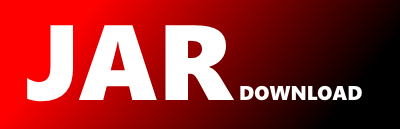
lnrpc.Lightning.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package lnrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* Lightning is the main RPC server of the daemon.
*/
@PekkoGrpcGenerated
trait Lightning {
/**
* lncli: `walletbalance`
* WalletBalance returns total unspent outputs(confirmed and unconfirmed), all
* confirmed unspent outputs and all unconfirmed unspent outputs under control
* of the wallet.
*/
def walletBalance(in: lnrpc.WalletBalanceRequest): scala.concurrent.Future[lnrpc.WalletBalanceResponse]
/**
* lncli: `channelbalance`
* ChannelBalance returns a report on the total funds across all open channels,
* categorized in local/remote, pending local/remote and unsettled local/remote
* balances.
*/
def channelBalance(in: lnrpc.ChannelBalanceRequest): scala.concurrent.Future[lnrpc.ChannelBalanceResponse]
/**
* lncli: `listchaintxns`
* GetTransactions returns a list describing all the known transactions
* relevant to the wallet.
*/
def getTransactions(in: lnrpc.GetTransactionsRequest): scala.concurrent.Future[lnrpc.TransactionDetails]
/**
* lncli: `estimatefee`
* EstimateFee asks the chain backend to estimate the fee rate and total fees
* for a transaction that pays to multiple specified outputs.
* When using REST, the `AddrToAmount` map type can be set by appending
* `&AddrToAmount[<address>]=<amount_to_send>` to the URL. Unfortunately this
* map type doesn't appear in the REST API documentation because of a bug in
* the grpc-gateway library.
*/
def estimateFee(in: lnrpc.EstimateFeeRequest): scala.concurrent.Future[lnrpc.EstimateFeeResponse]
/**
* lncli: `sendcoins`
* SendCoins executes a request to send coins to a particular address. Unlike
* SendMany, this RPC call only allows creating a single output at a time. If
* neither target_conf, or sat_per_vbyte are set, then the internal wallet will
* consult its fee model to determine a fee for the default confirmation
* target.
*/
def sendCoins(in: lnrpc.SendCoinsRequest): scala.concurrent.Future[lnrpc.SendCoinsResponse]
/**
* lncli: `listunspent`
* Deprecated, use walletrpc.ListUnspent instead.
* ListUnspent returns a list of all utxos spendable by the wallet with a
* number of confirmations between the specified minimum and maximum.
*/
def listUnspent(in: lnrpc.ListUnspentRequest): scala.concurrent.Future[lnrpc.ListUnspentResponse]
/**
* SubscribeTransactions creates a uni-directional stream from the server to
* the client in which any newly discovered transactions relevant to the
* wallet are sent over.
*/
def subscribeTransactions(in: lnrpc.GetTransactionsRequest): org.apache.pekko.stream.scaladsl.Source[lnrpc.Transaction, org.apache.pekko.NotUsed]
/**
* lncli: `sendmany`
* SendMany handles a request for a transaction that creates multiple specified
* outputs in parallel. If neither target_conf, or sat_per_vbyte are set, then
* the internal wallet will consult its fee model to determine a fee for the
* default confirmation target.
*/
def sendMany(in: lnrpc.SendManyRequest): scala.concurrent.Future[lnrpc.SendManyResponse]
/**
* lncli: `newaddress`
* NewAddress creates a new address under control of the local wallet.
*/
def newAddress(in: lnrpc.NewAddressRequest): scala.concurrent.Future[lnrpc.NewAddressResponse]
/**
* lncli: `signmessage`
* SignMessage signs a message with this node's private key. The returned
* signature string is `zbase32` encoded and pubkey recoverable, meaning that
* only the message digest and signature are needed for verification.
*/
def signMessage(in: lnrpc.SignMessageRequest): scala.concurrent.Future[lnrpc.SignMessageResponse]
/**
* lncli: `verifymessage`
* VerifyMessage verifies a signature over a message and recovers the signer's
* public key. The signature is only deemed valid if the recovered public key
* corresponds to a node key in the public Lightning network. The signature
* must be zbase32 encoded and signed by an active node in the resident node's
* channel database. In addition to returning the validity of the signature,
* VerifyMessage also returns the recovered pubkey from the signature.
*/
def verifyMessage(in: lnrpc.VerifyMessageRequest): scala.concurrent.Future[lnrpc.VerifyMessageResponse]
/**
* lncli: `connect`
* ConnectPeer attempts to establish a connection to a remote peer. This is at
* the networking level, and is used for communication between nodes. This is
* distinct from establishing a channel with a peer.
*/
def connectPeer(in: lnrpc.ConnectPeerRequest): scala.concurrent.Future[lnrpc.ConnectPeerResponse]
/**
* lncli: `disconnect`
* DisconnectPeer attempts to disconnect one peer from another identified by a
* given pubKey. In the case that we currently have a pending or active channel
* with the target peer, then this action will be not be allowed.
*/
def disconnectPeer(in: lnrpc.DisconnectPeerRequest): scala.concurrent.Future[lnrpc.DisconnectPeerResponse]
/**
* lncli: `listpeers`
* ListPeers returns a verbose listing of all currently active peers.
*/
def listPeers(in: lnrpc.ListPeersRequest): scala.concurrent.Future[lnrpc.ListPeersResponse]
/**
* SubscribePeerEvents creates a uni-directional stream from the server to
* the client in which any events relevant to the state of peers are sent
* over. Events include peers going online and offline.
*/
def subscribePeerEvents(in: lnrpc.PeerEventSubscription): org.apache.pekko.stream.scaladsl.Source[lnrpc.PeerEvent, org.apache.pekko.NotUsed]
/**
* lncli: `getinfo`
* GetInfo returns general information concerning the lightning node including
* it's identity pubkey, alias, the chains it is connected to, and information
* concerning the number of open+pending channels.
*/
def getInfo(in: lnrpc.GetInfoRequest): scala.concurrent.Future[lnrpc.GetInfoResponse]
/**
* * lncli: `getrecoveryinfo`
* GetRecoveryInfo returns information concerning the recovery mode including
* whether it's in a recovery mode, whether the recovery is finished, and the
* progress made so far.
*/
def getRecoveryInfo(in: lnrpc.GetRecoveryInfoRequest): scala.concurrent.Future[lnrpc.GetRecoveryInfoResponse]
/**
* lncli: `pendingchannels`
* PendingChannels returns a list of all the channels that are currently
* considered "pending". A channel is pending if it has finished the funding
* workflow and is waiting for confirmations for the funding txn, or is in the
* process of closure, either initiated cooperatively or non-cooperatively.
*/
def pendingChannels(in: lnrpc.PendingChannelsRequest): scala.concurrent.Future[lnrpc.PendingChannelsResponse]
/**
* lncli: `listchannels`
* ListChannels returns a description of all the open channels that this node
* is a participant in.
*/
def listChannels(in: lnrpc.ListChannelsRequest): scala.concurrent.Future[lnrpc.ListChannelsResponse]
/**
* SubscribeChannelEvents creates a uni-directional stream from the server to
* the client in which any updates relevant to the state of the channels are
* sent over. Events include new active channels, inactive channels, and closed
* channels.
*/
def subscribeChannelEvents(in: lnrpc.ChannelEventSubscription): org.apache.pekko.stream.scaladsl.Source[lnrpc.ChannelEventUpdate, org.apache.pekko.NotUsed]
/**
* lncli: `closedchannels`
* ClosedChannels returns a description of all the closed channels that
* this node was a participant in.
*/
def closedChannels(in: lnrpc.ClosedChannelsRequest): scala.concurrent.Future[lnrpc.ClosedChannelsResponse]
/**
* OpenChannelSync is a synchronous version of the OpenChannel RPC call. This
* call is meant to be consumed by clients to the REST proxy. As with all
* other sync calls, all byte slices are intended to be populated as hex
* encoded strings.
*/
def openChannelSync(in: lnrpc.OpenChannelRequest): scala.concurrent.Future[lnrpc.ChannelPoint]
/**
* lncli: `openchannel`
* OpenChannel attempts to open a singly funded channel specified in the
* request to a remote peer. Users are able to specify a target number of
* blocks that the funding transaction should be confirmed in, or a manual fee
* rate to us for the funding transaction. If neither are specified, then a
* lax block confirmation target is used. Each OpenStatusUpdate will return
* the pending channel ID of the in-progress channel. Depending on the
* arguments specified in the OpenChannelRequest, this pending channel ID can
* then be used to manually progress the channel funding flow.
*/
def openChannel(in: lnrpc.OpenChannelRequest): org.apache.pekko.stream.scaladsl.Source[lnrpc.OpenStatusUpdate, org.apache.pekko.NotUsed]
/**
* lncli: `batchopenchannel`
* BatchOpenChannel attempts to open multiple single-funded channels in a
* single transaction in an atomic way. This means either all channel open
* requests succeed at once or all attempts are aborted if any of them fail.
* This is the safer variant of using PSBTs to manually fund a batch of
* channels through the OpenChannel RPC.
*/
def batchOpenChannel(in: lnrpc.BatchOpenChannelRequest): scala.concurrent.Future[lnrpc.BatchOpenChannelResponse]
/**
* FundingStateStep is an advanced funding related call that allows the caller
* to either execute some preparatory steps for a funding workflow, or
* manually progress a funding workflow. The primary way a funding flow is
* identified is via its pending channel ID. As an example, this method can be
* used to specify that we're expecting a funding flow for a particular
* pending channel ID, for which we need to use specific parameters.
* Alternatively, this can be used to interactively drive PSBT signing for
* funding for partially complete funding transactions.
*/
def fundingStateStep(in: lnrpc.FundingTransitionMsg): scala.concurrent.Future[lnrpc.FundingStateStepResp]
/**
* ChannelAcceptor dispatches a bi-directional streaming RPC in which
* OpenChannel requests are sent to the client and the client responds with
* a boolean that tells LND whether or not to accept the channel. This allows
* node operators to specify their own criteria for accepting inbound channels
* through a single persistent connection.
*/
def channelAcceptor(in: org.apache.pekko.stream.scaladsl.Source[lnrpc.ChannelAcceptResponse, org.apache.pekko.NotUsed]): org.apache.pekko.stream.scaladsl.Source[lnrpc.ChannelAcceptRequest, org.apache.pekko.NotUsed]
/**
* lncli: `closechannel`
* CloseChannel attempts to close an active channel identified by its channel
* outpoint (ChannelPoint). The actions of this method can additionally be
* augmented to attempt a force close after a timeout period in the case of an
* inactive peer. If a non-force close (cooperative closure) is requested,
* then the user can specify either a target number of blocks until the
* closure transaction is confirmed, or a manual fee rate. If neither are
* specified, then a default lax, block confirmation target is used.
*/
def closeChannel(in: lnrpc.CloseChannelRequest): org.apache.pekko.stream.scaladsl.Source[lnrpc.CloseStatusUpdate, org.apache.pekko.NotUsed]
/**
* lncli: `abandonchannel`
* AbandonChannel removes all channel state from the database except for a
* close summary. This method can be used to get rid of permanently unusable
* channels due to bugs fixed in newer versions of lnd. This method can also be
* used to remove externally funded channels where the funding transaction was
* never broadcast. Only available for non-externally funded channels in dev
* build.
*/
def abandonChannel(in: lnrpc.AbandonChannelRequest): scala.concurrent.Future[lnrpc.AbandonChannelResponse]
/**
* lncli: `sendpayment`
* Deprecated, use routerrpc.SendPaymentV2. SendPayment dispatches a
* bi-directional streaming RPC for sending payments through the Lightning
* Network. A single RPC invocation creates a persistent bi-directional
* stream allowing clients to rapidly send payments through the Lightning
* Network with a single persistent connection.
*/
def sendPayment(in: org.apache.pekko.stream.scaladsl.Source[lnrpc.SendRequest, org.apache.pekko.NotUsed]): org.apache.pekko.stream.scaladsl.Source[lnrpc.SendResponse, org.apache.pekko.NotUsed]
/**
* SendPaymentSync is the synchronous non-streaming version of SendPayment.
* This RPC is intended to be consumed by clients of the REST proxy.
* Additionally, this RPC expects the destination's public key and the payment
* hash (if any) to be encoded as hex strings.
*/
def sendPaymentSync(in: lnrpc.SendRequest): scala.concurrent.Future[lnrpc.SendResponse]
/**
* lncli: `sendtoroute`
* Deprecated, use routerrpc.SendToRouteV2. SendToRoute is a bi-directional
* streaming RPC for sending payment through the Lightning Network. This
* method differs from SendPayment in that it allows users to specify a full
* route manually. This can be used for things like rebalancing, and atomic
* swaps.
*/
def sendToRoute(in: org.apache.pekko.stream.scaladsl.Source[lnrpc.SendToRouteRequest, org.apache.pekko.NotUsed]): org.apache.pekko.stream.scaladsl.Source[lnrpc.SendResponse, org.apache.pekko.NotUsed]
/**
* SendToRouteSync is a synchronous version of SendToRoute. It Will block
* until the payment either fails or succeeds.
*/
def sendToRouteSync(in: lnrpc.SendToRouteRequest): scala.concurrent.Future[lnrpc.SendResponse]
/**
* lncli: `addinvoice`
* AddInvoice attempts to add a new invoice to the invoice database. Any
* duplicated invoices are rejected, therefore all invoices *must* have a
* unique payment preimage.
*/
def addInvoice(in: lnrpc.Invoice): scala.concurrent.Future[lnrpc.AddInvoiceResponse]
/**
* lncli: `listinvoices`
* ListInvoices returns a list of all the invoices currently stored within the
* database. Any active debug invoices are ignored. It has full support for
* paginated responses, allowing users to query for specific invoices through
* their add_index. This can be done by using either the first_index_offset or
* last_index_offset fields included in the response as the index_offset of the
* next request. By default, the first 100 invoices created will be returned.
* Backwards pagination is also supported through the Reversed flag.
*/
def listInvoices(in: lnrpc.ListInvoiceRequest): scala.concurrent.Future[lnrpc.ListInvoiceResponse]
/**
* lncli: `lookupinvoice`
* LookupInvoice attempts to look up an invoice according to its payment hash.
* The passed payment hash *must* be exactly 32 bytes, if not, an error is
* returned.
*/
def lookupInvoice(in: lnrpc.PaymentHash): scala.concurrent.Future[lnrpc.Invoice]
/**
* SubscribeInvoices returns a uni-directional stream (server -> client) for
* notifying the client of newly added/settled invoices. The caller can
* optionally specify the add_index and/or the settle_index. If the add_index
* is specified, then we'll first start by sending add invoice events for all
* invoices with an add_index greater than the specified value. If the
* settle_index is specified, the next, we'll send out all settle events for
* invoices with a settle_index greater than the specified value. One or both
* of these fields can be set. If no fields are set, then we'll only send out
* the latest add/settle events.
*/
def subscribeInvoices(in: lnrpc.InvoiceSubscription): org.apache.pekko.stream.scaladsl.Source[lnrpc.Invoice, org.apache.pekko.NotUsed]
/**
* lncli: `decodepayreq`
* DecodePayReq takes an encoded payment request string and attempts to decode
* it, returning a full description of the conditions encoded within the
* payment request.
*/
def decodePayReq(in: lnrpc.PayReqString): scala.concurrent.Future[lnrpc.PayReq]
/**
* lncli: `listpayments`
* ListPayments returns a list of all outgoing payments.
*/
def listPayments(in: lnrpc.ListPaymentsRequest): scala.concurrent.Future[lnrpc.ListPaymentsResponse]
/**
* DeletePayment deletes an outgoing payment from DB. Note that it will not
* attempt to delete an In-Flight payment, since that would be unsafe.
*/
def deletePayment(in: lnrpc.DeletePaymentRequest): scala.concurrent.Future[lnrpc.DeletePaymentResponse]
/**
* DeleteAllPayments deletes all outgoing payments from DB. Note that it will
* not attempt to delete In-Flight payments, since that would be unsafe.
*/
def deleteAllPayments(in: lnrpc.DeleteAllPaymentsRequest): scala.concurrent.Future[lnrpc.DeleteAllPaymentsResponse]
/**
* lncli: `describegraph`
* DescribeGraph returns a description of the latest graph state from the
* point of view of the node. The graph information is partitioned into two
* components: all the nodes/vertexes, and all the edges that connect the
* vertexes themselves. As this is a directed graph, the edges also contain
* the node directional specific routing policy which includes: the time lock
* delta, fee information, etc.
*/
def describeGraph(in: lnrpc.ChannelGraphRequest): scala.concurrent.Future[lnrpc.ChannelGraph]
/**
* lncli: `getnodemetrics`
* GetNodeMetrics returns node metrics calculated from the graph. Currently
* the only supported metric is betweenness centrality of individual nodes.
*/
def getNodeMetrics(in: lnrpc.NodeMetricsRequest): scala.concurrent.Future[lnrpc.NodeMetricsResponse]
/**
* lncli: `getchaninfo`
* GetChanInfo returns the latest authenticated network announcement for the
* given channel identified by its channel ID: an 8-byte integer which
* uniquely identifies the location of transaction's funding output within the
* blockchain.
*/
def getChanInfo(in: lnrpc.ChanInfoRequest): scala.concurrent.Future[lnrpc.ChannelEdge]
/**
* lncli: `getnodeinfo`
* GetNodeInfo returns the latest advertised, aggregated, and authenticated
* channel information for the specified node identified by its public key.
*/
def getNodeInfo(in: lnrpc.NodeInfoRequest): scala.concurrent.Future[lnrpc.NodeInfo]
/**
* lncli: `queryroutes`
* QueryRoutes attempts to query the daemon's Channel Router for a possible
* route to a target destination capable of carrying a specific amount of
* satoshis. The returned route contains the full details required to craft and
* send an HTLC, also including the necessary information that should be
* present within the Sphinx packet encapsulated within the HTLC.
* When using REST, the `dest_custom_records` map type can be set by appending
* `&dest_custom_records[<record_number>]=<record_data_base64_url_encoded>`
* to the URL. Unfortunately this map type doesn't appear in the REST API
* documentation because of a bug in the grpc-gateway library.
*/
def queryRoutes(in: lnrpc.QueryRoutesRequest): scala.concurrent.Future[lnrpc.QueryRoutesResponse]
/**
* lncli: `getnetworkinfo`
* GetNetworkInfo returns some basic stats about the known channel graph from
* the point of view of the node.
*/
def getNetworkInfo(in: lnrpc.NetworkInfoRequest): scala.concurrent.Future[lnrpc.NetworkInfo]
/**
* lncli: `stop`
* StopDaemon will send a shutdown request to the interrupt handler, triggering
* a graceful shutdown of the daemon.
*/
def stopDaemon(in: lnrpc.StopRequest): scala.concurrent.Future[lnrpc.StopResponse]
/**
* SubscribeChannelGraph launches a streaming RPC that allows the caller to
* receive notifications upon any changes to the channel graph topology from
* the point of view of the responding node. Events notified include: new
* nodes coming online, nodes updating their authenticated attributes, new
* channels being advertised, updates in the routing policy for a directional
* channel edge, and when channels are closed on-chain.
*/
def subscribeChannelGraph(in: lnrpc.GraphTopologySubscription): org.apache.pekko.stream.scaladsl.Source[lnrpc.GraphTopologyUpdate, org.apache.pekko.NotUsed]
/**
* lncli: `debuglevel`
* DebugLevel allows a caller to programmatically set the logging verbosity of
* lnd. The logging can be targeted according to a coarse daemon-wide logging
* level, or in a granular fashion to specify the logging for a target
* sub-system.
*/
def debugLevel(in: lnrpc.DebugLevelRequest): scala.concurrent.Future[lnrpc.DebugLevelResponse]
/**
* lncli: `feereport`
* FeeReport allows the caller to obtain a report detailing the current fee
* schedule enforced by the node globally for each channel.
*/
def feeReport(in: lnrpc.FeeReportRequest): scala.concurrent.Future[lnrpc.FeeReportResponse]
/**
* lncli: `updatechanpolicy`
* UpdateChannelPolicy allows the caller to update the fee schedule and
* channel policies for all channels globally, or a particular channel.
*/
def updateChannelPolicy(in: lnrpc.PolicyUpdateRequest): scala.concurrent.Future[lnrpc.PolicyUpdateResponse]
/**
* lncli: `fwdinghistory`
* ForwardingHistory allows the caller to query the htlcswitch for a record of
* all HTLCs forwarded within the target time range, and integer offset
* within that time range, for a maximum number of events. If no maximum number
* of events is specified, up to 100 events will be returned. If no time-range
* is specified, then events will be returned in the order that they occured.
* A list of forwarding events are returned. The size of each forwarding event
* is 40 bytes, and the max message size able to be returned in gRPC is 4 MiB.
* As a result each message can only contain 50k entries. Each response has
* the index offset of the last entry. The index offset can be provided to the
* request to allow the caller to skip a series of records.
*/
def forwardingHistory(in: lnrpc.ForwardingHistoryRequest): scala.concurrent.Future[lnrpc.ForwardingHistoryResponse]
/**
* lncli: `exportchanbackup`
* ExportChannelBackup attempts to return an encrypted static channel backup
* for the target channel identified by it channel point. The backup is
* encrypted with a key generated from the aezeed seed of the user. The
* returned backup can either be restored using the RestoreChannelBackup
* method once lnd is running, or via the InitWallet and UnlockWallet methods
* from the WalletUnlocker service.
*/
def exportChannelBackup(in: lnrpc.ExportChannelBackupRequest): scala.concurrent.Future[lnrpc.ChannelBackup]
/**
* ExportAllChannelBackups returns static channel backups for all existing
* channels known to lnd. A set of regular singular static channel backups for
* each channel are returned. Additionally, a multi-channel backup is returned
* as well, which contains a single encrypted blob containing the backups of
* each channel.
*/
def exportAllChannelBackups(in: lnrpc.ChanBackupExportRequest): scala.concurrent.Future[lnrpc.ChanBackupSnapshot]
/**
* VerifyChanBackup allows a caller to verify the integrity of a channel backup
* snapshot. This method will accept either a packed Single or a packed Multi.
* Specifying both will result in an error.
*/
def verifyChanBackup(in: lnrpc.ChanBackupSnapshot): scala.concurrent.Future[lnrpc.VerifyChanBackupResponse]
/**
* lncli: `restorechanbackup`
* RestoreChannelBackups accepts a set of singular channel backups, or a
* single encrypted multi-chan backup and attempts to recover any funds
* remaining within the channel. If we are able to unpack the backup, then the
* new channel will be shown under listchannels, as well as pending channels.
*/
def restoreChannelBackups(in: lnrpc.RestoreChanBackupRequest): scala.concurrent.Future[lnrpc.RestoreBackupResponse]
/**
* SubscribeChannelBackups allows a client to sub-subscribe to the most up to
* date information concerning the state of all channel backups. Each time a
* new channel is added, we return the new set of channels, along with a
* multi-chan backup containing the backup info for all channels. Each time a
* channel is closed, we send a new update, which contains new new chan back
* ups, but the updated set of encrypted multi-chan backups with the closed
* channel(s) removed.
*/
def subscribeChannelBackups(in: lnrpc.ChannelBackupSubscription): org.apache.pekko.stream.scaladsl.Source[lnrpc.ChanBackupSnapshot, org.apache.pekko.NotUsed]
/**
* lncli: `bakemacaroon`
* BakeMacaroon allows the creation of a new macaroon with custom read and
* write permissions. No first-party caveats are added since this can be done
* offline.
*/
def bakeMacaroon(in: lnrpc.BakeMacaroonRequest): scala.concurrent.Future[lnrpc.BakeMacaroonResponse]
/**
* lncli: `listmacaroonids`
* ListMacaroonIDs returns all root key IDs that are in use.
*/
def listMacaroonIDs(in: lnrpc.ListMacaroonIDsRequest): scala.concurrent.Future[lnrpc.ListMacaroonIDsResponse]
/**
* lncli: `deletemacaroonid`
* DeleteMacaroonID deletes the specified macaroon ID and invalidates all
* macaroons derived from that ID.
*/
def deleteMacaroonID(in: lnrpc.DeleteMacaroonIDRequest): scala.concurrent.Future[lnrpc.DeleteMacaroonIDResponse]
/**
* lncli: `listpermissions`
* ListPermissions lists all RPC method URIs and their required macaroon
* permissions to access them.
*/
def listPermissions(in: lnrpc.ListPermissionsRequest): scala.concurrent.Future[lnrpc.ListPermissionsResponse]
/**
* CheckMacaroonPermissions checks whether a request follows the constraints
* imposed on the macaroon and that the macaroon is authorized to follow the
* provided permissions.
*/
def checkMacaroonPermissions(in: lnrpc.CheckMacPermRequest): scala.concurrent.Future[lnrpc.CheckMacPermResponse]
/**
* RegisterRPCMiddleware adds a new gRPC middleware to the interceptor chain. A
* gRPC middleware is software component external to lnd that aims to add
* additional business logic to lnd by observing/intercepting/validating
* incoming gRPC client requests and (if needed) replacing/overwriting outgoing
* messages before they're sent to the client. When registering the middleware
* must identify itself and indicate what custom macaroon caveats it wants to
* be responsible for. Only requests that contain a macaroon with that specific
* custom caveat are then sent to the middleware for inspection. The other
* option is to register for the read-only mode in which all requests/responses
* are forwarded for interception to the middleware but the middleware is not
* allowed to modify any responses. As a security measure, _no_ middleware can
* modify responses for requests made with _unencumbered_ macaroons!
*/
def registerRPCMiddleware(in: org.apache.pekko.stream.scaladsl.Source[lnrpc.RPCMiddlewareResponse, org.apache.pekko.NotUsed]): org.apache.pekko.stream.scaladsl.Source[lnrpc.RPCMiddlewareRequest, org.apache.pekko.NotUsed]
/**
* lncli: `sendcustom`
* SendCustomMessage sends a custom peer message.
*/
def sendCustomMessage(in: lnrpc.SendCustomMessageRequest): scala.concurrent.Future[lnrpc.SendCustomMessageResponse]
/**
* lncli: `subscribecustom`
* SubscribeCustomMessages subscribes to a stream of incoming custom peer
* messages.
* To include messages with type outside of the custom range (>= 32768) lnd
* needs to be compiled with the `dev` build tag, and the message type to
* override should be specified in lnd's experimental protocol configuration.
*/
def subscribeCustomMessages(in: lnrpc.SubscribeCustomMessagesRequest): org.apache.pekko.stream.scaladsl.Source[lnrpc.CustomMessage, org.apache.pekko.NotUsed]
/**
* lncli: `listaliases`
* ListAliases returns the set of all aliases that have ever existed with
* their confirmed SCID (if it exists) and/or the base SCID (in the case of
* zero conf).
*/
def listAliases(in: lnrpc.ListAliasesRequest): scala.concurrent.Future[lnrpc.ListAliasesResponse]
/**
* LookupHtlcResolution retrieves a final htlc resolution from the database.
* If the htlc has no final resolution yet, a NotFound grpc status code is
* returned.
*/
def lookupHtlcResolution(in: lnrpc.LookupHtlcResolutionRequest): scala.concurrent.Future[lnrpc.LookupHtlcResolutionResponse]
}
@PekkoGrpcGenerated
object Lightning extends pekko.grpc.ServiceDescription {
val name = "lnrpc.Lightning"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
lnrpc.LightningProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val WalletBalanceRequestSerializer = new ScalapbProtobufSerializer(lnrpc.WalletBalanceRequest.messageCompanion)
val ChannelBalanceRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelBalanceRequest.messageCompanion)
val GetTransactionsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.GetTransactionsRequest.messageCompanion)
val EstimateFeeRequestSerializer = new ScalapbProtobufSerializer(lnrpc.EstimateFeeRequest.messageCompanion)
val SendCoinsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SendCoinsRequest.messageCompanion)
val ListUnspentRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListUnspentRequest.messageCompanion)
val SendManyRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SendManyRequest.messageCompanion)
val NewAddressRequestSerializer = new ScalapbProtobufSerializer(lnrpc.NewAddressRequest.messageCompanion)
val SignMessageRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SignMessageRequest.messageCompanion)
val VerifyMessageRequestSerializer = new ScalapbProtobufSerializer(lnrpc.VerifyMessageRequest.messageCompanion)
val ConnectPeerRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ConnectPeerRequest.messageCompanion)
val DisconnectPeerRequestSerializer = new ScalapbProtobufSerializer(lnrpc.DisconnectPeerRequest.messageCompanion)
val ListPeersRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListPeersRequest.messageCompanion)
val PeerEventSubscriptionSerializer = new ScalapbProtobufSerializer(lnrpc.PeerEventSubscription.messageCompanion)
val GetInfoRequestSerializer = new ScalapbProtobufSerializer(lnrpc.GetInfoRequest.messageCompanion)
val GetRecoveryInfoRequestSerializer = new ScalapbProtobufSerializer(lnrpc.GetRecoveryInfoRequest.messageCompanion)
val PendingChannelsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.PendingChannelsRequest.messageCompanion)
val ListChannelsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListChannelsRequest.messageCompanion)
val ChannelEventSubscriptionSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelEventSubscription.messageCompanion)
val ClosedChannelsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ClosedChannelsRequest.messageCompanion)
val OpenChannelRequestSerializer = new ScalapbProtobufSerializer(lnrpc.OpenChannelRequest.messageCompanion)
val BatchOpenChannelRequestSerializer = new ScalapbProtobufSerializer(lnrpc.BatchOpenChannelRequest.messageCompanion)
val FundingTransitionMsgSerializer = new ScalapbProtobufSerializer(lnrpc.FundingTransitionMsg.messageCompanion)
val ChannelAcceptResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelAcceptResponse.messageCompanion)
val CloseChannelRequestSerializer = new ScalapbProtobufSerializer(lnrpc.CloseChannelRequest.messageCompanion)
val AbandonChannelRequestSerializer = new ScalapbProtobufSerializer(lnrpc.AbandonChannelRequest.messageCompanion)
val SendRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SendRequest.messageCompanion)
val SendToRouteRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SendToRouteRequest.messageCompanion)
val InvoiceSerializer = new ScalapbProtobufSerializer(lnrpc.Invoice.messageCompanion)
val ListInvoiceRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListInvoiceRequest.messageCompanion)
val PaymentHashSerializer = new ScalapbProtobufSerializer(lnrpc.PaymentHash.messageCompanion)
val InvoiceSubscriptionSerializer = new ScalapbProtobufSerializer(lnrpc.InvoiceSubscription.messageCompanion)
val PayReqStringSerializer = new ScalapbProtobufSerializer(lnrpc.PayReqString.messageCompanion)
val ListPaymentsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListPaymentsRequest.messageCompanion)
val DeletePaymentRequestSerializer = new ScalapbProtobufSerializer(lnrpc.DeletePaymentRequest.messageCompanion)
val DeleteAllPaymentsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.DeleteAllPaymentsRequest.messageCompanion)
val ChannelGraphRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelGraphRequest.messageCompanion)
val NodeMetricsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.NodeMetricsRequest.messageCompanion)
val ChanInfoRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChanInfoRequest.messageCompanion)
val NodeInfoRequestSerializer = new ScalapbProtobufSerializer(lnrpc.NodeInfoRequest.messageCompanion)
val QueryRoutesRequestSerializer = new ScalapbProtobufSerializer(lnrpc.QueryRoutesRequest.messageCompanion)
val NetworkInfoRequestSerializer = new ScalapbProtobufSerializer(lnrpc.NetworkInfoRequest.messageCompanion)
val StopRequestSerializer = new ScalapbProtobufSerializer(lnrpc.StopRequest.messageCompanion)
val GraphTopologySubscriptionSerializer = new ScalapbProtobufSerializer(lnrpc.GraphTopologySubscription.messageCompanion)
val DebugLevelRequestSerializer = new ScalapbProtobufSerializer(lnrpc.DebugLevelRequest.messageCompanion)
val FeeReportRequestSerializer = new ScalapbProtobufSerializer(lnrpc.FeeReportRequest.messageCompanion)
val PolicyUpdateRequestSerializer = new ScalapbProtobufSerializer(lnrpc.PolicyUpdateRequest.messageCompanion)
val ForwardingHistoryRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ForwardingHistoryRequest.messageCompanion)
val ExportChannelBackupRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ExportChannelBackupRequest.messageCompanion)
val ChanBackupExportRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChanBackupExportRequest.messageCompanion)
val ChanBackupSnapshotSerializer = new ScalapbProtobufSerializer(lnrpc.ChanBackupSnapshot.messageCompanion)
val RestoreChanBackupRequestSerializer = new ScalapbProtobufSerializer(lnrpc.RestoreChanBackupRequest.messageCompanion)
val ChannelBackupSubscriptionSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelBackupSubscription.messageCompanion)
val BakeMacaroonRequestSerializer = new ScalapbProtobufSerializer(lnrpc.BakeMacaroonRequest.messageCompanion)
val ListMacaroonIDsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListMacaroonIDsRequest.messageCompanion)
val DeleteMacaroonIDRequestSerializer = new ScalapbProtobufSerializer(lnrpc.DeleteMacaroonIDRequest.messageCompanion)
val ListPermissionsRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListPermissionsRequest.messageCompanion)
val CheckMacPermRequestSerializer = new ScalapbProtobufSerializer(lnrpc.CheckMacPermRequest.messageCompanion)
val RPCMiddlewareResponseSerializer = new ScalapbProtobufSerializer(lnrpc.RPCMiddlewareResponse.messageCompanion)
val SendCustomMessageRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SendCustomMessageRequest.messageCompanion)
val SubscribeCustomMessagesRequestSerializer = new ScalapbProtobufSerializer(lnrpc.SubscribeCustomMessagesRequest.messageCompanion)
val ListAliasesRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ListAliasesRequest.messageCompanion)
val LookupHtlcResolutionRequestSerializer = new ScalapbProtobufSerializer(lnrpc.LookupHtlcResolutionRequest.messageCompanion)
val WalletBalanceResponseSerializer = new ScalapbProtobufSerializer(lnrpc.WalletBalanceResponse.messageCompanion)
val ChannelBalanceResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelBalanceResponse.messageCompanion)
val TransactionDetailsSerializer = new ScalapbProtobufSerializer(lnrpc.TransactionDetails.messageCompanion)
val EstimateFeeResponseSerializer = new ScalapbProtobufSerializer(lnrpc.EstimateFeeResponse.messageCompanion)
val SendCoinsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.SendCoinsResponse.messageCompanion)
val ListUnspentResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListUnspentResponse.messageCompanion)
val TransactionSerializer = new ScalapbProtobufSerializer(lnrpc.Transaction.messageCompanion)
val SendManyResponseSerializer = new ScalapbProtobufSerializer(lnrpc.SendManyResponse.messageCompanion)
val NewAddressResponseSerializer = new ScalapbProtobufSerializer(lnrpc.NewAddressResponse.messageCompanion)
val SignMessageResponseSerializer = new ScalapbProtobufSerializer(lnrpc.SignMessageResponse.messageCompanion)
val VerifyMessageResponseSerializer = new ScalapbProtobufSerializer(lnrpc.VerifyMessageResponse.messageCompanion)
val ConnectPeerResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ConnectPeerResponse.messageCompanion)
val DisconnectPeerResponseSerializer = new ScalapbProtobufSerializer(lnrpc.DisconnectPeerResponse.messageCompanion)
val ListPeersResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListPeersResponse.messageCompanion)
val PeerEventSerializer = new ScalapbProtobufSerializer(lnrpc.PeerEvent.messageCompanion)
val GetInfoResponseSerializer = new ScalapbProtobufSerializer(lnrpc.GetInfoResponse.messageCompanion)
val GetRecoveryInfoResponseSerializer = new ScalapbProtobufSerializer(lnrpc.GetRecoveryInfoResponse.messageCompanion)
val PendingChannelsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.PendingChannelsResponse.messageCompanion)
val ListChannelsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListChannelsResponse.messageCompanion)
val ChannelEventUpdateSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelEventUpdate.messageCompanion)
val ClosedChannelsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ClosedChannelsResponse.messageCompanion)
val ChannelPointSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelPoint.messageCompanion)
val OpenStatusUpdateSerializer = new ScalapbProtobufSerializer(lnrpc.OpenStatusUpdate.messageCompanion)
val BatchOpenChannelResponseSerializer = new ScalapbProtobufSerializer(lnrpc.BatchOpenChannelResponse.messageCompanion)
val FundingStateStepRespSerializer = new ScalapbProtobufSerializer(lnrpc.FundingStateStepResp.messageCompanion)
val ChannelAcceptRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelAcceptRequest.messageCompanion)
val CloseStatusUpdateSerializer = new ScalapbProtobufSerializer(lnrpc.CloseStatusUpdate.messageCompanion)
val AbandonChannelResponseSerializer = new ScalapbProtobufSerializer(lnrpc.AbandonChannelResponse.messageCompanion)
val SendResponseSerializer = new ScalapbProtobufSerializer(lnrpc.SendResponse.messageCompanion)
val AddInvoiceResponseSerializer = new ScalapbProtobufSerializer(lnrpc.AddInvoiceResponse.messageCompanion)
val ListInvoiceResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListInvoiceResponse.messageCompanion)
val PayReqSerializer = new ScalapbProtobufSerializer(lnrpc.PayReq.messageCompanion)
val ListPaymentsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListPaymentsResponse.messageCompanion)
val DeletePaymentResponseSerializer = new ScalapbProtobufSerializer(lnrpc.DeletePaymentResponse.messageCompanion)
val DeleteAllPaymentsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.DeleteAllPaymentsResponse.messageCompanion)
val ChannelGraphSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelGraph.messageCompanion)
val NodeMetricsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.NodeMetricsResponse.messageCompanion)
val ChannelEdgeSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelEdge.messageCompanion)
val NodeInfoSerializer = new ScalapbProtobufSerializer(lnrpc.NodeInfo.messageCompanion)
val QueryRoutesResponseSerializer = new ScalapbProtobufSerializer(lnrpc.QueryRoutesResponse.messageCompanion)
val NetworkInfoSerializer = new ScalapbProtobufSerializer(lnrpc.NetworkInfo.messageCompanion)
val StopResponseSerializer = new ScalapbProtobufSerializer(lnrpc.StopResponse.messageCompanion)
val GraphTopologyUpdateSerializer = new ScalapbProtobufSerializer(lnrpc.GraphTopologyUpdate.messageCompanion)
val DebugLevelResponseSerializer = new ScalapbProtobufSerializer(lnrpc.DebugLevelResponse.messageCompanion)
val FeeReportResponseSerializer = new ScalapbProtobufSerializer(lnrpc.FeeReportResponse.messageCompanion)
val PolicyUpdateResponseSerializer = new ScalapbProtobufSerializer(lnrpc.PolicyUpdateResponse.messageCompanion)
val ForwardingHistoryResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ForwardingHistoryResponse.messageCompanion)
val ChannelBackupSerializer = new ScalapbProtobufSerializer(lnrpc.ChannelBackup.messageCompanion)
val VerifyChanBackupResponseSerializer = new ScalapbProtobufSerializer(lnrpc.VerifyChanBackupResponse.messageCompanion)
val RestoreBackupResponseSerializer = new ScalapbProtobufSerializer(lnrpc.RestoreBackupResponse.messageCompanion)
val BakeMacaroonResponseSerializer = new ScalapbProtobufSerializer(lnrpc.BakeMacaroonResponse.messageCompanion)
val ListMacaroonIDsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListMacaroonIDsResponse.messageCompanion)
val DeleteMacaroonIDResponseSerializer = new ScalapbProtobufSerializer(lnrpc.DeleteMacaroonIDResponse.messageCompanion)
val ListPermissionsResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListPermissionsResponse.messageCompanion)
val CheckMacPermResponseSerializer = new ScalapbProtobufSerializer(lnrpc.CheckMacPermResponse.messageCompanion)
val RPCMiddlewareRequestSerializer = new ScalapbProtobufSerializer(lnrpc.RPCMiddlewareRequest.messageCompanion)
val SendCustomMessageResponseSerializer = new ScalapbProtobufSerializer(lnrpc.SendCustomMessageResponse.messageCompanion)
val CustomMessageSerializer = new ScalapbProtobufSerializer(lnrpc.CustomMessage.messageCompanion)
val ListAliasesResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ListAliasesResponse.messageCompanion)
val LookupHtlcResolutionResponseSerializer = new ScalapbProtobufSerializer(lnrpc.LookupHtlcResolutionResponse.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val walletBalanceDescriptor: MethodDescriptor[lnrpc.WalletBalanceRequest, lnrpc.WalletBalanceResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "WalletBalance"))
.setRequestMarshaller(new Marshaller(WalletBalanceRequestSerializer))
.setResponseMarshaller(new Marshaller(WalletBalanceResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val channelBalanceDescriptor: MethodDescriptor[lnrpc.ChannelBalanceRequest, lnrpc.ChannelBalanceResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ChannelBalance"))
.setRequestMarshaller(new Marshaller(ChannelBalanceRequestSerializer))
.setResponseMarshaller(new Marshaller(ChannelBalanceResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getTransactionsDescriptor: MethodDescriptor[lnrpc.GetTransactionsRequest, lnrpc.TransactionDetails] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetTransactions"))
.setRequestMarshaller(new Marshaller(GetTransactionsRequestSerializer))
.setResponseMarshaller(new Marshaller(TransactionDetailsSerializer))
.setSampledToLocalTracing(true)
.build()
val estimateFeeDescriptor: MethodDescriptor[lnrpc.EstimateFeeRequest, lnrpc.EstimateFeeResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "EstimateFee"))
.setRequestMarshaller(new Marshaller(EstimateFeeRequestSerializer))
.setResponseMarshaller(new Marshaller(EstimateFeeResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendCoinsDescriptor: MethodDescriptor[lnrpc.SendCoinsRequest, lnrpc.SendCoinsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendCoins"))
.setRequestMarshaller(new Marshaller(SendCoinsRequestSerializer))
.setResponseMarshaller(new Marshaller(SendCoinsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listUnspentDescriptor: MethodDescriptor[lnrpc.ListUnspentRequest, lnrpc.ListUnspentResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListUnspent"))
.setRequestMarshaller(new Marshaller(ListUnspentRequestSerializer))
.setResponseMarshaller(new Marshaller(ListUnspentResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeTransactionsDescriptor: MethodDescriptor[lnrpc.GetTransactionsRequest, lnrpc.Transaction] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeTransactions"))
.setRequestMarshaller(new Marshaller(GetTransactionsRequestSerializer))
.setResponseMarshaller(new Marshaller(TransactionSerializer))
.setSampledToLocalTracing(true)
.build()
val sendManyDescriptor: MethodDescriptor[lnrpc.SendManyRequest, lnrpc.SendManyResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendMany"))
.setRequestMarshaller(new Marshaller(SendManyRequestSerializer))
.setResponseMarshaller(new Marshaller(SendManyResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val newAddressDescriptor: MethodDescriptor[lnrpc.NewAddressRequest, lnrpc.NewAddressResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "NewAddress"))
.setRequestMarshaller(new Marshaller(NewAddressRequestSerializer))
.setResponseMarshaller(new Marshaller(NewAddressResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val signMessageDescriptor: MethodDescriptor[lnrpc.SignMessageRequest, lnrpc.SignMessageResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SignMessage"))
.setRequestMarshaller(new Marshaller(SignMessageRequestSerializer))
.setResponseMarshaller(new Marshaller(SignMessageResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val verifyMessageDescriptor: MethodDescriptor[lnrpc.VerifyMessageRequest, lnrpc.VerifyMessageResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "VerifyMessage"))
.setRequestMarshaller(new Marshaller(VerifyMessageRequestSerializer))
.setResponseMarshaller(new Marshaller(VerifyMessageResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val connectPeerDescriptor: MethodDescriptor[lnrpc.ConnectPeerRequest, lnrpc.ConnectPeerResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ConnectPeer"))
.setRequestMarshaller(new Marshaller(ConnectPeerRequestSerializer))
.setResponseMarshaller(new Marshaller(ConnectPeerResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val disconnectPeerDescriptor: MethodDescriptor[lnrpc.DisconnectPeerRequest, lnrpc.DisconnectPeerResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DisconnectPeer"))
.setRequestMarshaller(new Marshaller(DisconnectPeerRequestSerializer))
.setResponseMarshaller(new Marshaller(DisconnectPeerResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listPeersDescriptor: MethodDescriptor[lnrpc.ListPeersRequest, lnrpc.ListPeersResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListPeers"))
.setRequestMarshaller(new Marshaller(ListPeersRequestSerializer))
.setResponseMarshaller(new Marshaller(ListPeersResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribePeerEventsDescriptor: MethodDescriptor[lnrpc.PeerEventSubscription, lnrpc.PeerEvent] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribePeerEvents"))
.setRequestMarshaller(new Marshaller(PeerEventSubscriptionSerializer))
.setResponseMarshaller(new Marshaller(PeerEventSerializer))
.setSampledToLocalTracing(true)
.build()
val getInfoDescriptor: MethodDescriptor[lnrpc.GetInfoRequest, lnrpc.GetInfoResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetInfo"))
.setRequestMarshaller(new Marshaller(GetInfoRequestSerializer))
.setResponseMarshaller(new Marshaller(GetInfoResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getRecoveryInfoDescriptor: MethodDescriptor[lnrpc.GetRecoveryInfoRequest, lnrpc.GetRecoveryInfoResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetRecoveryInfo"))
.setRequestMarshaller(new Marshaller(GetRecoveryInfoRequestSerializer))
.setResponseMarshaller(new Marshaller(GetRecoveryInfoResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val pendingChannelsDescriptor: MethodDescriptor[lnrpc.PendingChannelsRequest, lnrpc.PendingChannelsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "PendingChannels"))
.setRequestMarshaller(new Marshaller(PendingChannelsRequestSerializer))
.setResponseMarshaller(new Marshaller(PendingChannelsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listChannelsDescriptor: MethodDescriptor[lnrpc.ListChannelsRequest, lnrpc.ListChannelsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListChannels"))
.setRequestMarshaller(new Marshaller(ListChannelsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListChannelsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeChannelEventsDescriptor: MethodDescriptor[lnrpc.ChannelEventSubscription, lnrpc.ChannelEventUpdate] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeChannelEvents"))
.setRequestMarshaller(new Marshaller(ChannelEventSubscriptionSerializer))
.setResponseMarshaller(new Marshaller(ChannelEventUpdateSerializer))
.setSampledToLocalTracing(true)
.build()
val closedChannelsDescriptor: MethodDescriptor[lnrpc.ClosedChannelsRequest, lnrpc.ClosedChannelsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ClosedChannels"))
.setRequestMarshaller(new Marshaller(ClosedChannelsRequestSerializer))
.setResponseMarshaller(new Marshaller(ClosedChannelsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val openChannelSyncDescriptor: MethodDescriptor[lnrpc.OpenChannelRequest, lnrpc.ChannelPoint] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "OpenChannelSync"))
.setRequestMarshaller(new Marshaller(OpenChannelRequestSerializer))
.setResponseMarshaller(new Marshaller(ChannelPointSerializer))
.setSampledToLocalTracing(true)
.build()
val openChannelDescriptor: MethodDescriptor[lnrpc.OpenChannelRequest, lnrpc.OpenStatusUpdate] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "OpenChannel"))
.setRequestMarshaller(new Marshaller(OpenChannelRequestSerializer))
.setResponseMarshaller(new Marshaller(OpenStatusUpdateSerializer))
.setSampledToLocalTracing(true)
.build()
val batchOpenChannelDescriptor: MethodDescriptor[lnrpc.BatchOpenChannelRequest, lnrpc.BatchOpenChannelResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "BatchOpenChannel"))
.setRequestMarshaller(new Marshaller(BatchOpenChannelRequestSerializer))
.setResponseMarshaller(new Marshaller(BatchOpenChannelResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val fundingStateStepDescriptor: MethodDescriptor[lnrpc.FundingTransitionMsg, lnrpc.FundingStateStepResp] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "FundingStateStep"))
.setRequestMarshaller(new Marshaller(FundingTransitionMsgSerializer))
.setResponseMarshaller(new Marshaller(FundingStateStepRespSerializer))
.setSampledToLocalTracing(true)
.build()
val channelAcceptorDescriptor: MethodDescriptor[lnrpc.ChannelAcceptResponse, lnrpc.ChannelAcceptRequest] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.BIDI_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ChannelAcceptor"))
.setRequestMarshaller(new Marshaller(ChannelAcceptResponseSerializer))
.setResponseMarshaller(new Marshaller(ChannelAcceptRequestSerializer))
.setSampledToLocalTracing(true)
.build()
val closeChannelDescriptor: MethodDescriptor[lnrpc.CloseChannelRequest, lnrpc.CloseStatusUpdate] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "CloseChannel"))
.setRequestMarshaller(new Marshaller(CloseChannelRequestSerializer))
.setResponseMarshaller(new Marshaller(CloseStatusUpdateSerializer))
.setSampledToLocalTracing(true)
.build()
val abandonChannelDescriptor: MethodDescriptor[lnrpc.AbandonChannelRequest, lnrpc.AbandonChannelResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "AbandonChannel"))
.setRequestMarshaller(new Marshaller(AbandonChannelRequestSerializer))
.setResponseMarshaller(new Marshaller(AbandonChannelResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendPaymentDescriptor: MethodDescriptor[lnrpc.SendRequest, lnrpc.SendResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.BIDI_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendPayment"))
.setRequestMarshaller(new Marshaller(SendRequestSerializer))
.setResponseMarshaller(new Marshaller(SendResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendPaymentSyncDescriptor: MethodDescriptor[lnrpc.SendRequest, lnrpc.SendResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendPaymentSync"))
.setRequestMarshaller(new Marshaller(SendRequestSerializer))
.setResponseMarshaller(new Marshaller(SendResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendToRouteDescriptor: MethodDescriptor[lnrpc.SendToRouteRequest, lnrpc.SendResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.BIDI_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendToRoute"))
.setRequestMarshaller(new Marshaller(SendToRouteRequestSerializer))
.setResponseMarshaller(new Marshaller(SendResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendToRouteSyncDescriptor: MethodDescriptor[lnrpc.SendToRouteRequest, lnrpc.SendResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendToRouteSync"))
.setRequestMarshaller(new Marshaller(SendToRouteRequestSerializer))
.setResponseMarshaller(new Marshaller(SendResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val addInvoiceDescriptor: MethodDescriptor[lnrpc.Invoice, lnrpc.AddInvoiceResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "AddInvoice"))
.setRequestMarshaller(new Marshaller(InvoiceSerializer))
.setResponseMarshaller(new Marshaller(AddInvoiceResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listInvoicesDescriptor: MethodDescriptor[lnrpc.ListInvoiceRequest, lnrpc.ListInvoiceResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListInvoices"))
.setRequestMarshaller(new Marshaller(ListInvoiceRequestSerializer))
.setResponseMarshaller(new Marshaller(ListInvoiceResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val lookupInvoiceDescriptor: MethodDescriptor[lnrpc.PaymentHash, lnrpc.Invoice] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "LookupInvoice"))
.setRequestMarshaller(new Marshaller(PaymentHashSerializer))
.setResponseMarshaller(new Marshaller(InvoiceSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeInvoicesDescriptor: MethodDescriptor[lnrpc.InvoiceSubscription, lnrpc.Invoice] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeInvoices"))
.setRequestMarshaller(new Marshaller(InvoiceSubscriptionSerializer))
.setResponseMarshaller(new Marshaller(InvoiceSerializer))
.setSampledToLocalTracing(true)
.build()
val decodePayReqDescriptor: MethodDescriptor[lnrpc.PayReqString, lnrpc.PayReq] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DecodePayReq"))
.setRequestMarshaller(new Marshaller(PayReqStringSerializer))
.setResponseMarshaller(new Marshaller(PayReqSerializer))
.setSampledToLocalTracing(true)
.build()
val listPaymentsDescriptor: MethodDescriptor[lnrpc.ListPaymentsRequest, lnrpc.ListPaymentsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListPayments"))
.setRequestMarshaller(new Marshaller(ListPaymentsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListPaymentsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val deletePaymentDescriptor: MethodDescriptor[lnrpc.DeletePaymentRequest, lnrpc.DeletePaymentResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DeletePayment"))
.setRequestMarshaller(new Marshaller(DeletePaymentRequestSerializer))
.setResponseMarshaller(new Marshaller(DeletePaymentResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val deleteAllPaymentsDescriptor: MethodDescriptor[lnrpc.DeleteAllPaymentsRequest, lnrpc.DeleteAllPaymentsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DeleteAllPayments"))
.setRequestMarshaller(new Marshaller(DeleteAllPaymentsRequestSerializer))
.setResponseMarshaller(new Marshaller(DeleteAllPaymentsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val describeGraphDescriptor: MethodDescriptor[lnrpc.ChannelGraphRequest, lnrpc.ChannelGraph] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DescribeGraph"))
.setRequestMarshaller(new Marshaller(ChannelGraphRequestSerializer))
.setResponseMarshaller(new Marshaller(ChannelGraphSerializer))
.setSampledToLocalTracing(true)
.build()
val getNodeMetricsDescriptor: MethodDescriptor[lnrpc.NodeMetricsRequest, lnrpc.NodeMetricsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetNodeMetrics"))
.setRequestMarshaller(new Marshaller(NodeMetricsRequestSerializer))
.setResponseMarshaller(new Marshaller(NodeMetricsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getChanInfoDescriptor: MethodDescriptor[lnrpc.ChanInfoRequest, lnrpc.ChannelEdge] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetChanInfo"))
.setRequestMarshaller(new Marshaller(ChanInfoRequestSerializer))
.setResponseMarshaller(new Marshaller(ChannelEdgeSerializer))
.setSampledToLocalTracing(true)
.build()
val getNodeInfoDescriptor: MethodDescriptor[lnrpc.NodeInfoRequest, lnrpc.NodeInfo] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetNodeInfo"))
.setRequestMarshaller(new Marshaller(NodeInfoRequestSerializer))
.setResponseMarshaller(new Marshaller(NodeInfoSerializer))
.setSampledToLocalTracing(true)
.build()
val queryRoutesDescriptor: MethodDescriptor[lnrpc.QueryRoutesRequest, lnrpc.QueryRoutesResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "QueryRoutes"))
.setRequestMarshaller(new Marshaller(QueryRoutesRequestSerializer))
.setResponseMarshaller(new Marshaller(QueryRoutesResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val getNetworkInfoDescriptor: MethodDescriptor[lnrpc.NetworkInfoRequest, lnrpc.NetworkInfo] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "GetNetworkInfo"))
.setRequestMarshaller(new Marshaller(NetworkInfoRequestSerializer))
.setResponseMarshaller(new Marshaller(NetworkInfoSerializer))
.setSampledToLocalTracing(true)
.build()
val stopDaemonDescriptor: MethodDescriptor[lnrpc.StopRequest, lnrpc.StopResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "StopDaemon"))
.setRequestMarshaller(new Marshaller(StopRequestSerializer))
.setResponseMarshaller(new Marshaller(StopResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeChannelGraphDescriptor: MethodDescriptor[lnrpc.GraphTopologySubscription, lnrpc.GraphTopologyUpdate] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeChannelGraph"))
.setRequestMarshaller(new Marshaller(GraphTopologySubscriptionSerializer))
.setResponseMarshaller(new Marshaller(GraphTopologyUpdateSerializer))
.setSampledToLocalTracing(true)
.build()
val debugLevelDescriptor: MethodDescriptor[lnrpc.DebugLevelRequest, lnrpc.DebugLevelResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DebugLevel"))
.setRequestMarshaller(new Marshaller(DebugLevelRequestSerializer))
.setResponseMarshaller(new Marshaller(DebugLevelResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val feeReportDescriptor: MethodDescriptor[lnrpc.FeeReportRequest, lnrpc.FeeReportResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "FeeReport"))
.setRequestMarshaller(new Marshaller(FeeReportRequestSerializer))
.setResponseMarshaller(new Marshaller(FeeReportResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val updateChannelPolicyDescriptor: MethodDescriptor[lnrpc.PolicyUpdateRequest, lnrpc.PolicyUpdateResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "UpdateChannelPolicy"))
.setRequestMarshaller(new Marshaller(PolicyUpdateRequestSerializer))
.setResponseMarshaller(new Marshaller(PolicyUpdateResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val forwardingHistoryDescriptor: MethodDescriptor[lnrpc.ForwardingHistoryRequest, lnrpc.ForwardingHistoryResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ForwardingHistory"))
.setRequestMarshaller(new Marshaller(ForwardingHistoryRequestSerializer))
.setResponseMarshaller(new Marshaller(ForwardingHistoryResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val exportChannelBackupDescriptor: MethodDescriptor[lnrpc.ExportChannelBackupRequest, lnrpc.ChannelBackup] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ExportChannelBackup"))
.setRequestMarshaller(new Marshaller(ExportChannelBackupRequestSerializer))
.setResponseMarshaller(new Marshaller(ChannelBackupSerializer))
.setSampledToLocalTracing(true)
.build()
val exportAllChannelBackupsDescriptor: MethodDescriptor[lnrpc.ChanBackupExportRequest, lnrpc.ChanBackupSnapshot] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ExportAllChannelBackups"))
.setRequestMarshaller(new Marshaller(ChanBackupExportRequestSerializer))
.setResponseMarshaller(new Marshaller(ChanBackupSnapshotSerializer))
.setSampledToLocalTracing(true)
.build()
val verifyChanBackupDescriptor: MethodDescriptor[lnrpc.ChanBackupSnapshot, lnrpc.VerifyChanBackupResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "VerifyChanBackup"))
.setRequestMarshaller(new Marshaller(ChanBackupSnapshotSerializer))
.setResponseMarshaller(new Marshaller(VerifyChanBackupResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val restoreChannelBackupsDescriptor: MethodDescriptor[lnrpc.RestoreChanBackupRequest, lnrpc.RestoreBackupResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "RestoreChannelBackups"))
.setRequestMarshaller(new Marshaller(RestoreChanBackupRequestSerializer))
.setResponseMarshaller(new Marshaller(RestoreBackupResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeChannelBackupsDescriptor: MethodDescriptor[lnrpc.ChannelBackupSubscription, lnrpc.ChanBackupSnapshot] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeChannelBackups"))
.setRequestMarshaller(new Marshaller(ChannelBackupSubscriptionSerializer))
.setResponseMarshaller(new Marshaller(ChanBackupSnapshotSerializer))
.setSampledToLocalTracing(true)
.build()
val bakeMacaroonDescriptor: MethodDescriptor[lnrpc.BakeMacaroonRequest, lnrpc.BakeMacaroonResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "BakeMacaroon"))
.setRequestMarshaller(new Marshaller(BakeMacaroonRequestSerializer))
.setResponseMarshaller(new Marshaller(BakeMacaroonResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listMacaroonIDsDescriptor: MethodDescriptor[lnrpc.ListMacaroonIDsRequest, lnrpc.ListMacaroonIDsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListMacaroonIDs"))
.setRequestMarshaller(new Marshaller(ListMacaroonIDsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListMacaroonIDsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val deleteMacaroonIDDescriptor: MethodDescriptor[lnrpc.DeleteMacaroonIDRequest, lnrpc.DeleteMacaroonIDResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "DeleteMacaroonID"))
.setRequestMarshaller(new Marshaller(DeleteMacaroonIDRequestSerializer))
.setResponseMarshaller(new Marshaller(DeleteMacaroonIDResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listPermissionsDescriptor: MethodDescriptor[lnrpc.ListPermissionsRequest, lnrpc.ListPermissionsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListPermissions"))
.setRequestMarshaller(new Marshaller(ListPermissionsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListPermissionsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val checkMacaroonPermissionsDescriptor: MethodDescriptor[lnrpc.CheckMacPermRequest, lnrpc.CheckMacPermResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "CheckMacaroonPermissions"))
.setRequestMarshaller(new Marshaller(CheckMacPermRequestSerializer))
.setResponseMarshaller(new Marshaller(CheckMacPermResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val registerRPCMiddlewareDescriptor: MethodDescriptor[lnrpc.RPCMiddlewareResponse, lnrpc.RPCMiddlewareRequest] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.BIDI_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "RegisterRPCMiddleware"))
.setRequestMarshaller(new Marshaller(RPCMiddlewareResponseSerializer))
.setResponseMarshaller(new Marshaller(RPCMiddlewareRequestSerializer))
.setSampledToLocalTracing(true)
.build()
val sendCustomMessageDescriptor: MethodDescriptor[lnrpc.SendCustomMessageRequest, lnrpc.SendCustomMessageResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SendCustomMessage"))
.setRequestMarshaller(new Marshaller(SendCustomMessageRequestSerializer))
.setResponseMarshaller(new Marshaller(SendCustomMessageResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val subscribeCustomMessagesDescriptor: MethodDescriptor[lnrpc.SubscribeCustomMessagesRequest, lnrpc.CustomMessage] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.SERVER_STREAMING
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "SubscribeCustomMessages"))
.setRequestMarshaller(new Marshaller(SubscribeCustomMessagesRequestSerializer))
.setResponseMarshaller(new Marshaller(CustomMessageSerializer))
.setSampledToLocalTracing(true)
.build()
val listAliasesDescriptor: MethodDescriptor[lnrpc.ListAliasesRequest, lnrpc.ListAliasesResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "ListAliases"))
.setRequestMarshaller(new Marshaller(ListAliasesRequestSerializer))
.setResponseMarshaller(new Marshaller(ListAliasesResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val lookupHtlcResolutionDescriptor: MethodDescriptor[lnrpc.LookupHtlcResolutionRequest, lnrpc.LookupHtlcResolutionResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.Lightning", "LookupHtlcResolution"))
.setRequestMarshaller(new Marshaller(LookupHtlcResolutionRequestSerializer))
.setResponseMarshaller(new Marshaller(LookupHtlcResolutionResponseSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy