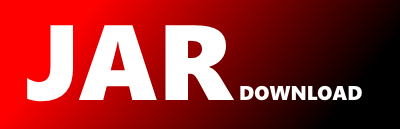
lnrpc.InvoiceHTLC.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package lnrpc
import org.bitcoins.lnd.rpc.LndUtils._
/** Details of an HTLC that paid to an invoice
*
* @param chanId
* Short channel id over which the htlc was received.
* @param htlcIndex
* Index identifying the htlc on the channel.
* @param amtMsat
* The amount of the htlc in msat.
* @param acceptHeight
* Block height at which this htlc was accepted.
* @param acceptTime
* Time at which this htlc was accepted.
* @param resolveTime
* Time at which this htlc was settled or canceled.
* @param expiryHeight
* Block height at which this htlc expires.
* @param state
* Current state the htlc is in.
* @param customRecords
* Custom tlv records.
* @param mppTotalAmtMsat
* The total amount of the mpp payment in msat.
* @param amp
* Details relevant to AMP HTLCs, only populated if this is an AMP HTLC.
*/
@SerialVersionUID(0L)
final case class InvoiceHTLC(
chanId: org.bitcoins.core.number.UInt64 = lnrpc.InvoiceHTLC._typemapper_chanId.toCustom(0L),
htlcIndex: org.bitcoins.core.number.UInt64 = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toCustom(0L),
amtMsat: org.bitcoins.core.number.UInt64 = lnrpc.InvoiceHTLC._typemapper_amtMsat.toCustom(0L),
acceptHeight: _root_.scala.Int = 0,
acceptTime: _root_.scala.Long = 0L,
resolveTime: _root_.scala.Long = 0L,
expiryHeight: _root_.scala.Int = 0,
state: lnrpc.InvoiceHTLCState = lnrpc.InvoiceHTLCState.ACCEPTED,
customRecords: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString] = _root_.scala.collection.immutable.Map.empty,
mppTotalAmtMsat: org.bitcoins.core.number.UInt64 = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toCustom(0L),
amp: _root_.scala.Option[lnrpc.AMP] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[InvoiceHTLC] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = lnrpc.InvoiceHTLC._typemapper_chanId.toBase(chanId)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(1, __value)
}
};
{
val __value = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toBase(htlcIndex)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(2, __value)
}
};
{
val __value = lnrpc.InvoiceHTLC._typemapper_amtMsat.toBase(amtMsat)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(3, __value)
}
};
{
val __value = acceptHeight
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(4, __value)
}
};
{
val __value = acceptTime
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(5, __value)
}
};
{
val __value = resolveTime
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(6, __value)
}
};
{
val __value = expiryHeight
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(7, __value)
}
};
{
val __value = state.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(8, __value)
}
};
customRecords.foreach { __item =>
val __value = lnrpc.InvoiceHTLC._typemapper_customRecords.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toBase(mppTotalAmtMsat)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(10, __value)
}
};
if (amp.isDefined) {
val __value = amp.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = lnrpc.InvoiceHTLC._typemapper_chanId.toBase(chanId)
if (__v != 0L) {
_output__.writeUInt64(1, __v)
}
};
{
val __v = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toBase(htlcIndex)
if (__v != 0L) {
_output__.writeUInt64(2, __v)
}
};
{
val __v = lnrpc.InvoiceHTLC._typemapper_amtMsat.toBase(amtMsat)
if (__v != 0L) {
_output__.writeUInt64(3, __v)
}
};
{
val __v = acceptHeight
if (__v != 0) {
_output__.writeInt32(4, __v)
}
};
{
val __v = acceptTime
if (__v != 0L) {
_output__.writeInt64(5, __v)
}
};
{
val __v = resolveTime
if (__v != 0L) {
_output__.writeInt64(6, __v)
}
};
{
val __v = expiryHeight
if (__v != 0) {
_output__.writeInt32(7, __v)
}
};
{
val __v = state.value
if (__v != 0) {
_output__.writeEnum(8, __v)
}
};
customRecords.foreach { __v =>
val __m = lnrpc.InvoiceHTLC._typemapper_customRecords.toBase(__v)
_output__.writeTag(9, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toBase(mppTotalAmtMsat)
if (__v != 0L) {
_output__.writeUInt64(10, __v)
}
};
amp.foreach { __v =>
val __m = __v
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withChanId(__v: org.bitcoins.core.number.UInt64): InvoiceHTLC = copy(chanId = __v)
def withHtlcIndex(__v: org.bitcoins.core.number.UInt64): InvoiceHTLC = copy(htlcIndex = __v)
def withAmtMsat(__v: org.bitcoins.core.number.UInt64): InvoiceHTLC = copy(amtMsat = __v)
def withAcceptHeight(__v: _root_.scala.Int): InvoiceHTLC = copy(acceptHeight = __v)
def withAcceptTime(__v: _root_.scala.Long): InvoiceHTLC = copy(acceptTime = __v)
def withResolveTime(__v: _root_.scala.Long): InvoiceHTLC = copy(resolveTime = __v)
def withExpiryHeight(__v: _root_.scala.Int): InvoiceHTLC = copy(expiryHeight = __v)
def withState(__v: lnrpc.InvoiceHTLCState): InvoiceHTLC = copy(state = __v)
def clearCustomRecords = copy(customRecords = _root_.scala.collection.immutable.Map.empty)
def addCustomRecords(__vs: (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString) *): InvoiceHTLC = addAllCustomRecords(__vs)
def addAllCustomRecords(__vs: Iterable[(org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)]): InvoiceHTLC = copy(customRecords = customRecords ++ __vs)
def withCustomRecords(__v: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]): InvoiceHTLC = copy(customRecords = __v)
def withMppTotalAmtMsat(__v: org.bitcoins.core.number.UInt64): InvoiceHTLC = copy(mppTotalAmtMsat = __v)
def getAmp: lnrpc.AMP = amp.getOrElse(lnrpc.AMP.defaultInstance)
def clearAmp: InvoiceHTLC = copy(amp = _root_.scala.None)
def withAmp(__v: lnrpc.AMP): InvoiceHTLC = copy(amp = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = lnrpc.InvoiceHTLC._typemapper_chanId.toBase(chanId)
if (__t != 0L) __t else null
}
case 2 => {
val __t = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toBase(htlcIndex)
if (__t != 0L) __t else null
}
case 3 => {
val __t = lnrpc.InvoiceHTLC._typemapper_amtMsat.toBase(amtMsat)
if (__t != 0L) __t else null
}
case 4 => {
val __t = acceptHeight
if (__t != 0) __t else null
}
case 5 => {
val __t = acceptTime
if (__t != 0L) __t else null
}
case 6 => {
val __t = resolveTime
if (__t != 0L) __t else null
}
case 7 => {
val __t = expiryHeight
if (__t != 0) __t else null
}
case 8 => {
val __t = state.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 9 => customRecords.iterator.map(lnrpc.InvoiceHTLC._typemapper_customRecords.toBase(_)).toSeq
case 10 => {
val __t = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toBase(mppTotalAmtMsat)
if (__t != 0L) __t else null
}
case 11 => amp.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PLong(lnrpc.InvoiceHTLC._typemapper_chanId.toBase(chanId))
case 2 => _root_.scalapb.descriptors.PLong(lnrpc.InvoiceHTLC._typemapper_htlcIndex.toBase(htlcIndex))
case 3 => _root_.scalapb.descriptors.PLong(lnrpc.InvoiceHTLC._typemapper_amtMsat.toBase(amtMsat))
case 4 => _root_.scalapb.descriptors.PInt(acceptHeight)
case 5 => _root_.scalapb.descriptors.PLong(acceptTime)
case 6 => _root_.scalapb.descriptors.PLong(resolveTime)
case 7 => _root_.scalapb.descriptors.PInt(expiryHeight)
case 8 => _root_.scalapb.descriptors.PEnum(state.scalaValueDescriptor)
case 9 => _root_.scalapb.descriptors.PRepeated(customRecords.iterator.map(lnrpc.InvoiceHTLC._typemapper_customRecords.toBase(_).toPMessage).toVector)
case 10 => _root_.scalapb.descriptors.PLong(lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toBase(mppTotalAmtMsat))
case 11 => amp.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.InvoiceHTLC.type = lnrpc.InvoiceHTLC
// @@protoc_insertion_point(GeneratedMessage[lnrpc.InvoiceHTLC])
}
object InvoiceHTLC extends scalapb.GeneratedMessageCompanion[lnrpc.InvoiceHTLC] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.InvoiceHTLC] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.InvoiceHTLC = {
var __chanId: _root_.scala.Long = 0L
var __htlcIndex: _root_.scala.Long = 0L
var __amtMsat: _root_.scala.Long = 0L
var __acceptHeight: _root_.scala.Int = 0
var __acceptTime: _root_.scala.Long = 0L
var __resolveTime: _root_.scala.Long = 0L
var __expiryHeight: _root_.scala.Int = 0
var __state: lnrpc.InvoiceHTLCState = lnrpc.InvoiceHTLCState.ACCEPTED
val __customRecords: _root_.scala.collection.mutable.Builder[(org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString), _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]] = _root_.scala.collection.immutable.Map.newBuilder[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]
var __mppTotalAmtMsat: _root_.scala.Long = 0L
var __amp: _root_.scala.Option[lnrpc.AMP] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__chanId = _input__.readUInt64()
case 16 =>
__htlcIndex = _input__.readUInt64()
case 24 =>
__amtMsat = _input__.readUInt64()
case 32 =>
__acceptHeight = _input__.readInt32()
case 40 =>
__acceptTime = _input__.readInt64()
case 48 =>
__resolveTime = _input__.readInt64()
case 56 =>
__expiryHeight = _input__.readInt32()
case 64 =>
__state = lnrpc.InvoiceHTLCState.fromValue(_input__.readEnum())
case 74 =>
__customRecords += lnrpc.InvoiceHTLC._typemapper_customRecords.toCustom(_root_.scalapb.LiteParser.readMessage[lnrpc.InvoiceHTLC.CustomRecordsEntry](_input__))
case 80 =>
__mppTotalAmtMsat = _input__.readUInt64()
case 90 =>
__amp = Option(__amp.fold(_root_.scalapb.LiteParser.readMessage[lnrpc.AMP](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.InvoiceHTLC(
chanId = lnrpc.InvoiceHTLC._typemapper_chanId.toCustom(__chanId),
htlcIndex = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toCustom(__htlcIndex),
amtMsat = lnrpc.InvoiceHTLC._typemapper_amtMsat.toCustom(__amtMsat),
acceptHeight = __acceptHeight,
acceptTime = __acceptTime,
resolveTime = __resolveTime,
expiryHeight = __expiryHeight,
state = __state,
customRecords = __customRecords.result(),
mppTotalAmtMsat = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toCustom(__mppTotalAmtMsat),
amp = __amp,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.InvoiceHTLC] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.InvoiceHTLC(
chanId = lnrpc.InvoiceHTLC._typemapper_chanId.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
htlcIndex = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
amtMsat = lnrpc.InvoiceHTLC._typemapper_amtMsat.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
acceptHeight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Int]).getOrElse(0),
acceptTime = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
resolveTime = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
expiryHeight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Int]).getOrElse(0),
state = lnrpc.InvoiceHTLCState.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(lnrpc.InvoiceHTLCState.ACCEPTED.scalaValueDescriptor).number),
customRecords = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Seq[lnrpc.InvoiceHTLC.CustomRecordsEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(lnrpc.InvoiceHTLC._typemapper_customRecords.toCustom(_)).toMap,
mppTotalAmtMsat = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
amp = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).flatMap(_.as[_root_.scala.Option[lnrpc.AMP]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = LightningProto.javaDescriptor.getMessageTypes().get(129)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = LightningProto.scalaDescriptor.messages(129)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 9 => __out = lnrpc.InvoiceHTLC.CustomRecordsEntry
case 11 => __out = lnrpc.AMP
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.lnrpc.InvoiceHTLC.CustomRecordsEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 8 => lnrpc.InvoiceHTLCState
}
}
lazy val defaultInstance = lnrpc.InvoiceHTLC(
chanId = lnrpc.InvoiceHTLC._typemapper_chanId.toCustom(0L),
htlcIndex = lnrpc.InvoiceHTLC._typemapper_htlcIndex.toCustom(0L),
amtMsat = lnrpc.InvoiceHTLC._typemapper_amtMsat.toCustom(0L),
acceptHeight = 0,
acceptTime = 0L,
resolveTime = 0L,
expiryHeight = 0,
state = lnrpc.InvoiceHTLCState.ACCEPTED,
customRecords = _root_.scala.collection.immutable.Map.empty,
mppTotalAmtMsat = lnrpc.InvoiceHTLC._typemapper_mppTotalAmtMsat.toCustom(0L),
amp = _root_.scala.None
)
@SerialVersionUID(0L)
final case class CustomRecordsEntry(
key: org.bitcoins.core.number.UInt64 = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toCustom(0L),
value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[CustomRecordsEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toBase(key)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(1, __value)
}
};
{
val __value = value
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toBase(key)
if (__v != 0L) {
_output__.writeUInt64(1, __v)
}
};
{
val __v = value
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withKey(__v: org.bitcoins.core.number.UInt64): CustomRecordsEntry = copy(key = __v)
def withValue(__v: _root_.com.google.protobuf.ByteString): CustomRecordsEntry = copy(value = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toBase(key)
if (__t != 0L) __t else null
}
case 2 => {
val __t = value
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PLong(lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toBase(key))
case 2 => _root_.scalapb.descriptors.PByteString(value)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.InvoiceHTLC.CustomRecordsEntry.type = lnrpc.InvoiceHTLC.CustomRecordsEntry
// @@protoc_insertion_point(GeneratedMessage[lnrpc.InvoiceHTLC.CustomRecordsEntry])
}
object CustomRecordsEntry extends scalapb.GeneratedMessageCompanion[lnrpc.InvoiceHTLC.CustomRecordsEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.InvoiceHTLC.CustomRecordsEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.InvoiceHTLC.CustomRecordsEntry = {
var __key: _root_.scala.Long = 0L
var __value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__key = _input__.readUInt64()
case 18 =>
__value = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.InvoiceHTLC.CustomRecordsEntry(
key = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toCustom(__key),
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.InvoiceHTLC.CustomRecordsEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.InvoiceHTLC.CustomRecordsEntry(
key = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = lnrpc.InvoiceHTLC.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = lnrpc.InvoiceHTLC.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.InvoiceHTLC.CustomRecordsEntry(
key = lnrpc.InvoiceHTLC.CustomRecordsEntry._typemapper_key.toCustom(0L),
value = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class CustomRecordsEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.InvoiceHTLC.CustomRecordsEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.InvoiceHTLC.CustomRecordsEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
private[lnrpc] val _typemapper_key: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[lnrpc.InvoiceHTLC.CustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)] =
_root_.scalapb.TypeMapper[lnrpc.InvoiceHTLC.CustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)](__m => (__m.key, __m.value))(__p => lnrpc.InvoiceHTLC.CustomRecordsEntry(__p._1, __p._2))
def of(
key: org.bitcoins.core.number.UInt64,
value: _root_.com.google.protobuf.ByteString
): _root_.lnrpc.InvoiceHTLC.CustomRecordsEntry = _root_.lnrpc.InvoiceHTLC.CustomRecordsEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.InvoiceHTLC.CustomRecordsEntry])
}
implicit class InvoiceHTLCLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.InvoiceHTLC]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.InvoiceHTLC](_l) {
def chanId: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.chanId)((c_, f_) => c_.copy(chanId = f_))
def htlcIndex: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.htlcIndex)((c_, f_) => c_.copy(htlcIndex = f_))
def amtMsat: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.amtMsat)((c_, f_) => c_.copy(amtMsat = f_))
def acceptHeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.acceptHeight)((c_, f_) => c_.copy(acceptHeight = f_))
def acceptTime: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.acceptTime)((c_, f_) => c_.copy(acceptTime = f_))
def resolveTime: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.resolveTime)((c_, f_) => c_.copy(resolveTime = f_))
def expiryHeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.expiryHeight)((c_, f_) => c_.copy(expiryHeight = f_))
def state: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.InvoiceHTLCState] = field(_.state)((c_, f_) => c_.copy(state = f_))
def customRecords: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]] = field(_.customRecords)((c_, f_) => c_.copy(customRecords = f_))
def mppTotalAmtMsat: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.mppTotalAmtMsat)((c_, f_) => c_.copy(mppTotalAmtMsat = f_))
def amp: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.AMP] = field(_.getAmp)((c_, f_) => c_.copy(amp = Option(f_)))
def optionalAmp: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[lnrpc.AMP]] = field(_.amp)((c_, f_) => c_.copy(amp = f_))
}
final val CHAN_ID_FIELD_NUMBER = 1
final val HTLC_INDEX_FIELD_NUMBER = 2
final val AMT_MSAT_FIELD_NUMBER = 3
final val ACCEPT_HEIGHT_FIELD_NUMBER = 4
final val ACCEPT_TIME_FIELD_NUMBER = 5
final val RESOLVE_TIME_FIELD_NUMBER = 6
final val EXPIRY_HEIGHT_FIELD_NUMBER = 7
final val STATE_FIELD_NUMBER = 8
final val CUSTOM_RECORDS_FIELD_NUMBER = 9
final val MPP_TOTAL_AMT_MSAT_FIELD_NUMBER = 10
final val AMP_FIELD_NUMBER = 11
@transient
private[lnrpc] val _typemapper_chanId: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_htlcIndex: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_amtMsat: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_customRecords: _root_.scalapb.TypeMapper[lnrpc.InvoiceHTLC.CustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)] = implicitly[_root_.scalapb.TypeMapper[lnrpc.InvoiceHTLC.CustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)]]
@transient
private[lnrpc] val _typemapper_mppTotalAmtMsat: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
def of(
chanId: org.bitcoins.core.number.UInt64,
htlcIndex: org.bitcoins.core.number.UInt64,
amtMsat: org.bitcoins.core.number.UInt64,
acceptHeight: _root_.scala.Int,
acceptTime: _root_.scala.Long,
resolveTime: _root_.scala.Long,
expiryHeight: _root_.scala.Int,
state: lnrpc.InvoiceHTLCState,
customRecords: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString],
mppTotalAmtMsat: org.bitcoins.core.number.UInt64,
amp: _root_.scala.Option[lnrpc.AMP]
): _root_.lnrpc.InvoiceHTLC = _root_.lnrpc.InvoiceHTLC(
chanId,
htlcIndex,
amtMsat,
acceptHeight,
acceptTime,
resolveTime,
expiryHeight,
state,
customRecords,
mppTotalAmtMsat,
amp
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.InvoiceHTLC])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy