.scala.Long = 0L,
@scala.deprecated(message="Marked as deprecated in proto file", "") settled: _root_.scala.Boolean = false,
creationDate: _root_.scala.Long = 0L,
settleDate: _root_.scala.Long = 0L,
paymentRequest: _root_.scala.Predef.String = "",
descriptionHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
expiry: _root_.scala.Long = 0L,
fallbackAddr: _root_.scala.Predef.String = "",
cltvExpiry: org.bitcoins.core.number.UInt64 = lnrpc.Invoice._typemapper_cltvExpiry.toCustom(0L),
routeHints: _root_.scala.Seq[lnrpc.RouteHint] = _root_.scala.Seq.empty,
`private`: _root_.scala.Boolean = false,
addIndex: org.bitcoins.core.number.UInt64 = lnrpc.Invoice._typemapper_addIndex.toCustom(0L),
settleIndex: org.bitcoins.core.number.UInt64 = lnrpc.Invoice._typemapper_settleIndex.toCustom(0L),
@scala.deprecated(message="Marked as deprecated in proto file", "") amtPaid: _root_.scala.Long = 0L,
amtPaidSat: _root_.scala.Long = 0L,
amtPaidMsat: _root_.scala.Long = 0L,
state: lnrpc.Invoice.InvoiceState = lnrpc.Invoice.InvoiceState.OPEN,
htlcs: _root_.scala.Seq[lnrpc.InvoiceHTLC] = _root_.scala.Seq.empty,
features: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature] = _root_.scala.collection.immutable.Map.empty,
isKeysend: _root_.scala.Boolean = false,
paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
isAmp: _root_.scala.Boolean = false,
ampInvoiceState: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, lnrpc.AMPInvoiceState] = _root_.scala.collection.immutable.Map.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Invoice] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = memo
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = rPreimage
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
{
val __value = rHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
{
val __value = value
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(5, __value)
}
};
{
val __value = valueMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(23, __value)
}
};
{
val __value = settled
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(6, __value)
}
};
{
val __value = creationDate
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(7, __value)
}
};
{
val __value = settleDate
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(8, __value)
}
};
{
val __value = paymentRequest
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(9, __value)
}
};
{
val __value = descriptionHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(10, __value)
}
};
{
val __value = expiry
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(11, __value)
}
};
{
val __value = fallbackAddr
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(12, __value)
}
};
{
val __value = lnrpc.Invoice._typemapper_cltvExpiry.toBase(cltvExpiry)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(13, __value)
}
};
routeHints.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = `private`
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(15, __value)
}
};
{
val __value = lnrpc.Invoice._typemapper_addIndex.toBase(addIndex)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(16, __value)
}
};
{
val __value = lnrpc.Invoice._typemapper_settleIndex.toBase(settleIndex)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(17, __value)
}
};
{
val __value = amtPaid
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(18, __value)
}
};
{
val __value = amtPaidSat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(19, __value)
}
};
{
val __value = amtPaidMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(20, __value)
}
};
{
val __value = state.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(21, __value)
}
};
htlcs.foreach { __item =>
val __value = __item
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
features.foreach { __item =>
val __value = lnrpc.Invoice._typemapper_features.toBase(__item)
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = isKeysend
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(25, __value)
}
};
{
val __value = paymentAddr
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(26, __value)
}
};
{
val __value = isAmp
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(27, __value)
}
};
ampInvoiceState.foreach { __item =>
val __value = lnrpc.Invoice._typemapper_ampInvoiceState.toBase(__item)
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = memo
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = rPreimage
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
{
val __v = rHash
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
{
val __v = value
if (__v != 0L) {
_output__.writeInt64(5, __v)
}
};
{
val __v = settled
if (__v != false) {
_output__.writeBool(6, __v)
}
};
{
val __v = creationDate
if (__v != 0L) {
_output__.writeInt64(7, __v)
}
};
{
val __v = settleDate
if (__v != 0L) {
_output__.writeInt64(8, __v)
}
};
{
val __v = paymentRequest
if (!__v.isEmpty) {
_output__.writeString(9, __v)
}
};
{
val __v = descriptionHash
if (!__v.isEmpty) {
_output__.writeBytes(10, __v)
}
};
{
val __v = expiry
if (__v != 0L) {
_output__.writeInt64(11, __v)
}
};
{
val __v = fallbackAddr
if (!__v.isEmpty) {
_output__.writeString(12, __v)
}
};
{
val __v = lnrpc.Invoice._typemapper_cltvExpiry.toBase(cltvExpiry)
if (__v != 0L) {
_output__.writeUInt64(13, __v)
}
};
routeHints.foreach { __v =>
val __m = __v
_output__.writeTag(14, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = `private`
if (__v != false) {
_output__.writeBool(15, __v)
}
};
{
val __v = lnrpc.Invoice._typemapper_addIndex.toBase(addIndex)
if (__v != 0L) {
_output__.writeUInt64(16, __v)
}
};
{
val __v = lnrpc.Invoice._typemapper_settleIndex.toBase(settleIndex)
if (__v != 0L) {
_output__.writeUInt64(17, __v)
}
};
{
val __v = amtPaid
if (__v != 0L) {
_output__.writeInt64(18, __v)
}
};
{
val __v = amtPaidSat
if (__v != 0L) {
_output__.writeInt64(19, __v)
}
};
{
val __v = amtPaidMsat
if (__v != 0L) {
_output__.writeInt64(20, __v)
}
};
{
val __v = state.value
if (__v != 0) {
_output__.writeEnum(21, __v)
}
};
htlcs.foreach { __v =>
val __m = __v
_output__.writeTag(22, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = valueMsat
if (__v != 0L) {
_output__.writeInt64(23, __v)
}
};
features.foreach { __v =>
val __m = lnrpc.Invoice._typemapper_features.toBase(__v)
_output__.writeTag(24, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = isKeysend
if (__v != false) {
_output__.writeBool(25, __v)
}
};
{
val __v = paymentAddr
if (!__v.isEmpty) {
_output__.writeBytes(26, __v)
}
};
{
val __v = isAmp
if (__v != false) {
_output__.writeBool(27, __v)
}
};
ampInvoiceState.foreach { __v =>
val __m = lnrpc.Invoice._typemapper_ampInvoiceState.toBase(__v)
_output__.writeTag(28, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withMemo(__v: _root_.scala.Predef.String): Invoice = copy(memo = __v)
def withRPreimage(__v: _root_.com.google.protobuf.ByteString): Invoice = copy(rPreimage = __v)
def withRHash(__v: _root_.com.google.protobuf.ByteString): Invoice = copy(rHash = __v)
def withValue(__v: _root_.scala.Long): Invoice = copy(value = __v)
def withValueMsat(__v: _root_.scala.Long): Invoice = copy(valueMsat = __v)
def withSettled(__v: _root_.scala.Boolean): Invoice = copy(settled = __v)
def withCreationDate(__v: _root_.scala.Long): Invoice = copy(creationDate = __v)
def withSettleDate(__v: _root_.scala.Long): Invoice = copy(settleDate = __v)
def withPaymentRequest(__v: _root_.scala.Predef.String): Invoice = copy(paymentRequest = __v)
def withDescriptionHash(__v: _root_.com.google.protobuf.ByteString): Invoice = copy(descriptionHash = __v)
def withExpiry(__v: _root_.scala.Long): Invoice = copy(expiry = __v)
def withFallbackAddr(__v: _root_.scala.Predef.String): Invoice = copy(fallbackAddr = __v)
def withCltvExpiry(__v: org.bitcoins.core.number.UInt64): Invoice = copy(cltvExpiry = __v)
def clearRouteHints = copy(routeHints = _root_.scala.Seq.empty)
def addRouteHints(__vs: lnrpc.RouteHint *): Invoice = addAllRouteHints(__vs)
def addAllRouteHints(__vs: Iterable[lnrpc.RouteHint]): Invoice = copy(routeHints = routeHints ++ __vs)
def withRouteHints(__v: _root_.scala.Seq[lnrpc.RouteHint]): Invoice = copy(routeHints = __v)
def withPrivate(__v: _root_.scala.Boolean): Invoice = copy(`private` = __v)
def withAddIndex(__v: org.bitcoins.core.number.UInt64): Invoice = copy(addIndex = __v)
def withSettleIndex(__v: org.bitcoins.core.number.UInt64): Invoice = copy(settleIndex = __v)
def withAmtPaid(__v: _root_.scala.Long): Invoice = copy(amtPaid = __v)
def withAmtPaidSat(__v: _root_.scala.Long): Invoice = copy(amtPaidSat = __v)
def withAmtPaidMsat(__v: _root_.scala.Long): Invoice = copy(amtPaidMsat = __v)
def withState(__v: lnrpc.Invoice.InvoiceState): Invoice = copy(state = __v)
def clearHtlcs = copy(htlcs = _root_.scala.Seq.empty)
def addHtlcs(__vs: lnrpc.InvoiceHTLC *): Invoice = addAllHtlcs(__vs)
def addAllHtlcs(__vs: Iterable[lnrpc.InvoiceHTLC]): Invoice = copy(htlcs = htlcs ++ __vs)
def withHtlcs(__v: _root_.scala.Seq[lnrpc.InvoiceHTLC]): Invoice = copy(htlcs = __v)
def clearFeatures = copy(features = _root_.scala.collection.immutable.Map.empty)
def addFeatures(__vs: (org.bitcoins.core.number.UInt32, lnrpc.Feature) *): Invoice = addAllFeatures(__vs)
def addAllFeatures(__vs: Iterable[(org.bitcoins.core.number.UInt32, lnrpc.Feature)]): Invoice = copy(features = features ++ __vs)
def withFeatures(__v: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]): Invoice = copy(features = __v)
def withIsKeysend(__v: _root_.scala.Boolean): Invoice = copy(isKeysend = __v)
def withPaymentAddr(__v: _root_.com.google.protobuf.ByteString): Invoice = copy(paymentAddr = __v)
def withIsAmp(__v: _root_.scala.Boolean): Invoice = copy(isAmp = __v)
def clearAmpInvoiceState = copy(ampInvoiceState = _root_.scala.collection.immutable.Map.empty)
def addAmpInvoiceState(__vs: (_root_.scala.Predef.String, lnrpc.AMPInvoiceState) *): Invoice = addAllAmpInvoiceState(__vs)
def addAllAmpInvoiceState(__vs: Iterable[(_root_.scala.Predef.String, lnrpc.AMPInvoiceState)]): Invoice = copy(ampInvoiceState = ampInvoiceState ++ __vs)
def withAmpInvoiceState(__v: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, lnrpc.AMPInvoiceState]): Invoice = copy(ampInvoiceState = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = memo
if (__t != "") __t else null
}
case 3 => {
val __t = rPreimage
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 4 => {
val __t = rHash
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => {
val __t = value
if (__t != 0L) __t else null
}
case 23 => {
val __t = valueMsat
if (__t != 0L) __t else null
}
case 6 => {
val __t = settled
if (__t != false) __t else null
}
case 7 => {
val __t = creationDate
if (__t != 0L) __t else null
}
case 8 => {
val __t = settleDate
if (__t != 0L) __t else null
}
case 9 => {
val __t = paymentRequest
if (__t != "") __t else null
}
case 10 => {
val __t = descriptionHash
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 11 => {
val __t = expiry
if (__t != 0L) __t else null
}
case 12 => {
val __t = fallbackAddr
if (__t != "") __t else null
}
case 13 => {
val __t = lnrpc.Invoice._typemapper_cltvExpiry.toBase(cltvExpiry)
if (__t != 0L) __t else null
}
case 14 => routeHints
case 15 => {
val __t = `private`
if (__t != false) __t else null
}
case 16 => {
val __t = lnrpc.Invoice._typemapper_addIndex.toBase(addIndex)
if (__t != 0L) __t else null
}
case 17 => {
val __t = lnrpc.Invoice._typemapper_settleIndex.toBase(settleIndex)
if (__t != 0L) __t else null
}
case 18 => {
val __t = amtPaid
if (__t != 0L) __t else null
}
case 19 => {
val __t = amtPaidSat
if (__t != 0L) __t else null
}
case 20 => {
val __t = amtPaidMsat
if (__t != 0L) __t else null
}
case 21 => {
val __t = state.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 22 => htlcs
case 24 => features.iterator.map(lnrpc.Invoice._typemapper_features.toBase(_)).toSeq
case 25 => {
val __t = isKeysend
if (__t != false) __t else null
}
case 26 => {
val __t = paymentAddr
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 27 => {
val __t = isAmp
if (__t != false) __t else null
}
case 28 => ampInvoiceState.iterator.map(lnrpc.Invoice._typemapper_ampInvoiceState.toBase(_)).toSeq
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(memo)
case 3 => _root_.scalapb.descriptors.PByteString(rPreimage)
case 4 => _root_.scalapb.descriptors.PByteString(rHash)
case 5 => _root_.scalapb.descriptors.PLong(value)
case 23 => _root_.scalapb.descriptors.PLong(valueMsat)
case 6 => _root_.scalapb.descriptors.PBoolean(settled)
case 7 => _root_.scalapb.descriptors.PLong(creationDate)
case 8 => _root_.scalapb.descriptors.PLong(settleDate)
case 9 => _root_.scalapb.descriptors.PString(paymentRequest)
case 10 => _root_.scalapb.descriptors.PByteString(descriptionHash)
case 11 => _root_.scalapb.descriptors.PLong(expiry)
case 12 => _root_.scalapb.descriptors.PString(fallbackAddr)
case 13 => _root_.scalapb.descriptors.PLong(lnrpc.Invoice._typemapper_cltvExpiry.toBase(cltvExpiry))
case 14 => _root_.scalapb.descriptors.PRepeated(routeHints.iterator.map(_.toPMessage).toVector)
case 15 => _root_.scalapb.descriptors.PBoolean(`private`)
case 16 => _root_.scalapb.descriptors.PLong(lnrpc.Invoice._typemapper_addIndex.toBase(addIndex))
case 17 => _root_.scalapb.descriptors.PLong(lnrpc.Invoice._typemapper_settleIndex.toBase(settleIndex))
case 18 => _root_.scalapb.descriptors.PLong(amtPaid)
case 19 => _root_.scalapb.descriptors.PLong(amtPaidSat)
case 20 => _root_.scalapb.descriptors.PLong(amtPaidMsat)
case 21 => _root_.scalapb.descriptors.PEnum(state.scalaValueDescriptor)
case 22 => _root_.scalapb.descriptors.PRepeated(htlcs.iterator.map(_.toPMessage).toVector)
case 24 => _root_.scalapb.descriptors.PRepeated(features.iterator.map(lnrpc.Invoice._typemapper_features.toBase(_).toPMessage).toVector)
case 25 => _root_.scalapb.descriptors.PBoolean(isKeysend)
case 26 => _root_.scalapb.descriptors.PByteString(paymentAddr)
case 27 => _root_.scalapb.descriptors.PBoolean(isAmp)
case 28 => _root_.scalapb.descriptors.PRepeated(ampInvoiceState.iterator.map(lnrpc.Invoice._typemapper_ampInvoiceState.toBase(_).toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Invoice.type = lnrpc.Invoice
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Invoice])
}
object Invoice extends scalapb.GeneratedMessageCompanion[lnrpc.Invoice] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Invoice] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Invoice = {
var __memo: _root_.scala.Predef.String = ""
var __rPreimage: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __rHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __value: _root_.scala.Long = 0L
var __valueMsat: _root_.scala.Long = 0L
var __settled: _root_.scala.Boolean = false
var __creationDate: _root_.scala.Long = 0L
var __settleDate: _root_.scala.Long = 0L
var __paymentRequest: _root_.scala.Predef.String = ""
var __descriptionHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __expiry: _root_.scala.Long = 0L
var __fallbackAddr: _root_.scala.Predef.String = ""
var __cltvExpiry: _root_.scala.Long = 0L
val __routeHints: _root_.scala.collection.immutable.VectorBuilder[lnrpc.RouteHint] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.RouteHint]
var __private: _root_.scala.Boolean = false
var __addIndex: _root_.scala.Long = 0L
var __settleIndex: _root_.scala.Long = 0L
var __amtPaid: _root_.scala.Long = 0L
var __amtPaidSat: _root_.scala.Long = 0L
var __amtPaidMsat: _root_.scala.Long = 0L
var __state: lnrpc.Invoice.InvoiceState = lnrpc.Invoice.InvoiceState.OPEN
val __htlcs: _root_.scala.collection.immutable.VectorBuilder[lnrpc.InvoiceHTLC] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.InvoiceHTLC]
val __features: _root_.scala.collection.mutable.Builder[(org.bitcoins.core.number.UInt32, lnrpc.Feature), _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]] = _root_.scala.collection.immutable.Map.newBuilder[org.bitcoins.core.number.UInt32, lnrpc.Feature]
var __isKeysend: _root_.scala.Boolean = false
var __paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __isAmp: _root_.scala.Boolean = false
val __ampInvoiceState: _root_.scala.collection.mutable.Builder[(_root_.scala.Predef.String, lnrpc.AMPInvoiceState), _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, lnrpc.AMPInvoiceState]] = _root_.scala.collection.immutable.Map.newBuilder[_root_.scala.Predef.String, lnrpc.AMPInvoiceState]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__memo = _input__.readStringRequireUtf8()
case 26 =>
__rPreimage = _input__.readBytes()
case 34 =>
__rHash = _input__.readBytes()
case 40 =>
__value = _input__.readInt64()
case 184 =>
__valueMsat = _input__.readInt64()
case 48 =>
__settled = _input__.readBool()
case 56 =>
__creationDate = _input__.readInt64()
case 64 =>
__settleDate = _input__.readInt64()
case 74 =>
__paymentRequest = _input__.readStringRequireUtf8()
case 82 =>
__descriptionHash = _input__.readBytes()
case 88 =>
__expiry = _input__.readInt64()
case 98 =>
__fallbackAddr = _input__.readStringRequireUtf8()
case 104 =>
__cltvExpiry = _input__.readUInt64()
case 114 =>
__routeHints += _root_.scalapb.LiteParser.readMessage[lnrpc.RouteHint](_input__)
case 120 =>
__private = _input__.readBool()
case 128 =>
__addIndex = _input__.readUInt64()
case 136 =>
__settleIndex = _input__.readUInt64()
case 144 =>
__amtPaid = _input__.readInt64()
case 152 =>
__amtPaidSat = _input__.readInt64()
case 160 =>
__amtPaidMsat = _input__.readInt64()
case 168 =>
__state = lnrpc.Invoice.InvoiceState.fromValue(_input__.readEnum())
case 178 =>
__htlcs += _root_.scalapb.LiteParser.readMessage[lnrpc.InvoiceHTLC](_input__)
case 194 =>
__features += lnrpc.Invoice._typemapper_features.toCustom(_root_.scalapb.LiteParser.readMessage[lnrpc.Invoice.FeaturesEntry](_input__))
case 200 =>
__isKeysend = _input__.readBool()
case 210 =>
__paymentAddr = _input__.readBytes()
case 216 =>
__isAmp = _input__.readBool()
case 226 =>
__ampInvoiceState += lnrpc.Invoice._typemapper_ampInvoiceState.toCustom(_root_.scalapb.LiteParser.readMessage[lnrpc.Invoice.AmpInvoiceStateEntry](_input__))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Invoice(
memo = __memo,
rPreimage = __rPreimage,
rHash = __rHash,
value = __value,
valueMsat = __valueMsat,
settled = __settled,
creationDate = __creationDate,
settleDate = __settleDate,
paymentRequest = __paymentRequest,
descriptionHash = __descriptionHash,
expiry = __expiry,
fallbackAddr = __fallbackAddr,
cltvExpiry = lnrpc.Invoice._typemapper_cltvExpiry.toCustom(__cltvExpiry),
routeHints = __routeHints.result(),
`private` = __private,
addIndex = lnrpc.Invoice._typemapper_addIndex.toCustom(__addIndex),
settleIndex = lnrpc.Invoice._typemapper_settleIndex.toCustom(__settleIndex),
amtPaid = __amtPaid,
amtPaidSat = __amtPaidSat,
amtPaidMsat = __amtPaidMsat,
state = __state,
htlcs = __htlcs.result(),
features = __features.result(),
isKeysend = __isKeysend,
paymentAddr = __paymentAddr,
isAmp = __isAmp,
ampInvoiceState = __ampInvoiceState.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Invoice] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Invoice(
memo = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
rPreimage = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
rHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
valueMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(23).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
settled = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
creationDate = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
settleDate = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
paymentRequest = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
descriptionHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
expiry = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
fallbackAddr = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
cltvExpiry = lnrpc.Invoice._typemapper_cltvExpiry.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
routeHints = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scala.Seq[lnrpc.RouteHint]]).getOrElse(_root_.scala.Seq.empty),
`private` = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
addIndex = lnrpc.Invoice._typemapper_addIndex.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(16).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
settleIndex = lnrpc.Invoice._typemapper_settleIndex.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(17).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
amtPaid = __fieldsMap.get(scalaDescriptor.findFieldByNumber(18).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
amtPaidSat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(19).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
amtPaidMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(20).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
state = lnrpc.Invoice.InvoiceState.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(21).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(lnrpc.Invoice.InvoiceState.OPEN.scalaValueDescriptor).number),
htlcs = __fieldsMap.get(scalaDescriptor.findFieldByNumber(22).get).map(_.as[_root_.scala.Seq[lnrpc.InvoiceHTLC]]).getOrElse(_root_.scala.Seq.empty),
features = __fieldsMap.get(scalaDescriptor.findFieldByNumber(24).get).map(_.as[_root_.scala.Seq[lnrpc.Invoice.FeaturesEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(lnrpc.Invoice._typemapper_features.toCustom(_)).toMap,
isKeysend = __fieldsMap.get(scalaDescriptor.findFieldByNumber(25).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
paymentAddr = __fieldsMap.get(scalaDescriptor.findFieldByNumber(26).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
isAmp = __fieldsMap.get(scalaDescriptor.findFieldByNumber(27).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
ampInvoiceState = __fieldsMap.get(scalaDescriptor.findFieldByNumber(28).get).map(_.as[_root_.scala.Seq[lnrpc.Invoice.AmpInvoiceStateEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(lnrpc.Invoice._typemapper_ampInvoiceState.toCustom(_)).toMap
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = LightningProto.javaDescriptor.getMessageTypes().get(128)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = LightningProto.scalaDescriptor.messages(128)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 14 => __out = lnrpc.RouteHint
case 22 => __out = lnrpc.InvoiceHTLC
case 24 => __out = lnrpc.Invoice.FeaturesEntry
case 28 => __out = lnrpc.Invoice.AmpInvoiceStateEntry
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.lnrpc.Invoice.FeaturesEntry,
_root_.lnrpc.Invoice.AmpInvoiceStateEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 21 => lnrpc.Invoice.InvoiceState
}
}
lazy val defaultInstance = lnrpc.Invoice(
memo = "",
rPreimage = _root_.com.google.protobuf.ByteString.EMPTY,
rHash = _root_.com.google.protobuf.ByteString.EMPTY,
value = 0L,
valueMsat = 0L,
settled = false,
creationDate = 0L,
settleDate = 0L,
paymentRequest = "",
descriptionHash = _root_.com.google.protobuf.ByteString.EMPTY,
expiry = 0L,
fallbackAddr = "",
cltvExpiry = lnrpc.Invoice._typemapper_cltvExpiry.toCustom(0L),
routeHints = _root_.scala.Seq.empty,
`private` = false,
addIndex = lnrpc.Invoice._typemapper_addIndex.toCustom(0L),
settleIndex = lnrpc.Invoice._typemapper_settleIndex.toCustom(0L),
amtPaid = 0L,
amtPaidSat = 0L,
amtPaidMsat = 0L,
state = lnrpc.Invoice.InvoiceState.OPEN,
htlcs = _root_.scala.Seq.empty,
features = _root_.scala.collection.immutable.Map.empty,
isKeysend = false,
paymentAddr = _root_.com.google.protobuf.ByteString.EMPTY,
isAmp = false,
ampInvoiceState = _root_.scala.collection.immutable.Map.empty
)
sealed abstract class InvoiceState(val value: _root_.scala.Int) extends _root_.scalapb.GeneratedEnum {
type EnumType = InvoiceState
def isOpen: _root_.scala.Boolean = false
def isSettled: _root_.scala.Boolean = false
def isCanceled: _root_.scala.Boolean = false
def isAccepted: _root_.scala.Boolean = false
def companion: _root_.scalapb.GeneratedEnumCompanion[InvoiceState] = lnrpc.Invoice.InvoiceState
final def asRecognized: _root_.scala.Option[lnrpc.Invoice.InvoiceState.Recognized] = if (isUnrecognized) _root_.scala.None else _root_.scala.Some(this.asInstanceOf[lnrpc.Invoice.InvoiceState.Recognized])
}
object InvoiceState extends _root_.scalapb.GeneratedEnumCompanion[InvoiceState] {
sealed trait Recognized extends InvoiceState
implicit def enumCompanion: _root_.scalapb.GeneratedEnumCompanion[InvoiceState] = this
@SerialVersionUID(0L)
case object OPEN extends InvoiceState(0) with InvoiceState.Recognized {
val index = 0
val name = "OPEN"
override def isOpen: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object SETTLED extends InvoiceState(1) with InvoiceState.Recognized {
val index = 1
val name = "SETTLED"
override def isSettled: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object CANCELED extends InvoiceState(2) with InvoiceState.Recognized {
val index = 2
val name = "CANCELED"
override def isCanceled: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
case object ACCEPTED extends InvoiceState(3) with InvoiceState.Recognized {
val index = 3
val name = "ACCEPTED"
override def isAccepted: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
final case class Unrecognized(unrecognizedValue: _root_.scala.Int) extends InvoiceState(unrecognizedValue) with _root_.scalapb.UnrecognizedEnum
lazy val values = scala.collection.immutable.Seq(OPEN, SETTLED, CANCELED, ACCEPTED)
def fromValue(__value: _root_.scala.Int): InvoiceState = __value match {
case 0 => OPEN
case 1 => SETTLED
case 2 => CANCELED
case 3 => ACCEPTED
case __other => Unrecognized(__other)
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.EnumDescriptor = lnrpc.Invoice.javaDescriptor.getEnumTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.EnumDescriptor = lnrpc.Invoice.scalaDescriptor.enums(0)
}
@SerialVersionUID(0L)
final case class FeaturesEntry(
key: org.bitcoins.core.number.UInt32 = lnrpc.Invoice.FeaturesEntry._typemapper_key.toCustom(0),
value: _root_.scala.Option[lnrpc.Feature] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[FeaturesEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = lnrpc.Invoice.FeaturesEntry._typemapper_key.toBase(key)
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(1, __value)
}
};
if (value.isDefined) {
val __value = value.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = lnrpc.Invoice.FeaturesEntry._typemapper_key.toBase(key)
if (__v != 0) {
_output__.writeUInt32(1, __v)
}
};
value.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withKey(__v: org.bitcoins.core.number.UInt32): FeaturesEntry = copy(key = __v)
def getValue: lnrpc.Feature = value.getOrElse(lnrpc.Feature.defaultInstance)
def clearValue: FeaturesEntry = copy(value = _root_.scala.None)
def withValue(__v: lnrpc.Feature): FeaturesEntry = copy(value = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = lnrpc.Invoice.FeaturesEntry._typemapper_key.toBase(key)
if (__t != 0) __t else null
}
case 2 => value.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PInt(lnrpc.Invoice.FeaturesEntry._typemapper_key.toBase(key))
case 2 => value.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Invoice.FeaturesEntry.type = lnrpc.Invoice.FeaturesEntry
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Invoice.FeaturesEntry])
}
object FeaturesEntry extends scalapb.GeneratedMessageCompanion[lnrpc.Invoice.FeaturesEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Invoice.FeaturesEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Invoice.FeaturesEntry = {
var __key: _root_.scala.Int = 0
var __value: _root_.scala.Option[lnrpc.Feature] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__key = _input__.readUInt32()
case 18 =>
__value = Option(__value.fold(_root_.scalapb.LiteParser.readMessage[lnrpc.Feature](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Invoice.FeaturesEntry(
key = lnrpc.Invoice.FeaturesEntry._typemapper_key.toCustom(__key),
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Invoice.FeaturesEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Invoice.FeaturesEntry(
key = lnrpc.Invoice.FeaturesEntry._typemapper_key.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Int]).getOrElse(0)),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[lnrpc.Feature]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = lnrpc.Invoice.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = lnrpc.Invoice.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = lnrpc.Feature
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.Invoice.FeaturesEntry(
key = lnrpc.Invoice.FeaturesEntry._typemapper_key.toCustom(0),
value = _root_.scala.None
)
implicit class FeaturesEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Invoice.FeaturesEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Invoice.FeaturesEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt32] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Feature] = field(_.getValue)((c_, f_) => c_.copy(value = Option(f_)))
def optionalValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[lnrpc.Feature]] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
private[lnrpc] val _typemapper_key: _root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32]]
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[lnrpc.Invoice.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)] =
_root_.scalapb.TypeMapper[lnrpc.Invoice.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)](__m => (__m.key, __m.getValue))(__p => lnrpc.Invoice.FeaturesEntry(__p._1, Some(__p._2)))
def of(
key: org.bitcoins.core.number.UInt32,
value: _root_.scala.Option[lnrpc.Feature]
): _root_.lnrpc.Invoice.FeaturesEntry = _root_.lnrpc.Invoice.FeaturesEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Invoice.FeaturesEntry])
}
@SerialVersionUID(0L)
final case class AmpInvoiceStateEntry(
key: _root_.scala.Predef.String = "",
value: _root_.scala.Option[lnrpc.AMPInvoiceState] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[AmpInvoiceStateEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = key
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
if (value.isDefined) {
val __value = value.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = key
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
value.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withKey(__v: _root_.scala.Predef.String): AmpInvoiceStateEntry = copy(key = __v)
def getValue: lnrpc.AMPInvoiceState = value.getOrElse(lnrpc.AMPInvoiceState.defaultInstance)
def clearValue: AmpInvoiceStateEntry = copy(value = _root_.scala.None)
def withValue(__v: lnrpc.AMPInvoiceState): AmpInvoiceStateEntry = copy(value = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = key
if (__t != "") __t else null
}
case 2 => value.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(key)
case 2 => value.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Invoice.AmpInvoiceStateEntry.type = lnrpc.Invoice.AmpInvoiceStateEntry
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Invoice.AmpInvoiceStateEntry])
}
object AmpInvoiceStateEntry extends scalapb.GeneratedMessageCompanion[lnrpc.Invoice.AmpInvoiceStateEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Invoice.AmpInvoiceStateEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Invoice.AmpInvoiceStateEntry = {
var __key: _root_.scala.Predef.String = ""
var __value: _root_.scala.Option[lnrpc.AMPInvoiceState] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__key = _input__.readStringRequireUtf8()
case 18 =>
__value = Option(__value.fold(_root_.scalapb.LiteParser.readMessage[lnrpc.AMPInvoiceState](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Invoice.AmpInvoiceStateEntry(
key = __key,
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Invoice.AmpInvoiceStateEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Invoice.AmpInvoiceStateEntry(
key = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[lnrpc.AMPInvoiceState]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = lnrpc.Invoice.javaDescriptor.getNestedTypes().get(1)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = lnrpc.Invoice.scalaDescriptor.nestedMessages(1)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = lnrpc.AMPInvoiceState
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.Invoice.AmpInvoiceStateEntry(
key = "",
value = _root_.scala.None
)
implicit class AmpInvoiceStateEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Invoice.AmpInvoiceStateEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Invoice.AmpInvoiceStateEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.AMPInvoiceState] = field(_.getValue)((c_, f_) => c_.copy(value = Option(f_)))
def optionalValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[lnrpc.AMPInvoiceState]] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[lnrpc.Invoice.AmpInvoiceStateEntry, (_root_.scala.Predef.String, lnrpc.AMPInvoiceState)] =
_root_.scalapb.TypeMapper[lnrpc.Invoice.AmpInvoiceStateEntry, (_root_.scala.Predef.String, lnrpc.AMPInvoiceState)](__m => (__m.key, __m.getValue))(__p => lnrpc.Invoice.AmpInvoiceStateEntry(__p._1, Some(__p._2)))
def of(
key: _root_.scala.Predef.String,
value: _root_.scala.Option[lnrpc.AMPInvoiceState]
): _root_.lnrpc.Invoice.AmpInvoiceStateEntry = _root_.lnrpc.Invoice.AmpInvoiceStateEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Invoice.AmpInvoiceStateEntry])
}
implicit class InvoiceLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Invoice]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Invoice](_l) {
def memo: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.memo)((c_, f_) => c_.copy(memo = f_))
def rPreimage: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.rPreimage)((c_, f_) => c_.copy(rPreimage = f_))
def rHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.rHash)((c_, f_) => c_.copy(rHash = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.value)((c_, f_) => c_.copy(value = f_))
def valueMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.valueMsat)((c_, f_) => c_.copy(valueMsat = f_))
def settled: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.settled)((c_, f_) => c_.copy(settled = f_))
def creationDate: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.creationDate)((c_, f_) => c_.copy(creationDate = f_))
def settleDate: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.settleDate)((c_, f_) => c_.copy(settleDate = f_))
def paymentRequest: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.paymentRequest)((c_, f_) => c_.copy(paymentRequest = f_))
def descriptionHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.descriptionHash)((c_, f_) => c_.copy(descriptionHash = f_))
def expiry: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.expiry)((c_, f_) => c_.copy(expiry = f_))
def fallbackAddr: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.fallbackAddr)((c_, f_) => c_.copy(fallbackAddr = f_))
def cltvExpiry: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.cltvExpiry)((c_, f_) => c_.copy(cltvExpiry = f_))
def routeHints: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.RouteHint]] = field(_.routeHints)((c_, f_) => c_.copy(routeHints = f_))
def `private`: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.`private`)((c_, f_) => c_.copy(`private` = f_))
def addIndex: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.addIndex)((c_, f_) => c_.copy(addIndex = f_))
def settleIndex: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.settleIndex)((c_, f_) => c_.copy(settleIndex = f_))
def amtPaid: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amtPaid)((c_, f_) => c_.copy(amtPaid = f_))
def amtPaidSat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amtPaidSat)((c_, f_) => c_.copy(amtPaidSat = f_))
def amtPaidMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amtPaidMsat)((c_, f_) => c_.copy(amtPaidMsat = f_))
def state: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Invoice.InvoiceState] = field(_.state)((c_, f_) => c_.copy(state = f_))
def htlcs: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.InvoiceHTLC]] = field(_.htlcs)((c_, f_) => c_.copy(htlcs = f_))
def features: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]] = field(_.features)((c_, f_) => c_.copy(features = f_))
def isKeysend: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.isKeysend)((c_, f_) => c_.copy(isKeysend = f_))
def paymentAddr: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.paymentAddr)((c_, f_) => c_.copy(paymentAddr = f_))
def isAmp: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.isAmp)((c_, f_) => c_.copy(isAmp = f_))
def ampInvoiceState: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, lnrpc.AMPInvoiceState]] = field(_.ampInvoiceState)((c_, f_) => c_.copy(ampInvoiceState = f_))
}
final val MEMO_FIELD_NUMBER = 1
final val R_PREIMAGE_FIELD_NUMBER = 3
final val R_HASH_FIELD_NUMBER = 4
final val VALUE_FIELD_NUMBER = 5
final val VALUE_MSAT_FIELD_NUMBER = 23
final val SETTLED_FIELD_NUMBER = 6
final val CREATION_DATE_FIELD_NUMBER = 7
final val SETTLE_DATE_FIELD_NUMBER = 8
final val PAYMENT_REQUEST_FIELD_NUMBER = 9
final val DESCRIPTION_HASH_FIELD_NUMBER = 10
final val EXPIRY_FIELD_NUMBER = 11
final val FALLBACK_ADDR_FIELD_NUMBER = 12
final val CLTV_EXPIRY_FIELD_NUMBER = 13
final val ROUTE_HINTS_FIELD_NUMBER = 14
final val PRIVATE_FIELD_NUMBER = 15
final val ADD_INDEX_FIELD_NUMBER = 16
final val SETTLE_INDEX_FIELD_NUMBER = 17
final val AMT_PAID_FIELD_NUMBER = 18
final val AMT_PAID_SAT_FIELD_NUMBER = 19
final val AMT_PAID_MSAT_FIELD_NUMBER = 20
final val STATE_FIELD_NUMBER = 21
final val HTLCS_FIELD_NUMBER = 22
final val FEATURES_FIELD_NUMBER = 24
final val IS_KEYSEND_FIELD_NUMBER = 25
final val PAYMENT_ADDR_FIELD_NUMBER = 26
final val IS_AMP_FIELD_NUMBER = 27
final val AMP_INVOICE_STATE_FIELD_NUMBER = 28
@transient
private[lnrpc] val _typemapper_cltvExpiry: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_addIndex: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_settleIndex: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_features: _root_.scalapb.TypeMapper[lnrpc.Invoice.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)] = implicitly[_root_.scalapb.TypeMapper[lnrpc.Invoice.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)]]
@transient
private[lnrpc] val _typemapper_ampInvoiceState: _root_.scalapb.TypeMapper[lnrpc.Invoice.AmpInvoiceStateEntry, (_root_.scala.Predef.String, lnrpc.AMPInvoiceState)] = implicitly[_root_.scalapb.TypeMapper[lnrpc.Invoice.AmpInvoiceStateEntry, (_root_.scala.Predef.String, lnrpc.AMPInvoiceState)]]
def of(
memo: _root_.scala.Predef.String,
rPreimage: _root_.com.google.protobuf.ByteString,
rHash: _root_.com.google.protobuf.ByteString,
value: _root_.scala.Long,
valueMsat: _root_.scala.Long,
settled: _root_.scala.Boolean,
creationDate: _root_.scala.Long,
settleDate: _root_.scala.Long,
paymentRequest: _root_.scala.Predef.String,
descriptionHash: _root_.com.google.protobuf.ByteString,
expiry: _root_.scala.Long,
fallbackAddr: _root_.scala.Predef.String,
cltvExpiry: org.bitcoins.core.number.UInt64,
routeHints: _root_.scala.Seq[lnrpc.RouteHint],
`private`: _root_.scala.Boolean,
addIndex: org.bitcoins.core.number.UInt64,
settleIndex: org.bitcoins.core.number.UInt64,
amtPaid: _root_.scala.Long,
amtPaidSat: _root_.scala.Long,
amtPaidMsat: _root_.scala.Long,
state: lnrpc.Invoice.InvoiceState,
htlcs: _root_.scala.Seq[lnrpc.InvoiceHTLC],
features: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature],
isKeysend: _root_.scala.Boolean,
paymentAddr: _root_.com.google.protobuf.ByteString,
isAmp: _root_.scala.Boolean,
ampInvoiceState: _root_.scala.collection.immutable.Map[_root_.scala.Predef.String, lnrpc.AMPInvoiceState]
): _root_.lnrpc.Invoice = _root_.lnrpc.Invoice(
memo,
rPreimage,
rHash,
value,
valueMsat,
settled,
creationDate,
settleDate,
paymentRequest,
descriptionHash,
expiry,
fallbackAddr,
cltvExpiry,
routeHints,
`private`,
addIndex,
settleIndex,
amtPaid,
amtPaidSat,
amtPaidMsat,
state,
htlcs,
features,
isKeysend,
paymentAddr,
isAmp,
ampInvoiceState
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Invoice])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy