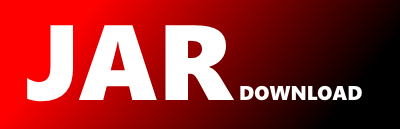
lnrpc.LightningHandler.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package lnrpc
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.grpc.scaladsl.{ GrpcExceptionHandler, GrpcMarshalling }
import pekko.grpc.Trailers
import pekko.actor.ActorSystem
import pekko.actor.ClassicActorSystemProvider
import pekko.annotation.ApiMayChange
import pekko.http.scaladsl.model
import pekko.stream.{Materializer, SystemMaterializer}
import pekko.grpc.internal.TelemetryExtension
import pekko.grpc.PekkoGrpcGenerated
/*
* Generated by Pekko gRPC. DO NOT EDIT.
*
* The API of this class may still change in future Pekko gRPC versions, see for instance
* https://github.com/akka/akka-grpc/issues/994
*/
@ApiMayChange
@PekkoGrpcGenerated
object LightningHandler {
private val notFound = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.NotFound))
private val unsupportedMediaType = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.UnsupportedMediaType))
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `LightningHandler.partial` when combining
* several services.
*/
def apply(implementation: Lightning)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `LightningHandler.partial` when combining
* several services.
*/
def apply(implementation: Lightning, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, Lightning.name, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `LightningHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: Lightning, prefix: String)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `LightningHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: Lightning, prefix: String, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler. The generated handler falls back to a reflection handler for
* `Lightning` and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `LightningHandler.partial` when combining
* several services.
*/
def withServerReflection(implementation: Lightning)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound(
LightningHandler.partial(implementation),
pekko.grpc.scaladsl.ServerReflection.partial(List(Lightning)))
/**
* Creates a partial `HttpRequest` to `HttpResponse` handler that can be combined with handlers of other
* services with `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` and then used in for example
* `Http().bindAndHandleAsync`.
*
* Use `LightningHandler.apply` if the server is only handling one service.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def partial(implementation: Lightning, prefix: String = Lightning.name, eHandler: ActorSystem => PartialFunction[Throwable, Trailers] = GrpcExceptionHandler.defaultMapper)(implicit system: ClassicActorSystemProvider): PartialFunction[model.HttpRequest, scala.concurrent.Future[model.HttpResponse]] = {
implicit val mat: Materializer = SystemMaterializer(system).materializer
implicit val ec: ExecutionContext = mat.executionContext
val spi = TelemetryExtension(system).spi
import Lightning.Serializers._
def handle(request: model.HttpRequest, method: String): scala.concurrent.Future[model.HttpResponse] =
GrpcMarshalling.negotiated(request, (reader, writer) =>
(method match {
case "WalletBalance" =>
GrpcMarshalling.unmarshal(request.entity)(WalletBalanceRequestSerializer, mat, reader)
.flatMap(implementation.walletBalance(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(WalletBalanceResponseSerializer, writer, system))
case "ChannelBalance" =>
GrpcMarshalling.unmarshal(request.entity)(ChannelBalanceRequestSerializer, mat, reader)
.flatMap(implementation.channelBalance(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChannelBalanceResponseSerializer, writer, system))
case "GetTransactions" =>
GrpcMarshalling.unmarshal(request.entity)(GetTransactionsRequestSerializer, mat, reader)
.flatMap(implementation.getTransactions(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(TransactionDetailsSerializer, writer, system))
case "EstimateFee" =>
GrpcMarshalling.unmarshal(request.entity)(EstimateFeeRequestSerializer, mat, reader)
.flatMap(implementation.estimateFee(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(EstimateFeeResponseSerializer, writer, system))
case "SendCoins" =>
GrpcMarshalling.unmarshal(request.entity)(SendCoinsRequestSerializer, mat, reader)
.flatMap(implementation.sendCoins(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendCoinsResponseSerializer, writer, system))
case "ListUnspent" =>
GrpcMarshalling.unmarshal(request.entity)(ListUnspentRequestSerializer, mat, reader)
.flatMap(implementation.listUnspent(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListUnspentResponseSerializer, writer, system))
case "SubscribeTransactions" =>
GrpcMarshalling.unmarshal(request.entity)(GetTransactionsRequestSerializer, mat, reader)
.map(implementation.subscribeTransactions(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(TransactionSerializer, writer, system))
case "SendMany" =>
GrpcMarshalling.unmarshal(request.entity)(SendManyRequestSerializer, mat, reader)
.flatMap(implementation.sendMany(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendManyResponseSerializer, writer, system))
case "NewAddress" =>
GrpcMarshalling.unmarshal(request.entity)(NewAddressRequestSerializer, mat, reader)
.flatMap(implementation.newAddress(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(NewAddressResponseSerializer, writer, system))
case "SignMessage" =>
GrpcMarshalling.unmarshal(request.entity)(SignMessageRequestSerializer, mat, reader)
.flatMap(implementation.signMessage(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SignMessageResponseSerializer, writer, system))
case "VerifyMessage" =>
GrpcMarshalling.unmarshal(request.entity)(VerifyMessageRequestSerializer, mat, reader)
.flatMap(implementation.verifyMessage(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(VerifyMessageResponseSerializer, writer, system))
case "ConnectPeer" =>
GrpcMarshalling.unmarshal(request.entity)(ConnectPeerRequestSerializer, mat, reader)
.flatMap(implementation.connectPeer(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ConnectPeerResponseSerializer, writer, system))
case "DisconnectPeer" =>
GrpcMarshalling.unmarshal(request.entity)(DisconnectPeerRequestSerializer, mat, reader)
.flatMap(implementation.disconnectPeer(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(DisconnectPeerResponseSerializer, writer, system))
case "ListPeers" =>
GrpcMarshalling.unmarshal(request.entity)(ListPeersRequestSerializer, mat, reader)
.flatMap(implementation.listPeers(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListPeersResponseSerializer, writer, system))
case "SubscribePeerEvents" =>
GrpcMarshalling.unmarshal(request.entity)(PeerEventSubscriptionSerializer, mat, reader)
.map(implementation.subscribePeerEvents(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(PeerEventSerializer, writer, system))
case "GetInfo" =>
GrpcMarshalling.unmarshal(request.entity)(GetInfoRequestSerializer, mat, reader)
.flatMap(implementation.getInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(GetInfoResponseSerializer, writer, system))
case "GetRecoveryInfo" =>
GrpcMarshalling.unmarshal(request.entity)(GetRecoveryInfoRequestSerializer, mat, reader)
.flatMap(implementation.getRecoveryInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(GetRecoveryInfoResponseSerializer, writer, system))
case "PendingChannels" =>
GrpcMarshalling.unmarshal(request.entity)(PendingChannelsRequestSerializer, mat, reader)
.flatMap(implementation.pendingChannels(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(PendingChannelsResponseSerializer, writer, system))
case "ListChannels" =>
GrpcMarshalling.unmarshal(request.entity)(ListChannelsRequestSerializer, mat, reader)
.flatMap(implementation.listChannels(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListChannelsResponseSerializer, writer, system))
case "SubscribeChannelEvents" =>
GrpcMarshalling.unmarshal(request.entity)(ChannelEventSubscriptionSerializer, mat, reader)
.map(implementation.subscribeChannelEvents(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(ChannelEventUpdateSerializer, writer, system))
case "ClosedChannels" =>
GrpcMarshalling.unmarshal(request.entity)(ClosedChannelsRequestSerializer, mat, reader)
.flatMap(implementation.closedChannels(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ClosedChannelsResponseSerializer, writer, system))
case "OpenChannelSync" =>
GrpcMarshalling.unmarshal(request.entity)(OpenChannelRequestSerializer, mat, reader)
.flatMap(implementation.openChannelSync(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChannelPointSerializer, writer, system))
case "OpenChannel" =>
GrpcMarshalling.unmarshal(request.entity)(OpenChannelRequestSerializer, mat, reader)
.map(implementation.openChannel(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(OpenStatusUpdateSerializer, writer, system))
case "BatchOpenChannel" =>
GrpcMarshalling.unmarshal(request.entity)(BatchOpenChannelRequestSerializer, mat, reader)
.flatMap(implementation.batchOpenChannel(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(BatchOpenChannelResponseSerializer, writer, system))
case "FundingStateStep" =>
GrpcMarshalling.unmarshal(request.entity)(FundingTransitionMsgSerializer, mat, reader)
.flatMap(implementation.fundingStateStep(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(FundingStateStepRespSerializer, writer, system))
case "ChannelAcceptor" =>
GrpcMarshalling.unmarshalStream(request.entity)(ChannelAcceptResponseSerializer, mat, reader)
.map(implementation.channelAcceptor(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(ChannelAcceptRequestSerializer, writer, system))
case "CloseChannel" =>
GrpcMarshalling.unmarshal(request.entity)(CloseChannelRequestSerializer, mat, reader)
.map(implementation.closeChannel(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(CloseStatusUpdateSerializer, writer, system))
case "AbandonChannel" =>
GrpcMarshalling.unmarshal(request.entity)(AbandonChannelRequestSerializer, mat, reader)
.flatMap(implementation.abandonChannel(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(AbandonChannelResponseSerializer, writer, system))
case "SendPayment" =>
GrpcMarshalling.unmarshalStream(request.entity)(SendRequestSerializer, mat, reader)
.map(implementation.sendPayment(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(SendResponseSerializer, writer, system))
case "SendPaymentSync" =>
GrpcMarshalling.unmarshal(request.entity)(SendRequestSerializer, mat, reader)
.flatMap(implementation.sendPaymentSync(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendResponseSerializer, writer, system))
case "SendToRoute" =>
GrpcMarshalling.unmarshalStream(request.entity)(SendToRouteRequestSerializer, mat, reader)
.map(implementation.sendToRoute(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(SendResponseSerializer, writer, system))
case "SendToRouteSync" =>
GrpcMarshalling.unmarshal(request.entity)(SendToRouteRequestSerializer, mat, reader)
.flatMap(implementation.sendToRouteSync(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendResponseSerializer, writer, system))
case "AddInvoice" =>
GrpcMarshalling.unmarshal(request.entity)(InvoiceSerializer, mat, reader)
.flatMap(implementation.addInvoice(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(AddInvoiceResponseSerializer, writer, system))
case "ListInvoices" =>
GrpcMarshalling.unmarshal(request.entity)(ListInvoiceRequestSerializer, mat, reader)
.flatMap(implementation.listInvoices(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListInvoiceResponseSerializer, writer, system))
case "LookupInvoice" =>
GrpcMarshalling.unmarshal(request.entity)(PaymentHashSerializer, mat, reader)
.flatMap(implementation.lookupInvoice(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(InvoiceSerializer, writer, system))
case "SubscribeInvoices" =>
GrpcMarshalling.unmarshal(request.entity)(InvoiceSubscriptionSerializer, mat, reader)
.map(implementation.subscribeInvoices(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(InvoiceSerializer, writer, system))
case "DecodePayReq" =>
GrpcMarshalling.unmarshal(request.entity)(PayReqStringSerializer, mat, reader)
.flatMap(implementation.decodePayReq(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(PayReqSerializer, writer, system))
case "ListPayments" =>
GrpcMarshalling.unmarshal(request.entity)(ListPaymentsRequestSerializer, mat, reader)
.flatMap(implementation.listPayments(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListPaymentsResponseSerializer, writer, system))
case "DeletePayment" =>
GrpcMarshalling.unmarshal(request.entity)(DeletePaymentRequestSerializer, mat, reader)
.flatMap(implementation.deletePayment(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(DeletePaymentResponseSerializer, writer, system))
case "DeleteAllPayments" =>
GrpcMarshalling.unmarshal(request.entity)(DeleteAllPaymentsRequestSerializer, mat, reader)
.flatMap(implementation.deleteAllPayments(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(DeleteAllPaymentsResponseSerializer, writer, system))
case "DescribeGraph" =>
GrpcMarshalling.unmarshal(request.entity)(ChannelGraphRequestSerializer, mat, reader)
.flatMap(implementation.describeGraph(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChannelGraphSerializer, writer, system))
case "GetNodeMetrics" =>
GrpcMarshalling.unmarshal(request.entity)(NodeMetricsRequestSerializer, mat, reader)
.flatMap(implementation.getNodeMetrics(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(NodeMetricsResponseSerializer, writer, system))
case "GetChanInfo" =>
GrpcMarshalling.unmarshal(request.entity)(ChanInfoRequestSerializer, mat, reader)
.flatMap(implementation.getChanInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChannelEdgeSerializer, writer, system))
case "GetNodeInfo" =>
GrpcMarshalling.unmarshal(request.entity)(NodeInfoRequestSerializer, mat, reader)
.flatMap(implementation.getNodeInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(NodeInfoSerializer, writer, system))
case "QueryRoutes" =>
GrpcMarshalling.unmarshal(request.entity)(QueryRoutesRequestSerializer, mat, reader)
.flatMap(implementation.queryRoutes(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(QueryRoutesResponseSerializer, writer, system))
case "GetNetworkInfo" =>
GrpcMarshalling.unmarshal(request.entity)(NetworkInfoRequestSerializer, mat, reader)
.flatMap(implementation.getNetworkInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(NetworkInfoSerializer, writer, system))
case "StopDaemon" =>
GrpcMarshalling.unmarshal(request.entity)(StopRequestSerializer, mat, reader)
.flatMap(implementation.stopDaemon(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(StopResponseSerializer, writer, system))
case "SubscribeChannelGraph" =>
GrpcMarshalling.unmarshal(request.entity)(GraphTopologySubscriptionSerializer, mat, reader)
.map(implementation.subscribeChannelGraph(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(GraphTopologyUpdateSerializer, writer, system))
case "DebugLevel" =>
GrpcMarshalling.unmarshal(request.entity)(DebugLevelRequestSerializer, mat, reader)
.flatMap(implementation.debugLevel(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(DebugLevelResponseSerializer, writer, system))
case "FeeReport" =>
GrpcMarshalling.unmarshal(request.entity)(FeeReportRequestSerializer, mat, reader)
.flatMap(implementation.feeReport(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(FeeReportResponseSerializer, writer, system))
case "UpdateChannelPolicy" =>
GrpcMarshalling.unmarshal(request.entity)(PolicyUpdateRequestSerializer, mat, reader)
.flatMap(implementation.updateChannelPolicy(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(PolicyUpdateResponseSerializer, writer, system))
case "ForwardingHistory" =>
GrpcMarshalling.unmarshal(request.entity)(ForwardingHistoryRequestSerializer, mat, reader)
.flatMap(implementation.forwardingHistory(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ForwardingHistoryResponseSerializer, writer, system))
case "ExportChannelBackup" =>
GrpcMarshalling.unmarshal(request.entity)(ExportChannelBackupRequestSerializer, mat, reader)
.flatMap(implementation.exportChannelBackup(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChannelBackupSerializer, writer, system))
case "ExportAllChannelBackups" =>
GrpcMarshalling.unmarshal(request.entity)(ChanBackupExportRequestSerializer, mat, reader)
.flatMap(implementation.exportAllChannelBackups(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ChanBackupSnapshotSerializer, writer, system))
case "VerifyChanBackup" =>
GrpcMarshalling.unmarshal(request.entity)(ChanBackupSnapshotSerializer, mat, reader)
.flatMap(implementation.verifyChanBackup(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(VerifyChanBackupResponseSerializer, writer, system))
case "RestoreChannelBackups" =>
GrpcMarshalling.unmarshal(request.entity)(RestoreChanBackupRequestSerializer, mat, reader)
.flatMap(implementation.restoreChannelBackups(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(RestoreBackupResponseSerializer, writer, system))
case "SubscribeChannelBackups" =>
GrpcMarshalling.unmarshal(request.entity)(ChannelBackupSubscriptionSerializer, mat, reader)
.map(implementation.subscribeChannelBackups(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(ChanBackupSnapshotSerializer, writer, system))
case "BakeMacaroon" =>
GrpcMarshalling.unmarshal(request.entity)(BakeMacaroonRequestSerializer, mat, reader)
.flatMap(implementation.bakeMacaroon(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(BakeMacaroonResponseSerializer, writer, system))
case "ListMacaroonIDs" =>
GrpcMarshalling.unmarshal(request.entity)(ListMacaroonIDsRequestSerializer, mat, reader)
.flatMap(implementation.listMacaroonIDs(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListMacaroonIDsResponseSerializer, writer, system))
case "DeleteMacaroonID" =>
GrpcMarshalling.unmarshal(request.entity)(DeleteMacaroonIDRequestSerializer, mat, reader)
.flatMap(implementation.deleteMacaroonID(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(DeleteMacaroonIDResponseSerializer, writer, system))
case "ListPermissions" =>
GrpcMarshalling.unmarshal(request.entity)(ListPermissionsRequestSerializer, mat, reader)
.flatMap(implementation.listPermissions(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListPermissionsResponseSerializer, writer, system))
case "CheckMacaroonPermissions" =>
GrpcMarshalling.unmarshal(request.entity)(CheckMacPermRequestSerializer, mat, reader)
.flatMap(implementation.checkMacaroonPermissions(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(CheckMacPermResponseSerializer, writer, system))
case "RegisterRPCMiddleware" =>
GrpcMarshalling.unmarshalStream(request.entity)(RPCMiddlewareResponseSerializer, mat, reader)
.map(implementation.registerRPCMiddleware(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(RPCMiddlewareRequestSerializer, writer, system))
case "SendCustomMessage" =>
GrpcMarshalling.unmarshal(request.entity)(SendCustomMessageRequestSerializer, mat, reader)
.flatMap(implementation.sendCustomMessage(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendCustomMessageResponseSerializer, writer, system))
case "SubscribeCustomMessages" =>
GrpcMarshalling.unmarshal(request.entity)(SubscribeCustomMessagesRequestSerializer, mat, reader)
.map(implementation.subscribeCustomMessages(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(CustomMessageSerializer, writer, system))
case "ListAliases" =>
GrpcMarshalling.unmarshal(request.entity)(ListAliasesRequestSerializer, mat, reader)
.flatMap(implementation.listAliases(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListAliasesResponseSerializer, writer, system))
case "LookupHtlcResolution" =>
GrpcMarshalling.unmarshal(request.entity)(LookupHtlcResolutionRequestSerializer, mat, reader)
.flatMap(implementation.lookupHtlcResolution(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(LookupHtlcResolutionResponseSerializer, writer, system))
case m => scala.concurrent.Future.failed(new NotImplementedError(s"Not implemented: $m"))
})
.recoverWith(GrpcExceptionHandler.from(eHandler(system.classicSystem))(system, writer))
).getOrElse(unsupportedMediaType)
Function.unlift((req: model.HttpRequest) => req.uri.path match {
case model.Uri.Path.Slash(model.Uri.Path.Segment(`prefix`, model.Uri.Path.Slash(model.Uri.Path.Segment(method, model.Uri.Path.Empty)))) =>
Some(handle(spi.onRequest(prefix, method, req), method))
case _ =>
None
})
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy